"this" implicitly has type "any" because it does not have a type annotation
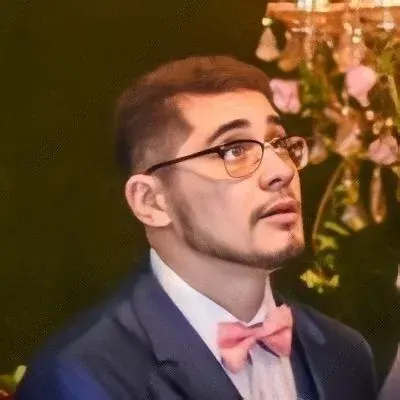
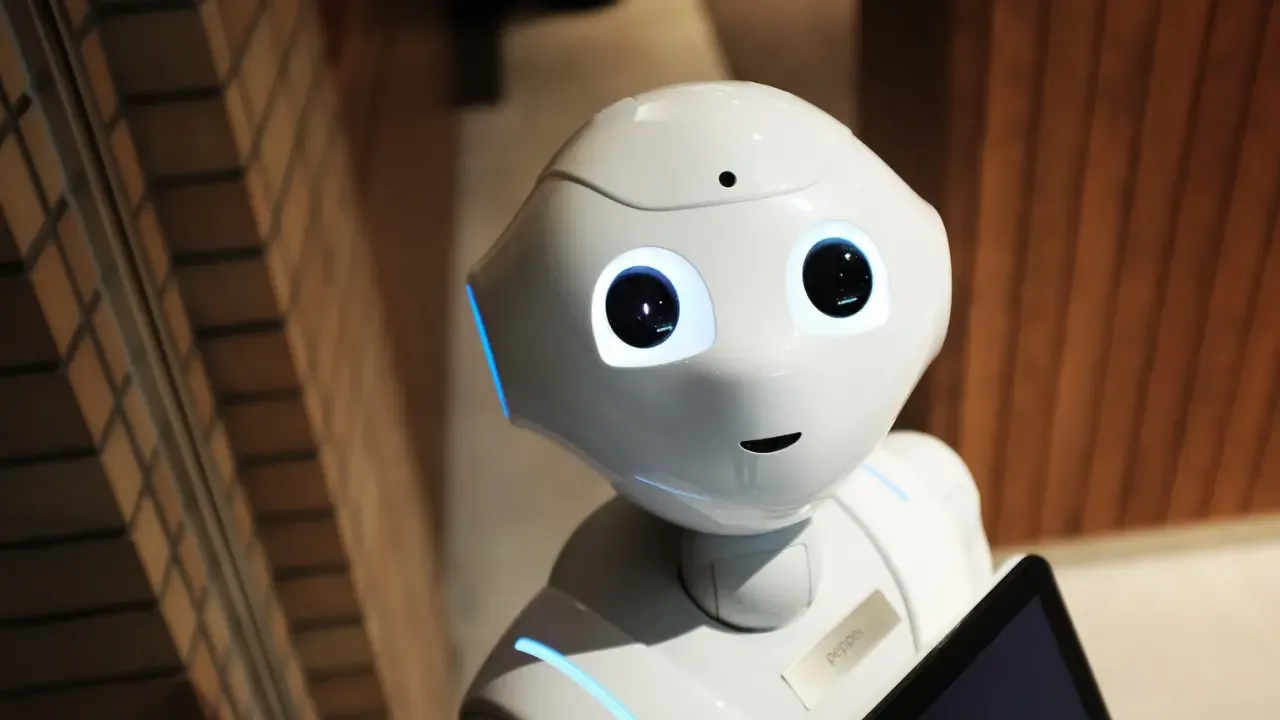
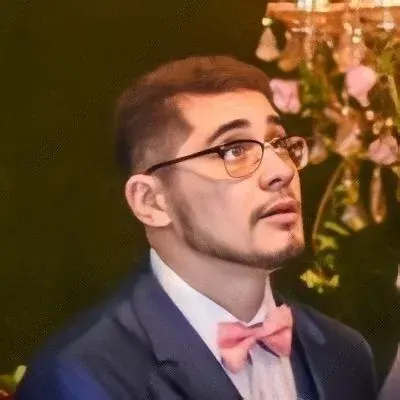
🚀 Solving the Implicit this
Error in TypeScript
Are you encountering the error message "'this' implicitly has type 'any' because it does not have a type annotation" when enabling noImplicitThis
in your tsconfig.json
file? Don't worry, you're not alone! This error is a common stumbling block for many TypeScript developers, but fear not, as we have easy solutions to help you overcome it.
⚠️ The Error
Let's take a look at the code snippet that triggers the implicit this
error:
class Foo implements EventEmitter {
on(name: string, fn: Function) { }
emit(name: string) { }
}
const foo = new Foo();
foo.on('error', function(err: any) {
console.log(err);
this.emit('end'); // error: `this` implicitly has type `any`
});
The error occurs when trying to access this
within a callback function. TypeScript is letting you know that this
does not have a specific type annotation, hence the implicit any
type. Let's explore simple fixes for this problem.
🛠️ Solution 1: Using Explicit Object Reference
One quick workaround is to replace this
with a direct reference to the object:
foo.on('error', (err: any) => {
console.log(err);
foo.emit('end');
});
By explicitly referencing the foo
object instead of using this
, TypeScript no longer complains about the implicit any
type.
🛠️ Solution 2: Adding Type Annotation for this
Another solution involves adding a type annotation specifically for this
within the callback function:
foo.on('error', function(this: Foo, err: any) {
console.log(err);
this.emit('end');
});
By int