Is there a way to "extract" the type of TypeScript interface property?
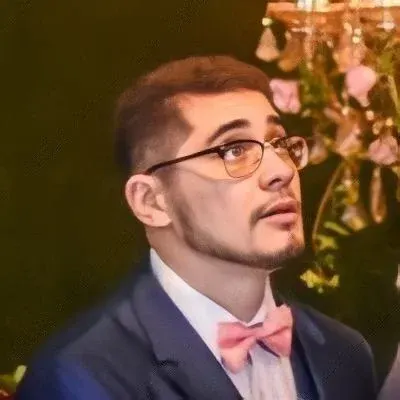
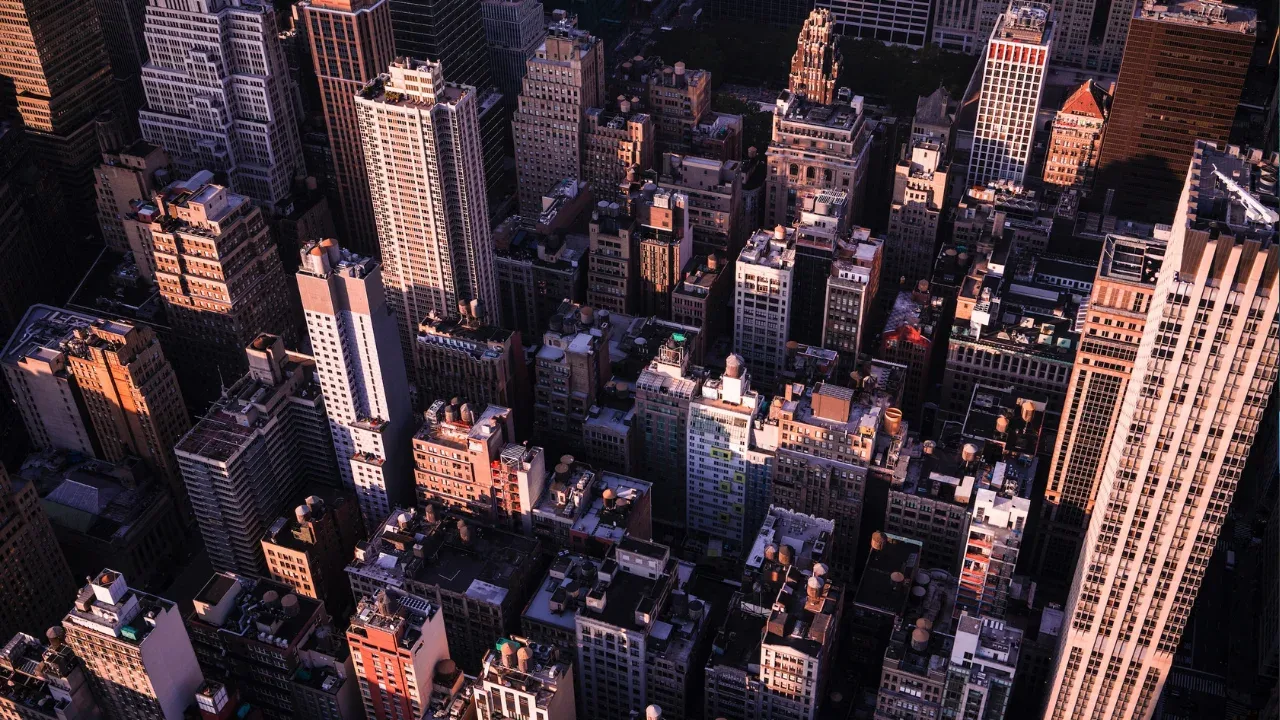
📝 Blog Post: Extracting TypeScript Interface Property Type
Hey there, fellow tech enthusiasts! 👋 Are you struggling with extracting the type of a property from a TypeScript interface? Fear not, as we're here to shed some light on this common issue and provide you with easy solutions! 💡
The Problem
Let's dive into a real-world scenario. Imagine you're working with a library called X that has its own typing file, which includes some interfaces. We'll focus on the following interfaces:
interface I1 {
x: any;
}
interface I2 {
y: {
a: I1,
b: I1,
c: I1
}
z: any
}
You need to pass around an object that has the exact same type as I2.y
.
