How to reject in async/await syntax?
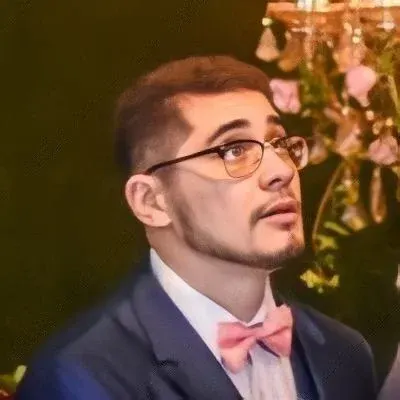
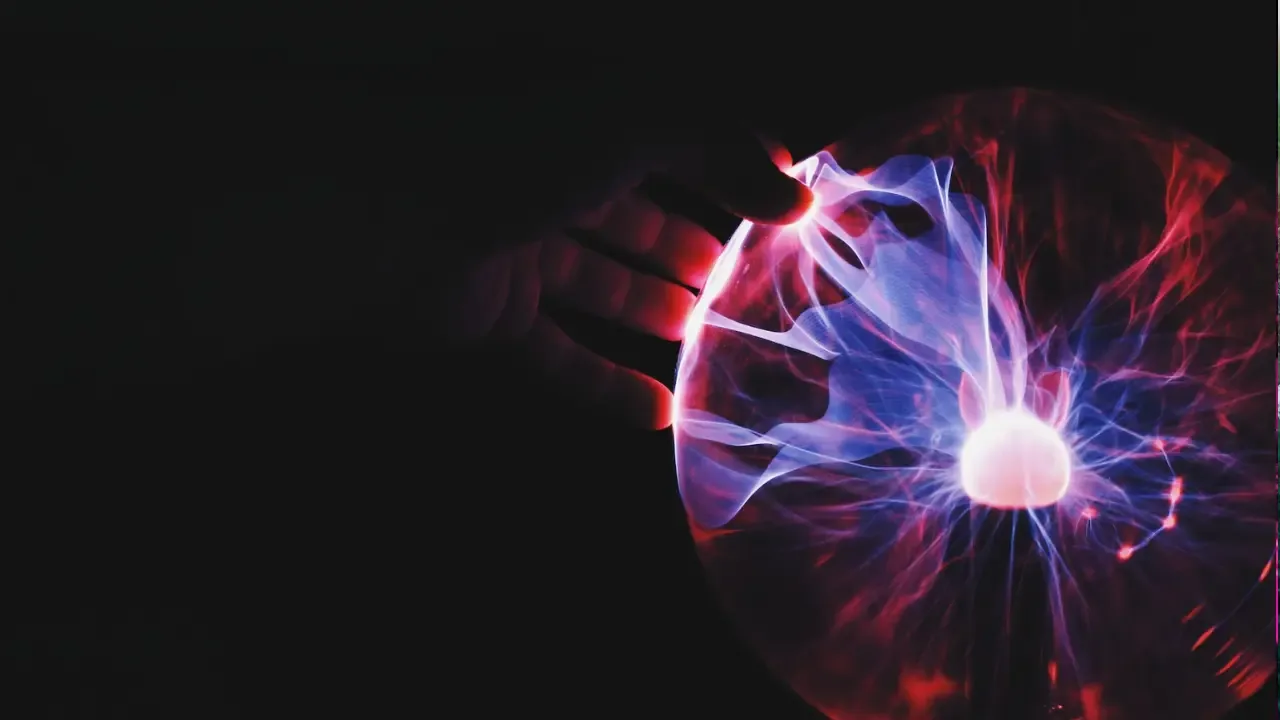
How to Reject in Async/Await Syntax? 😕
So you've just switched your code from using traditional promise syntax to the shiny new async/await
syntax. You're feeling pretty good about it, but then you run into a problem - how do you properly reject a promise in async/await
syntax? 😳
Don't worry, I've got you covered! In this article, I'll show you how to handle promise rejections in async/await
syntax and provide easy solutions to common issues you may encounter. Let's dive in! 💪
The Problem 🤔
In the code example you provided, you're using the async/await
syntax for a function called foo(id: string): Promise<A>
. If an error occurs inside the try
block, you want to properly reject the returned promise. However, simply returning the rejected value like return 400
doesn't work as expected. 😫
The Solution 🙌
To properly reject the promise, you need to throw
an error within the catch
block. Here's how you can do it:
async foo(id: string): Promise<A> {
try {
await someAsyncPromise();
return 200; // Resolves the promise with the value 200
} catch (error) {
throw new Error("Some error message"); // Rejects the promise with an error
}
}
Instead of returning the rejected value directly, we throw a new Error
object with a descriptive error message. This will cause the promise to be rejected and allow you to handle the error appropriately in the calling code. 🚀
Example Usage 🌟
Let's see how you can use the modified foo
function with proper promise rejection handling:
try {
const result = await foo("someId");
console.log(result); // If the promise resolves, prints: 200
} catch (error) {
console.error(error.message); // If the promise rejects, prints: Some error message
}
By wrapping the await
expression in a try/catch
block, you can catch any rejected promise and gracefully handle the error. Pretty neat, right? 😎
Takeaways and Call-to-Action 📝
Rejecting promises in async/await
syntax requires a slightly different approach compared to traditional promise syntax. Instead of returning the rejected value directly, you need to throw
an error to properly reject the promise.
