In Typescript, How to check if a string is Numeric
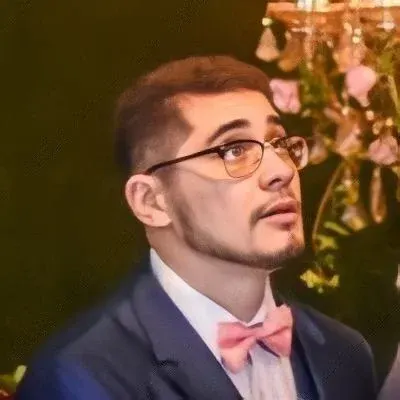
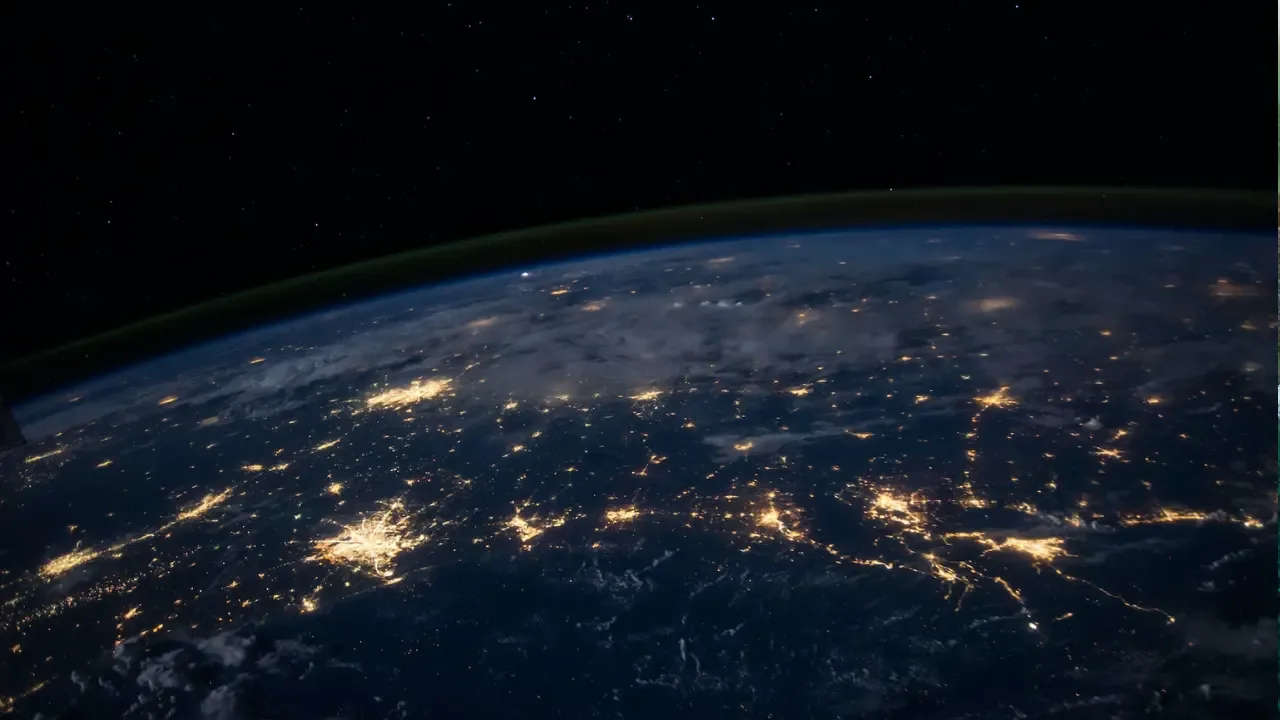
š Check if a String is Numeric in TypeScript
šÆ Problem
So you're working with TypeScript and you want to check if a string is numeric. You tried using the isNaN
function, but it throws an error when you pass a non-numeric string. You also noticed that using parseFloat
returns false instead of throwing an error. You want to find a better way to perform this check without encountering any issues.