The Traditional Approach
An immediate solution might be to create an identical interface in your source files. This would look something like:
interface MyInterface {
a: I1,
b: I1,
c: I1
}
let myVar: MyInterface;
However, this approach has some downsides. Firstly, you would have to manually keep your interface up to date with any changes made in the library's interface. Secondly, in complex scenarios with large interfaces, this can result in a lot of code duplication, making maintenance a headache. 😫
The Quest for a Better Solution
So, how can we extract the type of this specific property from the interface without all the burden and duplication? Good question! 🤔
Unfortunately, the simple approach let myVar: typeof I2.y
won't work and will result in a "Cannot find name I2" error. 😞
The Smart Solution
But don't lose hope just yet! After some tinkering in the TypeScript Playground, our brave coder friend stumbled upon a clever solution:
declare var x: I2;
let y: typeof x.y;
By declaring a redundant variable x
as the interface I2
, we can then extract the type of the specific property y
as typeof x.y
. Voila! 🎉
An Even Better Solution? 🤔
But, hey, who likes redundant variables, right? Our heroic coder friend is now on the lookout for a way to achieve this extraction without the need for that extra declaration. If you have any insights or alternative solutions, we'd love to hear from you! 😉
So, there you have it! Now you can extract the type of a TypeScript interface property and save yourself from the burdens of manual updates and code duplication. Remember, there's always a way to simplify complex problems! 💪
If you found this guide helpful or have any thoughts to share, feel free to leave a comment below. Keep coding and keep exploring! ✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
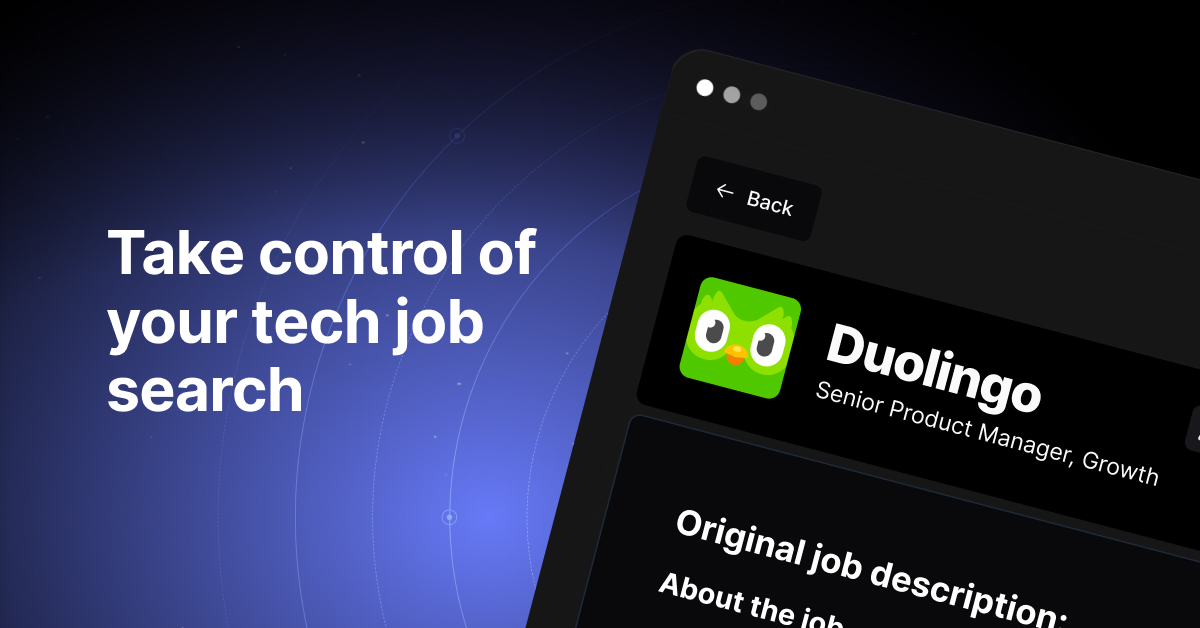