How to use moment.js library in angular 2 typescript app?
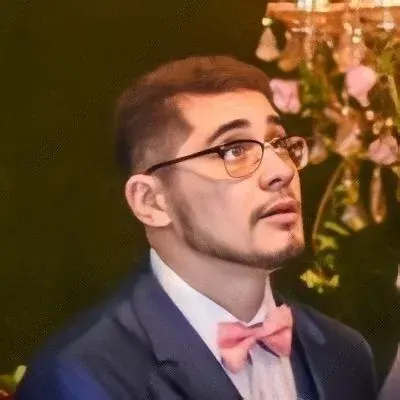
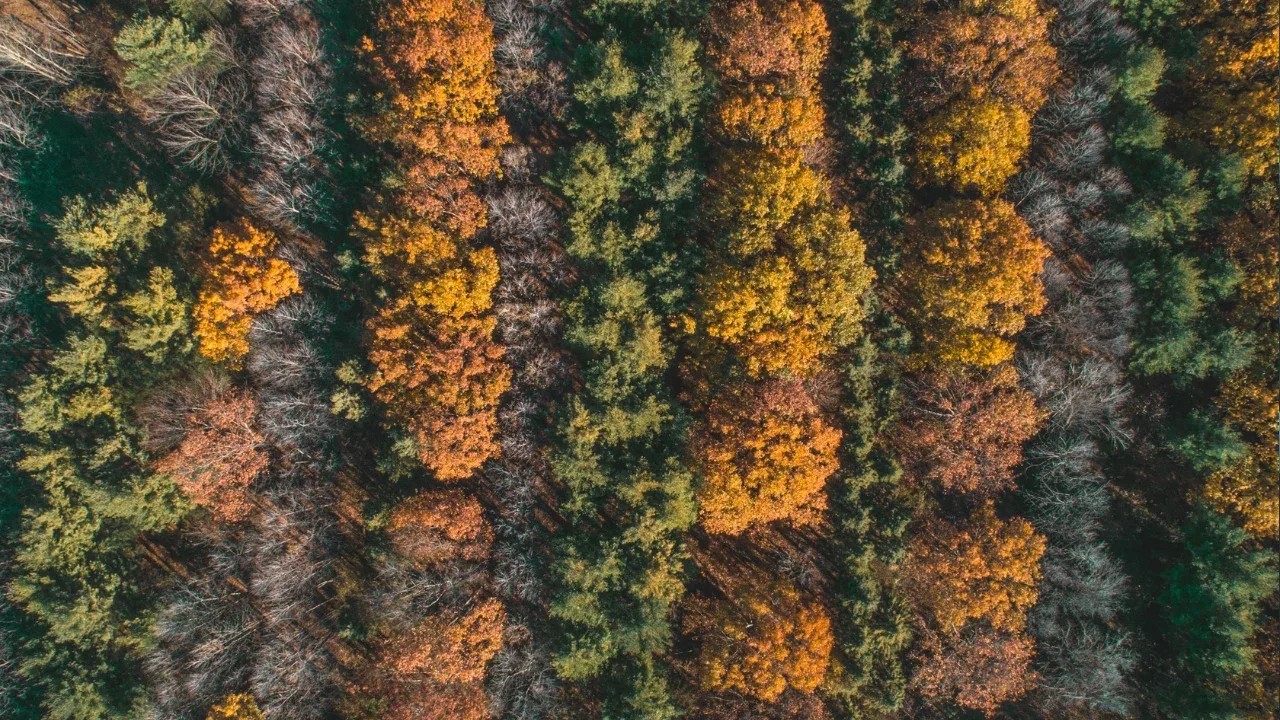
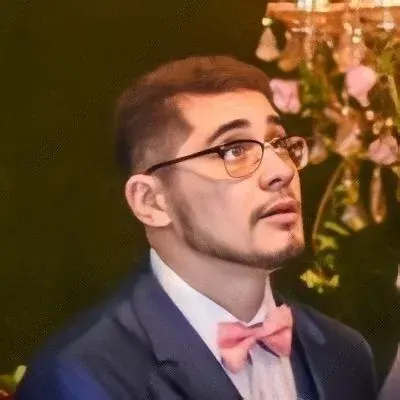
How to Use the Moment.js Library in an Angular 2 TypeScript App 😎
Are you trying to add the Moment.js library to your Angular 2 app written in TypeScript but encountering errors when calling the moment.format()
function? Don't worry, we've got you covered! In this guide, we will walk you through the steps to successfully use the Moment.js library in your Angular 2 TypeScript app. Let's dive right in! 🚀
Step 1: Installing Moment.js and TypeScript Bindings
The first step is to install Moment.js and its TypeScript bindings. Open your terminal and run the following commands:
npm install moment --save
typings install moment --ambient --save
This will install the Moment.js library and its TypeScript bindings, saving them as dependencies in your project.
Step 2: Importing Moment.js in Your App
Next, let's import Moment.js in your TypeScript file. Depending on your setup, you have two options:
Option 1: Using ES6 Modules
If you are using ES6 modules in your app, add the following line at the top of your TypeScript file:
import * as moment from 'moment';
This line imports the Moment.js library and assigns it to the variable moment
. Now, you can use the Moment.js functions and methods in your app.
Option 2: Using CommonJS Modules
If you are using CommonJS modules, use the following line instead:
const moment = require('moment');
This line requires the Moment.js library and assigns it to the variable moment
.
Step 3: Using Moment.js Functions
Now that you have imported Moment.js in your app, you can use its functions and methods. For example, let's format the current date using the moment.format()
function:
const currentDate = moment().format('YYYY-MM-DD');
console.log(currentDate);
The moment().format()
function retrieves the current date and formats it according to the provided format string. In this case, we used the format YYYY-MM-DD
to display the date in the "Year-Month-Day" format.
Troubleshooting Common Issues
If you still encounter errors when using Moment.js, here are a few common issues and solutions:
Issue: "Cannot find module 'moment' or its corresponding type declarations"
Solution:
Ensure you have installed Moment.js and its TypeScript bindings correctly using the commands mentioned in Step 1.
Issue: "TypeError: moment_1.default is not a function"
Solution:
If you see this error, it means you are using the CommonJS modules import syntax (
const moment = require('moment')
) in an ES6 modules setup. Switch to the ES6 modules import syntax (import * as moment from 'moment'
) mentioned in Step 2.
Conclusion and Actionable Next Steps
Congratulations! You have successfully integrated the Moment.js library into your Angular 2 TypeScript app. 🎉 Feel free to explore the wide range of features and functions Moment.js offers to handle dates and times effortlessly in your app.
If you found this guide helpful, please share it with other developers who might be facing similar issues. Also, feel free to leave a comment below if you have any questions or need further assistance. We love to hear from you! 😊
Stay tuned for more exciting tutorials and tips on our tech blog! 🌟