Iterating over Typescript Map
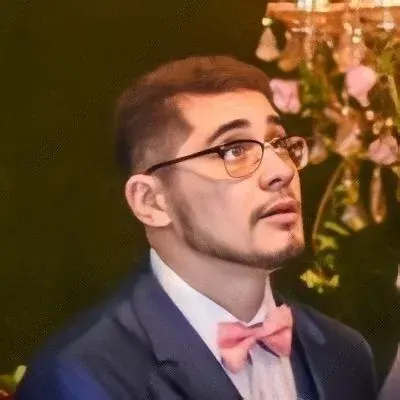
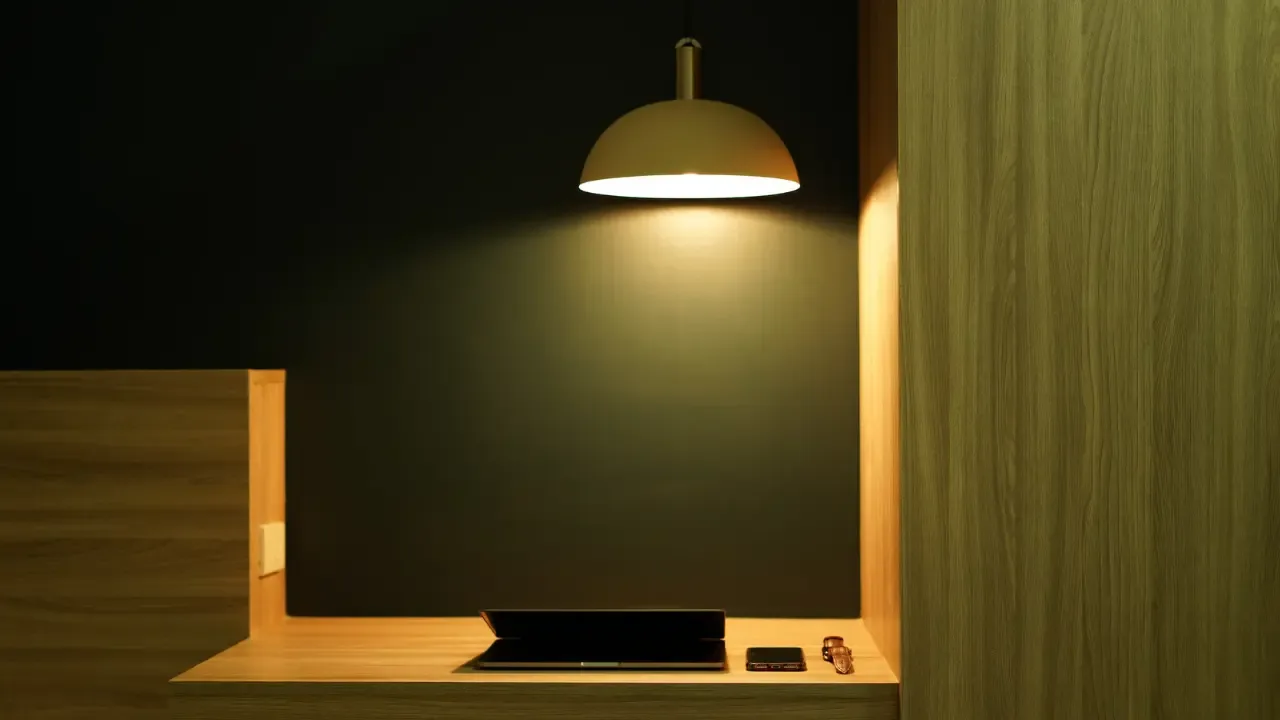
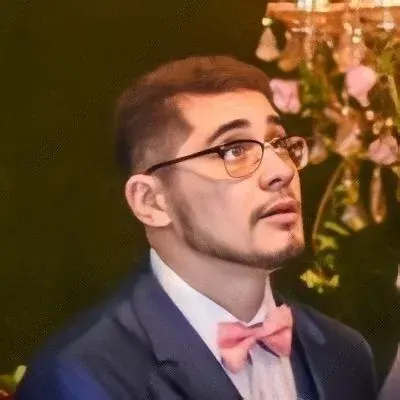
Iterating over TypeScript Map
So you're trying to iterate over a TypeScript Map and you're facing some errors? 😫 Don't worry, you're not alone! This seemingly trivial problem can sometimes cause headaches. But fear not, because we're here to help you solve it! 🙌
The Error
Let's first take a look at the error message you encountered:
Type 'IterableIteratorShim<[string, boolean]>' is not an array type or a string type.
This error message is telling you that the type returned by myMap.keys()
is not compatible with the for...of
loop. TypeScript expects an iterable array or string for the loop, but it seems that myMap.keys()
is returning an IterableIteratorShim<[string, boolean]>
instead.
The Solution
To overcome this error, we need to convert the IterableIteratorShim<[string, boolean]>
into something compatible with the for...of
loop. Luckily, there are a couple of straightforward solutions:
Solution 1: Convert Iterator to Array
const keysArray = Array.from(myMap.keys());
for (const key of keysArray) {
console.log(key);
}
In this solution, we convert the iterator object returned by myMap.keys()
into an array using the Array.from()
method. This array can then be used to iterate over the keys using the for...of
loop. Problem solved! 🎉
Solution 2: Spread Operator
const keysArray = [...myMap.keys()];
for (const key of keysArray) {
console.log(key);
}
Alternatively, we can use the spread operator (...
) to convert the iterator object into an array. This approach achieves the same result as the previous solution but in a more concise way.
The Call-to-Action
We hope this guide has helped you tackle the common issue of iterating over a TypeScript Map. If you found this information useful, why not share it with others who might be facing similar problems? Spread the knowledge! ✨
Also, if you have any other TypeScript questions or if you've encountered a different issue, feel free to leave a comment below. Let's help each other out! 😊