Is there a `valueof` similar to `keyof` in TypeScript?
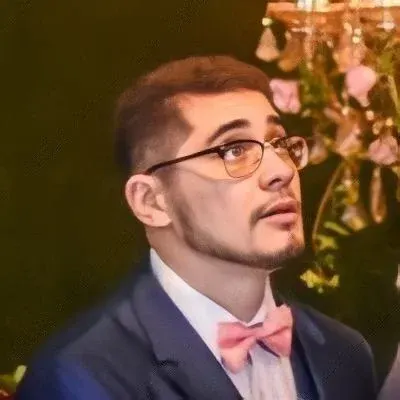
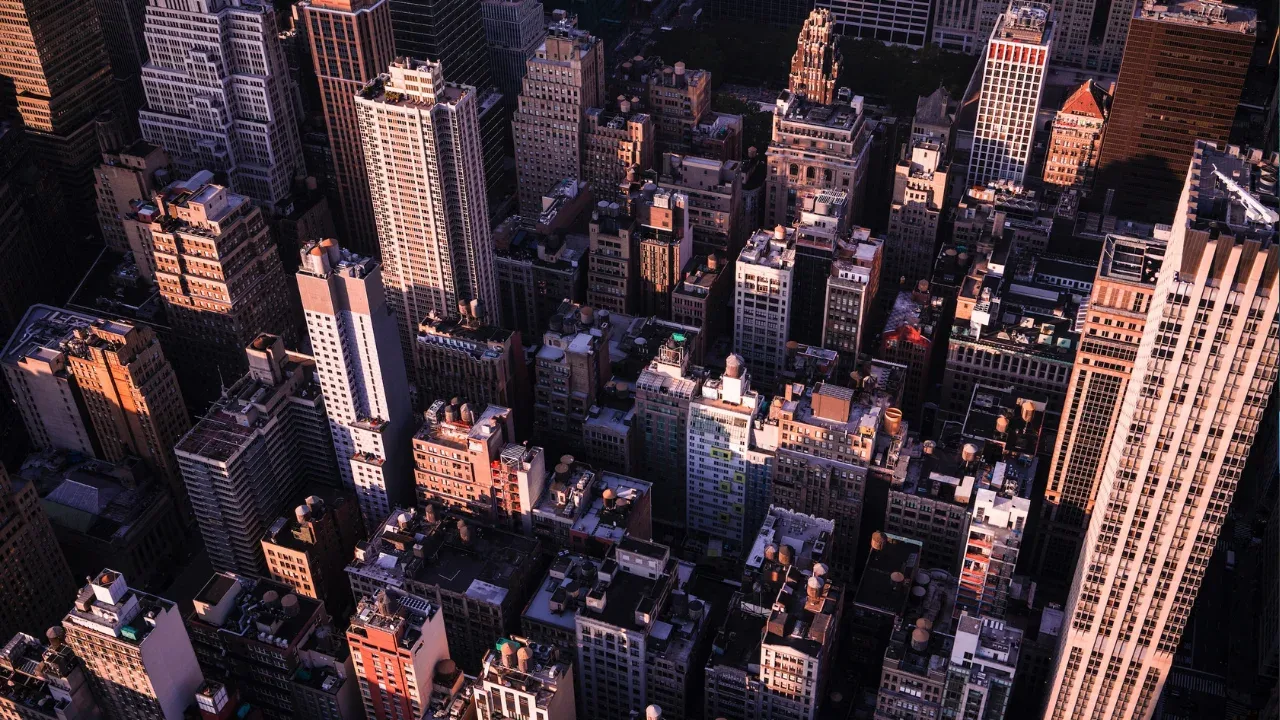
š Blog Post: Is there a valueof
similar to keyof
in TypeScript?
š Hey there fellow TypeScript developers! š„ļø Do you find yourself wondering if there is a similar valueof
type in TypeScript just like the keyof
type? š¤ Well, you've come to the right place! In this blog post, we will explore this question, address common issues, provide easy solutions, and help you tackle this problem with ease! šŖ
š Problem Scenario: Understanding the Issue
Alright, let's dive into the problem scenario. 𤿠The code given showcases an object JWT
with properties id
, token
, and expire
. The aim is to assign an object property to a value based on given key-value inputs while still being able to determine the type of the assigned value. This might sound a bit tricky, so let's take a look at the code snippet:
type JWT = { id: string, token: string, expire: Date };
const obj: JWT = { id: 'abc123', token: 'tk01', expire: new Date(2018, 2, 14) };
function print(key: keyof JWT) {
switch (key) {
case 'id':
case 'token':
console.log(obj[key].toUpperCase());
break;
case 'expire':
console.log(obj[key].toISOString());
break;
}
}
function onChange(key: keyof JWT, value: any) {
switch (key) {
case 'id':
case 'token':
obj[key] = value + ' (assigned)';
break;
case 'expire':
obj[key] = value;
break;
}
}
print('id');
print('expire');
onChange('id', 'def456');
onChange('expire', new Date(2018, 3, 14));
print('id');
print('expire');
onChange('expire', 1337); // should fail here at compile time
print('expire'); // actually fails here at run time
From the code above, we can see that the functions print
and onChange
are used to print or modify the values of the given object properties respectively.
