TypeScript and field initializers
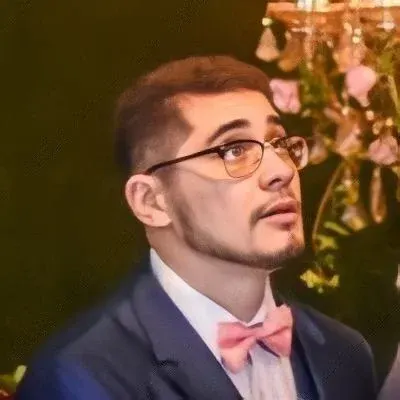
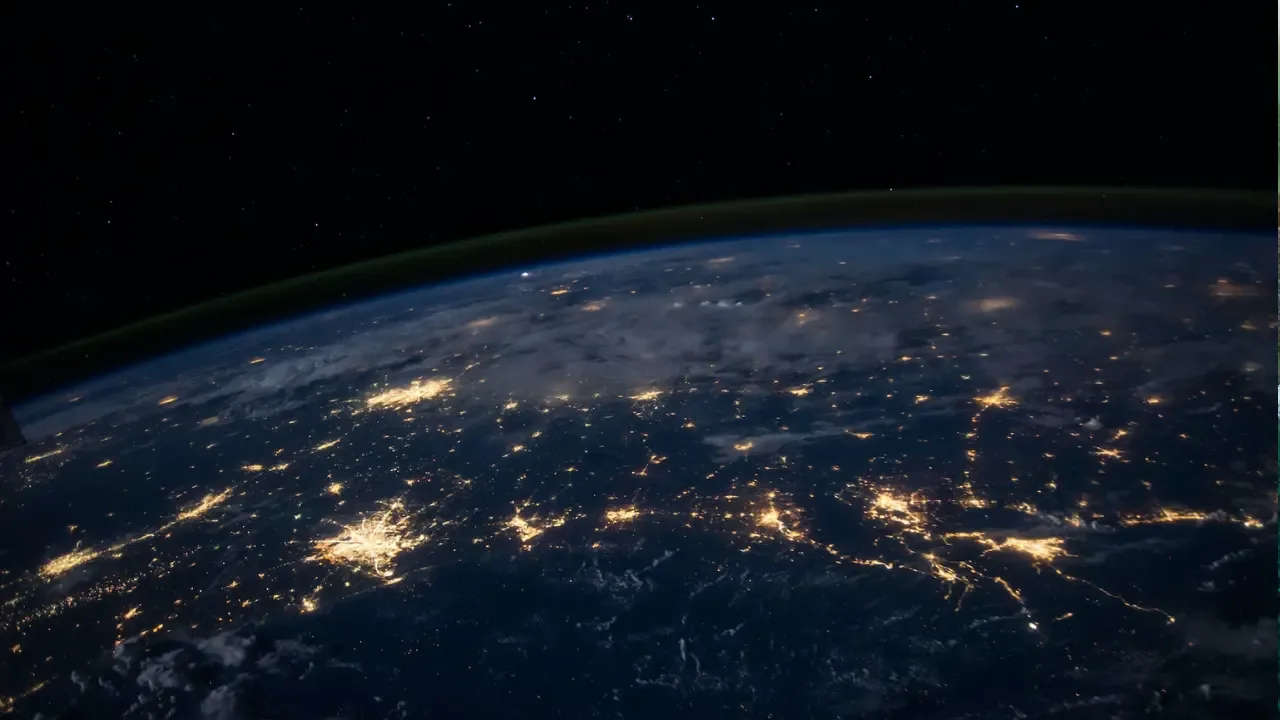
🚀 TypeScript and Field Initializers: Simplifying Class Initialization
Are you tired of spending extra time and effort initializing your TypeScript class fields? Do you wish there was an easier way to achieve the same level of convenience that languages like C# offer? In this blog post, we'll explore the concept of field initializers in TypeScript and how they can make your code more elegant and efficient.
🤷♂️ The Problem
Let's start by understanding the problem at hand. In the given context, the user wants to initialize a new class instance in TypeScript with specific field values, similar to how it's done in C# using object initializers. However, the syntax for achieving this in TypeScript is slightly different, which can be confusing for developers coming from other languages.
