TypeScript filter out nulls from an array
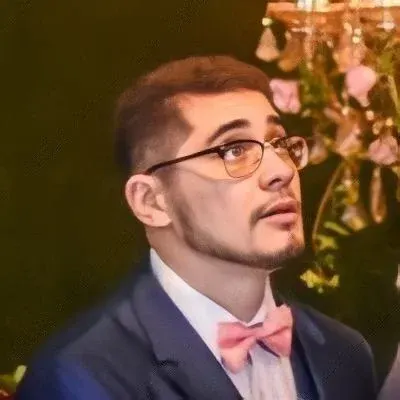

A Quick Guide: Filtering Nulls from an Array in TypeScript
π Hey there tech enthusiasts! Welcome to another exciting blog post on our tech blog. Today, we're going to tackle a common problem in TypeScript - how to filter out nulls from an array. π
The Setup: TypeScript with --strictNullChecks
Mode πͺ
First things first, let's set the context. We're working with TypeScript in --strictNullChecks
mode. This means that our array contains nullable strings of type (string | null)[]
. Our goal is to remove all the nulls and transform the array into type string[]
. Easy peasy, right? Let's dig in. π€
The Challenge: Array.filter Won't Do the Trick π±
At first glance, you might think that using the Array.filter
method is the way to go. However, if you try it out, you'll quickly realize it won't work in this case. π«
const array: (string | null)[] = ["foo", "bar", null, "zoo", null];
const filteredArray: string[] = array.filter(x => x != null);
Unfortunately, TypeScript throws an error: Type '(string | null)[]' is not assignable to type 'string[]'
. Oops! We need a different approach. π
The Solution: A Single-Expression Magic β¨
Thankfully, we have a simple, single-expression solution for you. Meet the Array.reduce
method. π
const array: (string | null)[] = ["foo", "bar", null, "zoo", null];
const filteredArray: string[] = array.reduce((acc, curr) => {
if (curr != null) {
acc.push(curr);
}
return acc;
}, []);
Woo-hoo! π By using Array.reduce
, we can traverse our array and build a new array that only contains the non-null values. This way, TypeScript is happy, and we have our desired string[]
type for filteredArray
.
Handling Common Use Cases: Filtering Any Union Type π
Now, let's say we have a more general problem - filtering an array of any union type by removing entries having a particular type from the union. Fear not, we've got you covered. π
For the most common use cases involving unions with null and maybe undefined values, we can utilize the NonNullish
type provided by TypeScript. Here's an example:
type NonNullish<T> = T extends null | undefined ? never : T;
const array: (string | null)[] = ["foo", "bar", null, "zoo", null];
const filteredArray: NonNullish<typeof array[number]>[] = array.reduce((acc, curr) => {
if (curr != null) {
acc.push(curr);
}
return acc;
}, []);
By using the NonNullish
type, we can filter out any specific type from a union, even if it's not limited to null and undefined values.
Your Turn: Engage with Us! π¬
β¨And that's a wrap, folks!β¨ We've tackled the challenge of filtering nulls from an array in TypeScript and even expanded the solution to handle common use cases involving union types.
Now, it's your time to shine. Have you ever encountered similar challenges while working with TypeScript arrays? Let us know in the comments below! We'd love to hear your experiences and any other TypeScript tips and tricks you have up your sleeve. π
Stay tuned for more exciting tech content and be sure to subscribe to our blog for regular updates. Happy coding! πβ¨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
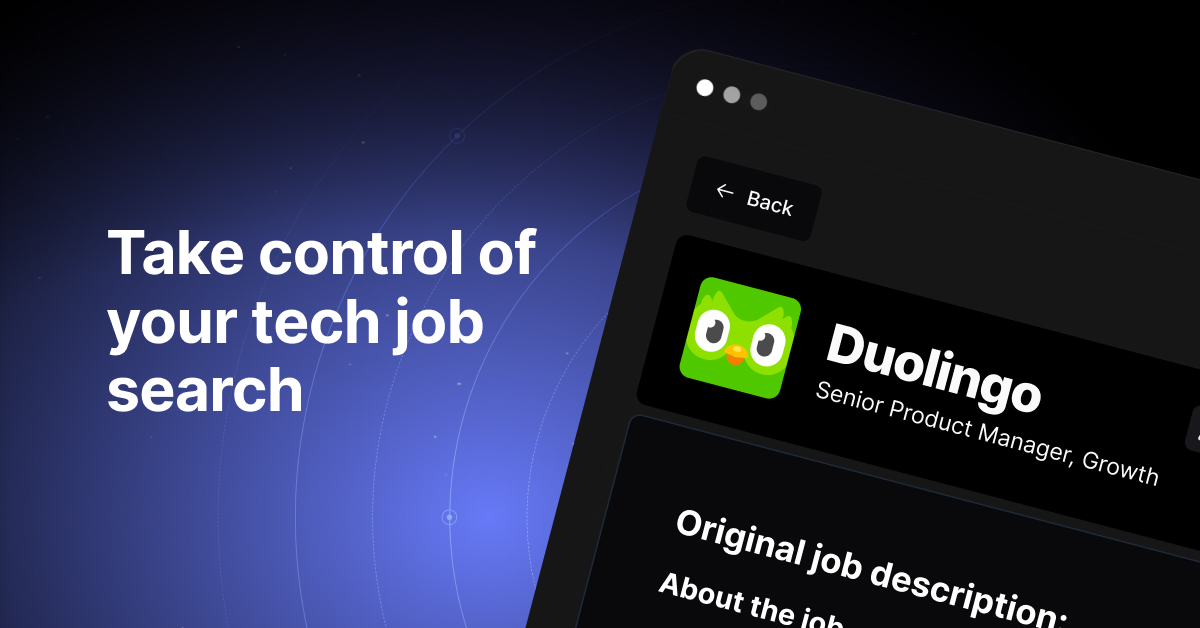