How to get a variable type in Typescript?
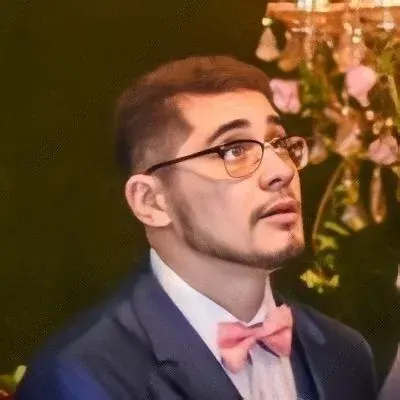
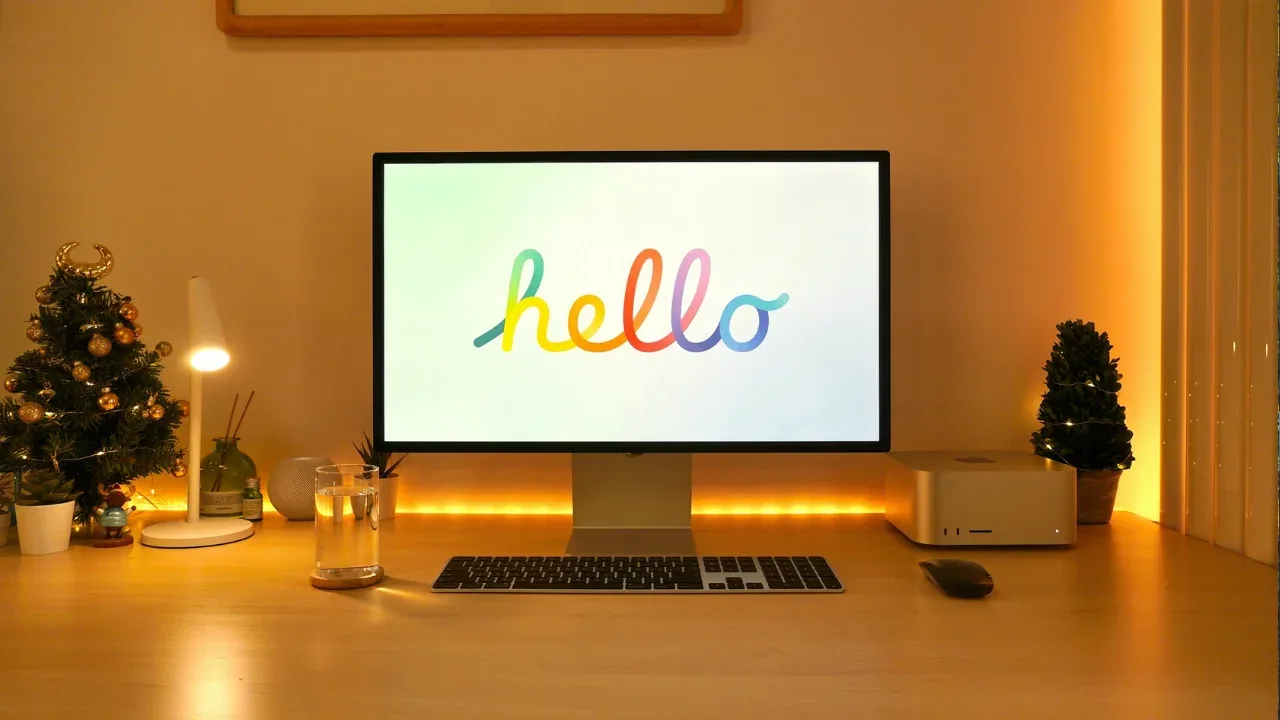
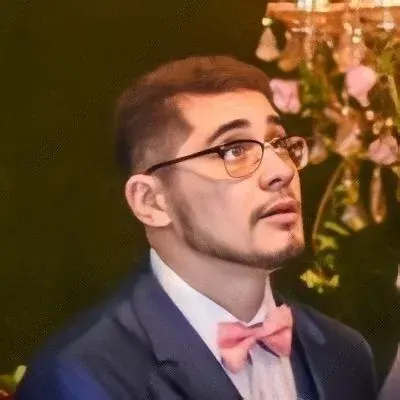
How to Get a Variable Type in TypeScript? 💪📝
So, you have a variable, and you want to check its type in TypeScript? Don't worry, you're not alone! This is a common question among TypeScript developers. In this blog post, we'll dive into ways to check the type of a variable and provide you with easy solutions to this problem. Let's get started! 🚀
The Scenario 📖
Imagine you have the following variable:
abc: number | string;
Now you want to perform a specific action based on the type of abc
. You might be thinking that you can simply check the type
property of abc
, like this:
if (abc.type === "number") {
// do something
}
Unfortunately, that won't work, as JavaScript and TypeScript do not provide a direct type
property on variables. However, fear not! TypeScript has several built-in features that allow you to determine the type of a variable in a more reliable and elegant way. Let's explore them together! 😊
Solution 1: typeof
Operator 🌟
One way to check the type of a variable is by using the typeof
operator. This operator returns a string representing the type of the variable. Here's how you can use it:
if (typeof abc === "number") {
// do something
} else if (typeof abc === "string") {
// do something else
}
By using the typeof
operator, you can compare the type of abc
with the desired types and perform actions accordingly. Easy as pie! 🍰
Solution 2: instanceof
Operator 🌟
Another way to check the type of a variable is by using the instanceof
operator. This operator checks if the variable is an instance of a specific class or constructor function. Here's an example:
if (abc instanceof Number) {
// do something
} else if (abc instanceof String) {
// do something else
}
In this case, we are checking if abc
is an instance of the Number
or String
constructor functions. Keep in mind that this method only works for checking if the variable is an instance of a class, not for primitive types like number
or string
. 🤷♂️
Solution 3: Type Guards 🌟
Type guards are a powerful feature of TypeScript that allow you to narrow down the type of a variable within a code block. There are several ways to create type guards, such as using typeof
, instanceof
, or even custom type predicates. Here's an example of using a type guard with typeof
:
function isNumber(value: any): value is number {
return typeof value === "number";
}
function isString(value: any): value is string {
return typeof value === "string";
}
// Usage
if (isNumber(abc)) {
// do something
} else if (isString(abc)) {
// do something else
}
By creating these type guard functions, you can explicitly check the type of abc
within the code block, enhancing both type safety and readability. 🔒🔐
Your Turn! 🙌
Now that you know how to check the type of a variable in TypeScript, it's time to apply this knowledge to your projects. Whether you choose to use the typeof
operator, the instanceof
operator, or type guards, always opt for the approach that best fits your specific use case. 💡💡
Remember, TypeScript provides powerful type checking capabilities that can help you catch bugs early and write more robust code. Embrace the type system, experiment with different techniques, and level up your TypeScript game! 💪🔥
Have any questions or other ingenious ways to check variable types? Let us know in the comments below! Let's create a dialogue and enhance our developer community. 🗣️🤝
Don't forget to share this post with your fellow TypeScript enthusiasts and spread the knowledge! 👩🏻💻👨🏻💻
Happy coding! ✨💻