💡 The Solution
Fortunately, TypeScript provides an elegant solution to tackle this issue: field initializers. With field initializers, you can conveniently initialize your class fields in a concise and readable manner.
To achieve the desired result, you can follow these steps:
Define your class with the corresponding fields:
class MyClass {
field1: string;
field2: string;
}
Initialize a new class instance using the traditional syntax:
const myClass = new MyClass();
Use field initializers to assign values to the desired fields:
myClass.field1 = "ASD";
myClass.field2 = "QWE";
By doing this, you can easily set the values of your class fields without the need for complex object initializers.
🌟 A Better Approach
While the above solution works perfectly fine, TypeScript also provides an alternative way to simplify field initialization using constructor parameter properties. This approach allows you to declare and initialize class fields in one go.
Here's an example of how you can achieve this:
class MyClass {
constructor(public field1: string, public field2: string) {}
}
const myClass = new MyClass("ASD", "QWE");
In this approach, the public
keyword in the constructor parameters automatically creates and assigns values to the corresponding fields. This cleaner syntax eliminates the need to explicitly assign values after creating the class instance.
📣 Take Action!
By leveraging TypeScript's field initializers or constructor parameter properties, you can significantly simplify your class initialization code. So why not give it a try in your next project? Embrace the elegance and efficiency that TypeScript offers.
Let us know in the comments below if you would like to learn more about TypeScript tricks and best practices. You can also share this blog post with your fellow developers who might find it helpful. Happy coding! 👨💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
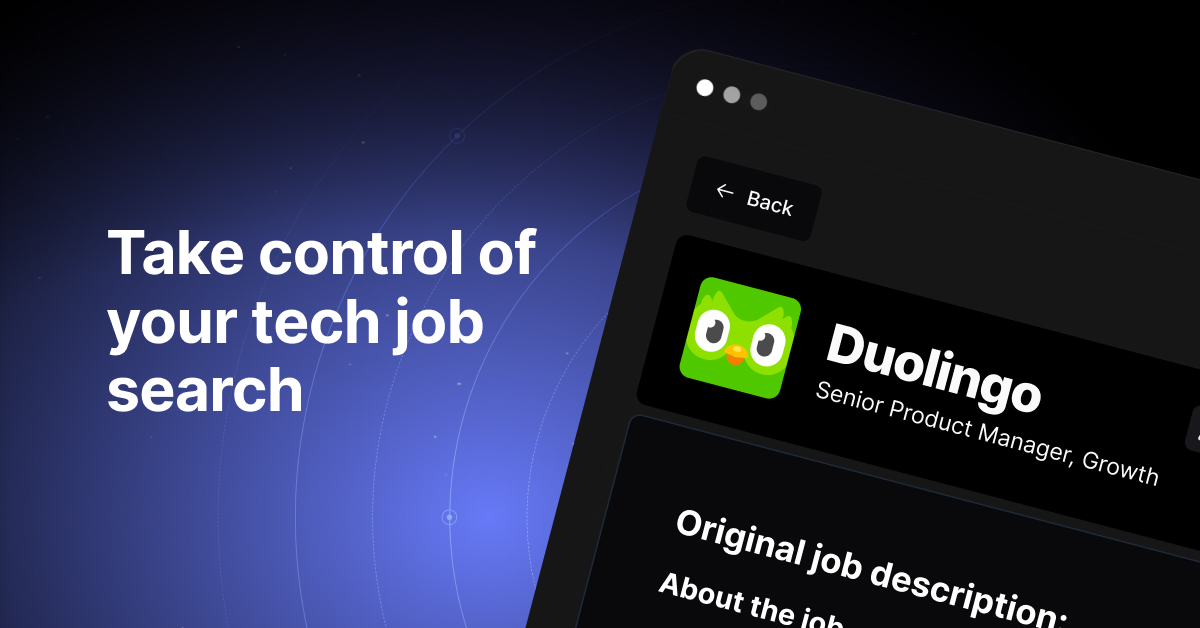