The challenge lies in making sure that the assigned value in the onChange
function matches the type of the targeted property. šÆ By default, the value
parameter is declared as any
, allowing any type of value to be assigned, which is not what we want.
So, the question arises: How can we enforce the assignment of the correct type for the given property value? Let's find out!
šµļøāāļø Solving the Problem: Introducing the valueof
Type
One approach we might consider is using a valueof
type, similar to the keyof
type in TypeScript. However, unfortunately, TypeScript doesn't provide a built-in valueof
type like keyof
. š But worry not, we can come up with a workaround to achieve the desired result!
To solve this, we can create a custom type utility called ValueOf
, which allows us to retrieve the value types of a given object type:
type ValueOf<T> = T[keyof T];
By using this ValueOf
utility, we can now update the onChange
function to specify the correct value type for the given key:
function onChange(key: keyof JWT, value: ValueOf<JWT>) {
// ...
}
š And there you have it! With this simple modification, TypeScript will now enforce that the assigned value in the onChange
function matches the type of the targeted property. š¦
⨠Engagement: Let's Connect!
We hope this blog post helped you understand the problem and provided a simple yet effective solution using a custom ValueOf
type utility. TypeScript is a powerful language, and sometimes it requires a bit of creativity to overcome certain challenges. š
Now, it's your turn! š¢ We would love to hear from you. Have you encountered any similar issues in TypeScript? What creative solutions did you come up with? Share your experiences and insights in the comments section below!
If you found this blog post helpful, don't forget to share it with your fellow TypeScript devs. Let's spread the knowledge and make TypeScript development a breeze for everyone! š¤
Happy coding! š»āØ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
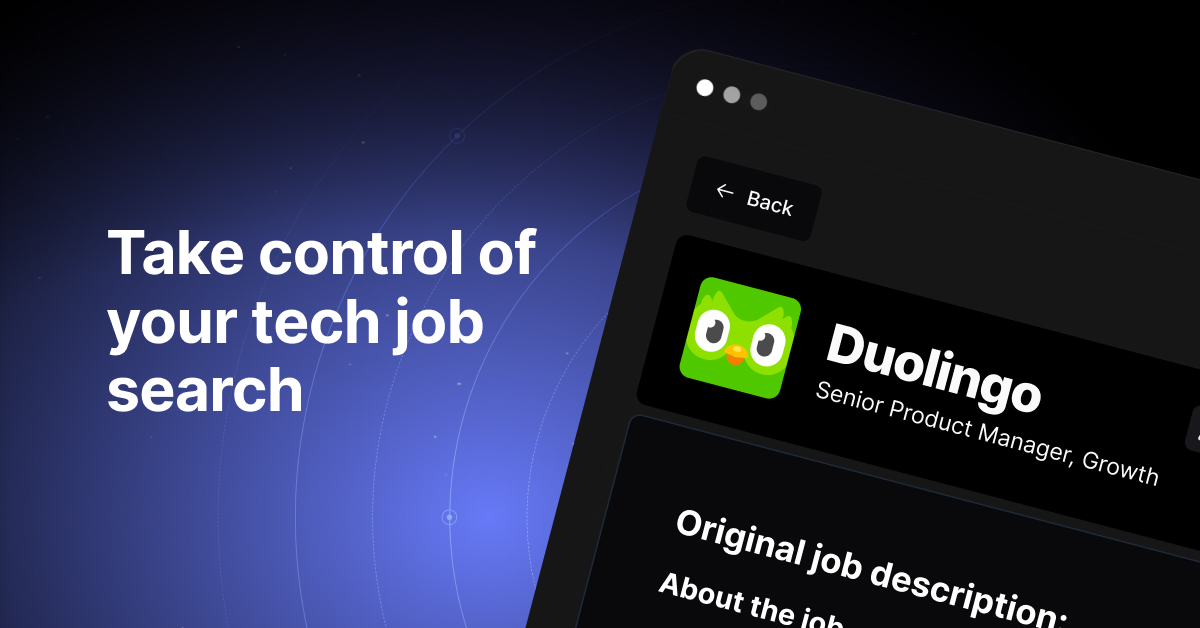