How to get query params from url in Angular 2?
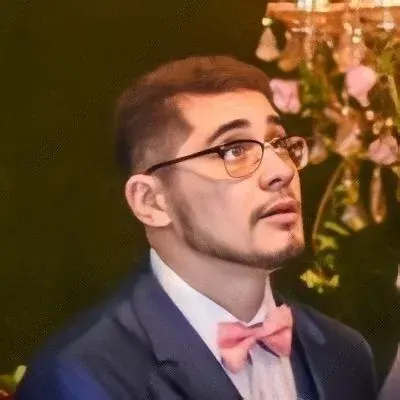
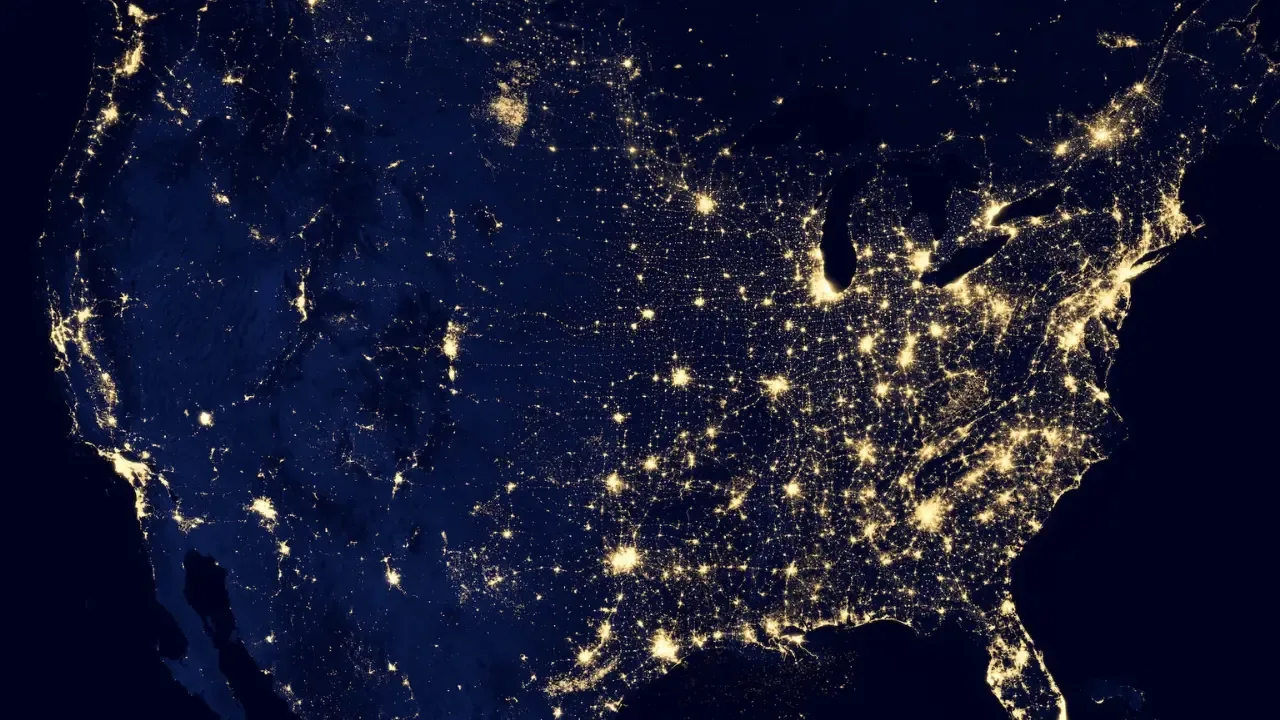
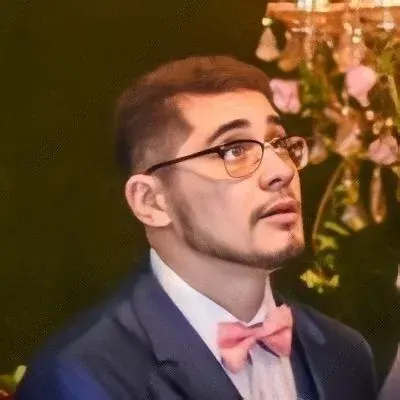
How to Get Query Params from URL in Angular 2?
So you're building a fancy Angular 2 application and you've come across a problem - you're not able to get those precious query parameters from the URL. 😱 Don't worry, we've got you covered!
The Problem
Let's take a look at the scenario. You have a component that is loaded on a specific path, say /path?query=value1
. But when the component is loaded, the query parameters are mysteriously stripped away and you're left with just /path
. So why did this happen? And more importantly, how can you preserve those valuable query parameters?
The Solution
Fear not, my fellow developer! The solution to this problem lies in the Angular 2 Router
. To preserve the query parameters, you need to use the queryParamsHandling
property of the NavigationExtras
object.
Here's an example of how you can preserve the query parameters:
import { Router, NavigationExtras } from '@angular/router';
// Inside your component
constructor(private router: Router) {}
// When navigating to a new route
preserveQueryParams() {
const queryParamsHandler: NavigationExtras = {
queryParamsHandling: 'preserve'
};
this.router.navigate(['/new-route'], queryParamsHandler);
}
By setting the queryParamsHandling
property to 'preserve'
, you ensure that the query parameters from the current route are preserved when navigating to the new route.
A Specific Problem
But what if you're facing a specific issue where you're unable to get the query parameters in a child route component? Let's take a closer look at this problem.
In your route configuration, you might have a main route and a child route. Here's an example:
// Main route
@RouteConfig([
{
path: '/todos/...',
name: 'TodoMain',
component: TodoMainComponent
}
])
// Child route
@RouteConfig([
{ path: '/', component: TodoListComponent, name: 'TodoList', useAsDefault: true },
{ path: '/:id', component: TodoDetailComponent, name: 'TodoDetail' }
])
In this case, you're not able to get the query parameters in the TodoListComponent
. 😔
But fret not, my friend! We have a workaround for this. Instead of using the classic query parameters, you can utilize the params
method to get the parameters from the URL.
Here's an example of how you can achieve this:
import { ActivatedRoute } from '@angular/router';
// Inside your component
constructor(private route: ActivatedRoute) {}
// Get the parameters in the component
getParams() {
const params = this.route.snapshot.params;
console.log(params);
}
By using the params
method of the ActivatedRoute
, you can easily get the parameters from the URL. Just keep in mind that this method will return all the parameters in the URL, including the ones from the parent route.
Share Your Experience
Now that you have the solution to your query parameter woes, why not share your experience with other developers? Have you faced any other roadblocks in Angular 2 and found ingenious solutions? Leave a comment below and let's help each other grow as developers! 💪
Happy coding! 🚀