Remember these key points:
Use
throw new Error("Your error message")
inside thecatch
block to reject the promise.Wrap the
await
expression in atry/catch
block to handle any rejected promise.
Now that you know how to properly reject promises in async/await
syntax, go forth and write cleaner, more elegant asynchronous code! 🙌 And don't forget to share this article with your fellow developers to spread the knowledge.
If you have any other questions or want to share your own tips, feel free to leave a comment below. Happy coding! 😄💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
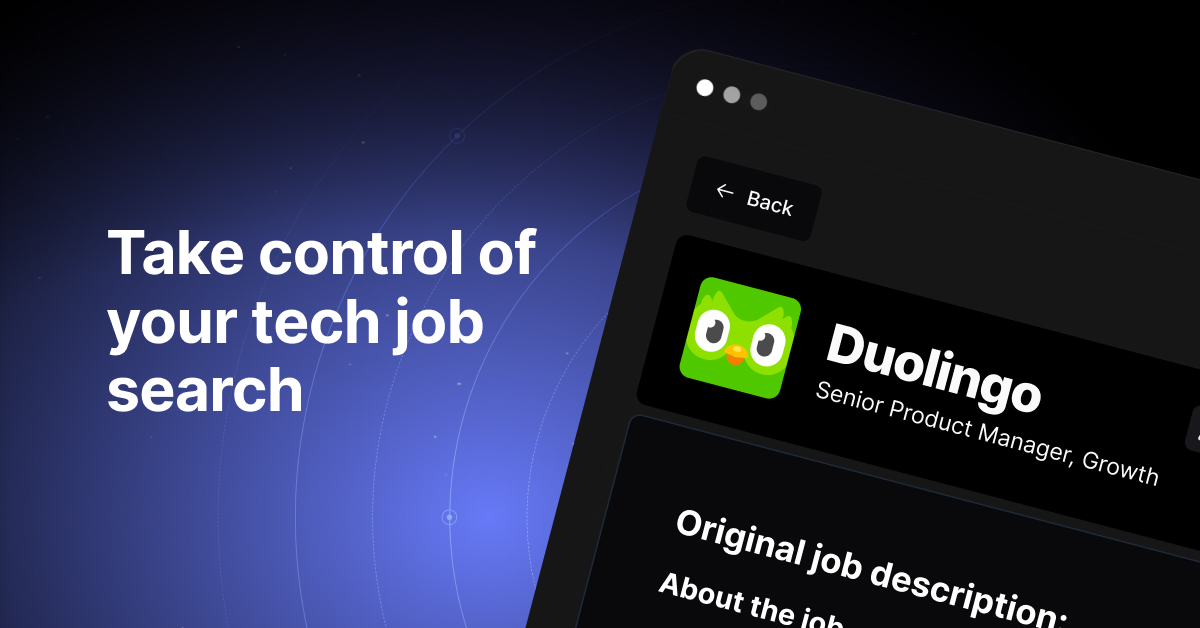