How to require a specific string in TypeScript interface
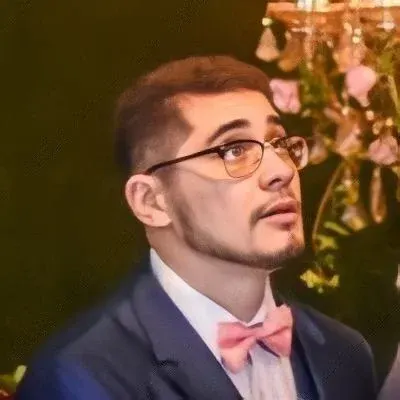
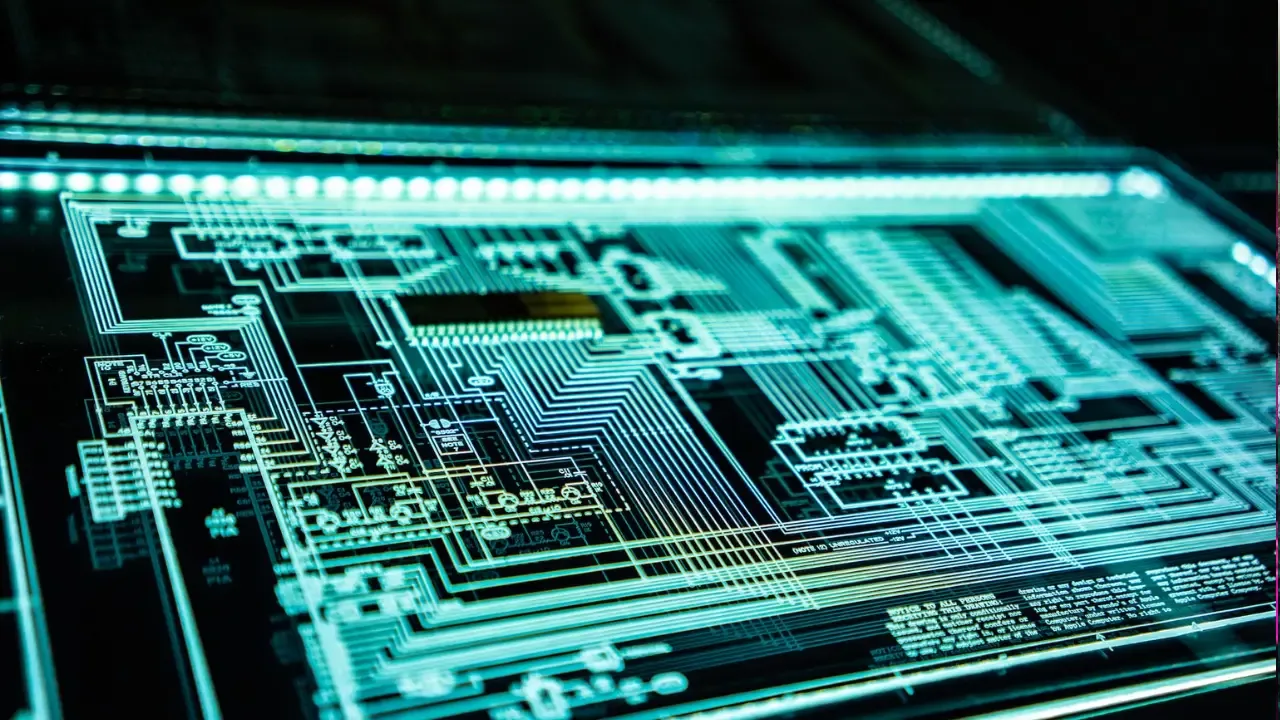
How to Require a Specific String in TypeScript Interface 🤔
So you're creating a TypeScript definition file for a 3rd party JavaScript library and you encounter a situation where one of the properties in an options object should only accept specific strings. You want the TypeScript interface to enforce this validation, allowing only strings from a predefined list.