š Understanding the Issue
The isNaN
function in TypeScript only accepts numeric values. When you pass a non-numeric string, it throws an error. On the other hand, parseFloat
tries to convert the string to a numeric representation. If it fails, it returns NaN
. However, if it succeeds, it returns the numeric value without throwing an error.
š” Easy Solution
To overcome this issue, you can use a modified function that wraps the parseFloat
function and performs additional checks on the result. Here's the modified function that you can use:
static isNaNModified = (inputStr: string) => {
var numericRepr = parseFloat(inputStr);
return isNaN(numericRepr) || numericRepr.toString().length != inputStr.length;
}
š Explanation
isNaNModified
is a static function that takesinputStr
as its parameter.It uses
parseFloat(inputStr)
to convert the string to a numeric representation.The function checks if
numericRepr
isNaN
usingisNaN(numericRepr)
.The function also compares the length of
numericRepr.toString()
withinputStr.length
.If they're not equal, it means there are non-numeric characters present in the string, so the function returns
true
.If they're equal, it means the string is entirely numeric, so the function returns
false
.
š Call-to-Action Now that you understand how to check if a string is numeric in TypeScript, try implementing it in your project. If you have any other questions or need further assistance, feel free to leave a comment below. Happy coding! š
āļø Engage with Us We love interacting with our readers! If you found this post helpful or have any suggestions, don't hesitate to share them in the comments. Let's continue the discussion, learn from each other, and grow together as developers. šŖ
š£ Share the Knowledge If you think this post could benefit someone else, spread the word! Share it on your favorite social media platforms or with your fellow developers. Let's help more people overcome this common TypeScript issue.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
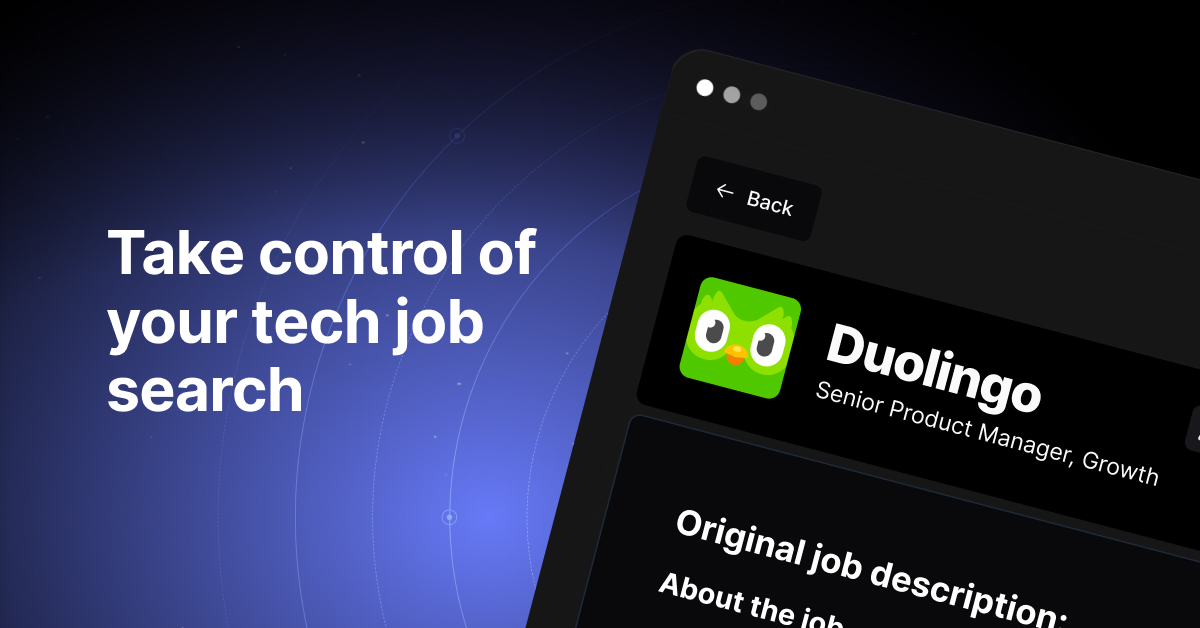