Setting default value for TypeScript object passed as argument
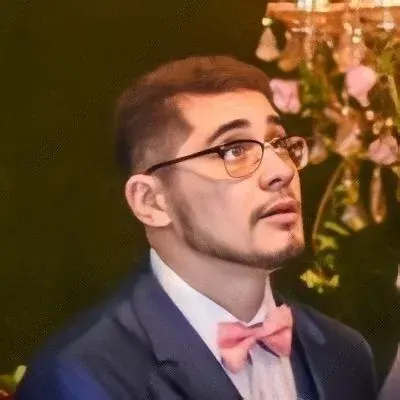
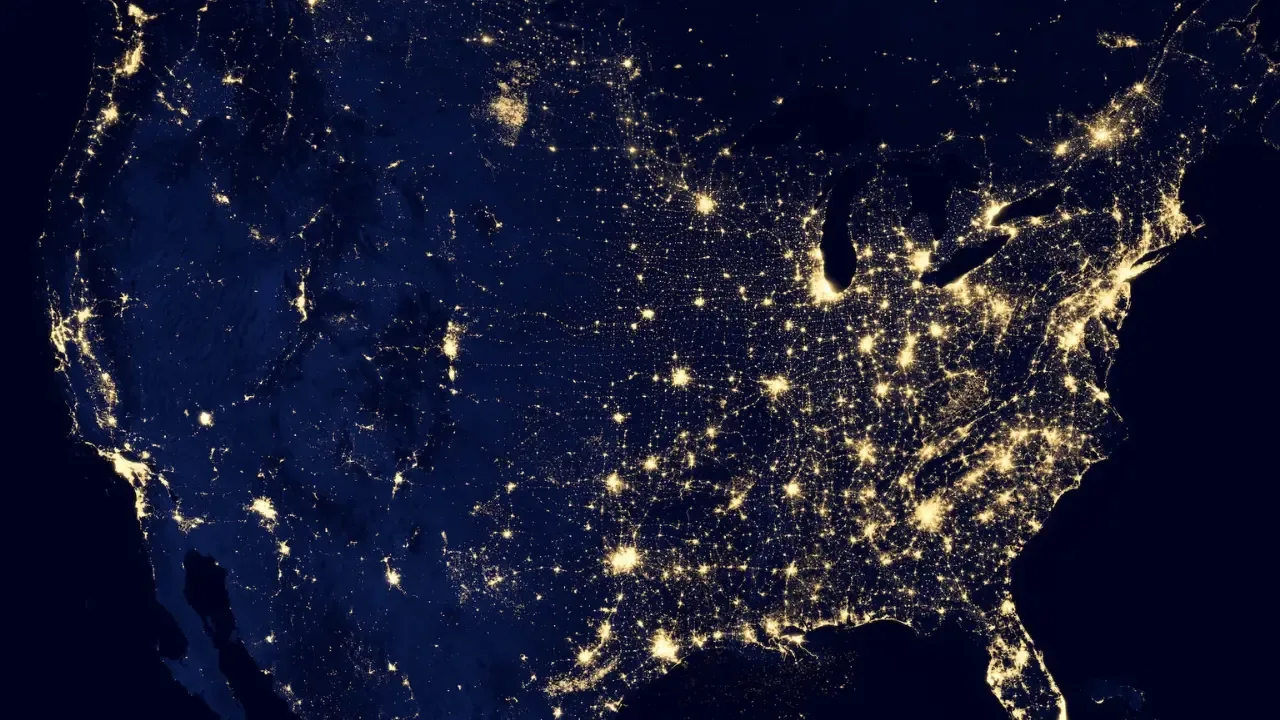
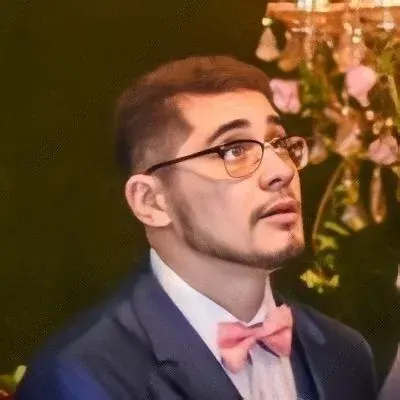
Setting Default Value for TypeScript Object Passed as Argument: The Easy Way
Have you ever encountered the problem of setting a default value for a TypeScript object passed as an argument? 🤔 You're not alone! It's a common issue that many developers face when working with TypeScript. But fear not, because I'm here to guide you through the process and provide easy solutions! 🚀
Let's start by looking at the context surrounding this question:
function sayName(params: {firstName: string; lastName?: string}) {
params.lastName = params.lastName || 'smith'; // <<-- any better alternative to this?
var name = params.firstName + params.lastName
alert(name);
}
sayName({firstName: 'bob'});
In the above code, we have a function called sayName
that takes in an object as a parameter. The object has two properties: firstName
and lastName
. The issue at hand is that if lastName
is not provided, we want to set a default value of 'smith'. The current solution is to use the logical OR operator (||
) to check if lastName
is falsy and then assign the default value. But is there a better alternative? 🤔
The Easy Solution
Yes, indeed there is! TypeScript allows us to set default values directly in the function signature, making our code cleaner and more concise. Here's how it's done:
function sayName(params: {firstName: string; lastName: string = 'smith'}) {
// Rest of the code
}
In this updated code, we have added = 'smith'
after the lastName
property in the function signature. This tells TypeScript that the default value for lastName
should be 'smith'. Now, if lastName
is not provided when calling the function, it will automatically be set to 'smith'. 🎉
But What About Regular Arguments?
You might be wondering, what if these were regular arguments instead of an object? Is there a way to set default values for them? Absolutely! TypeScript provides a simpler syntax for setting default values for regular arguments. Let's take a look:
function sayName(firstName: string, lastName = 'smith') {
// Rest of the code
}
sayName('bob');
In this code snippet, we have defined lastName
as a regular argument with a default value of 'smith'. Notice the = 'smith'
syntax? It does the same trick as before – if lastName
is not provided, it will default to 'smith'. 🌟
The CoffeeScript Alternative
If you're familiar with CoffeeScript, you may have encountered a similar situation. CoffeeScript provides the conditional existence operator (?=
) that allows you to set default values for properties in an object. Let's see how it translates to JavaScript:
param.lastName ?= 'smith'
The above CoffeeScript code compiles to the following JavaScript code:
if (param.lastName == null) {
param.lastName = 'smith';
}
As you can see, CoffeeScript provides a clean and concise way to set default values for object properties. It's always good to explore alternatives and see what suits your coding style and preferences! 👍
In Conclusion
Setting default values for TypeScript objects passed as arguments doesn't have to be complicated. By using TypeScript's default value syntax or exploring alternatives like CoffeeScript, you can make your code more readable and concise. So go ahead and give it a try in your next project! 💪
If you have any questions or alternative solutions, feel free to share them in the comments section below. Let's learn from each other! 🌟