The Challenge 🧠
The specific challenge you're facing is to ensure that the brace_style
property of the IOptions
interface only accepts strings from the list: "collapse"
, "expand"
, "end-expand"
, and "none"
.
The Solution 💡
Luckily, TypeScript provides a way to enforce such restrictions through the use of union types.
To achieve this, you can update your IOptions
interface like this:
interface IOptions {
indent_size?: number;
indent_char?: string;
brace_style?: "collapse" | "expand" | "end-expand" | "none";
}
By using the |
(pipe) symbol between each string value, you are creating a union type that restricts the property to only accept one of the predefined strings.
Example Usage 💻
Let's see how this works in practice. Consider the following code snippet:
const options1: IOptions = {
indent_size: 2,
indent_char: " ",
brace_style: "expand"
}; // This is valid, brace_style is set to "expand"
const options2: IOptions = {
indent_size: 4,
indent_char: "\t",
brace_style: "invalid"
}; // This will give a TypeScript error, as "invalid" is not a valid brace_style
As you can see, TypeScript enforces the restriction and throws an error when an invalid string is assigned to the brace_style
property.
The Call-to-Action ⚡️
Now that you know how to require a specific string in a TypeScript interface, it's time to put this knowledge into practice. Go ahead and update your TypeScript code to use union types for strict string validation in your interfaces!
Share this blog post with your fellow TypeScript enthusiasts and let's spread the knowledge! 😄 If you have any questions or experiences to share, leave a comment below. Happy coding! 🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
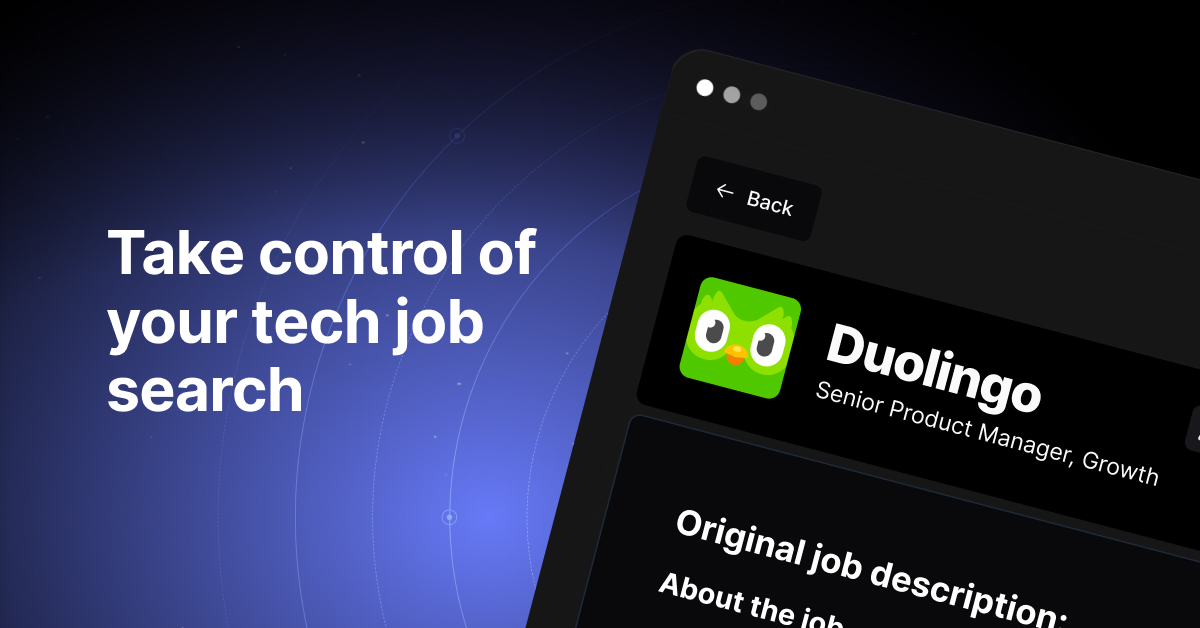