How do I prevent the error "Index signature of object type implicitly has an "any" type" when compiling typescript with noImplicitAny flag enabled?
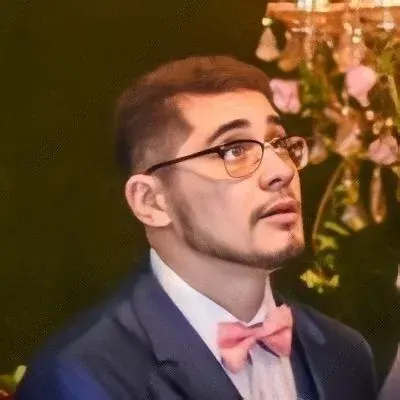
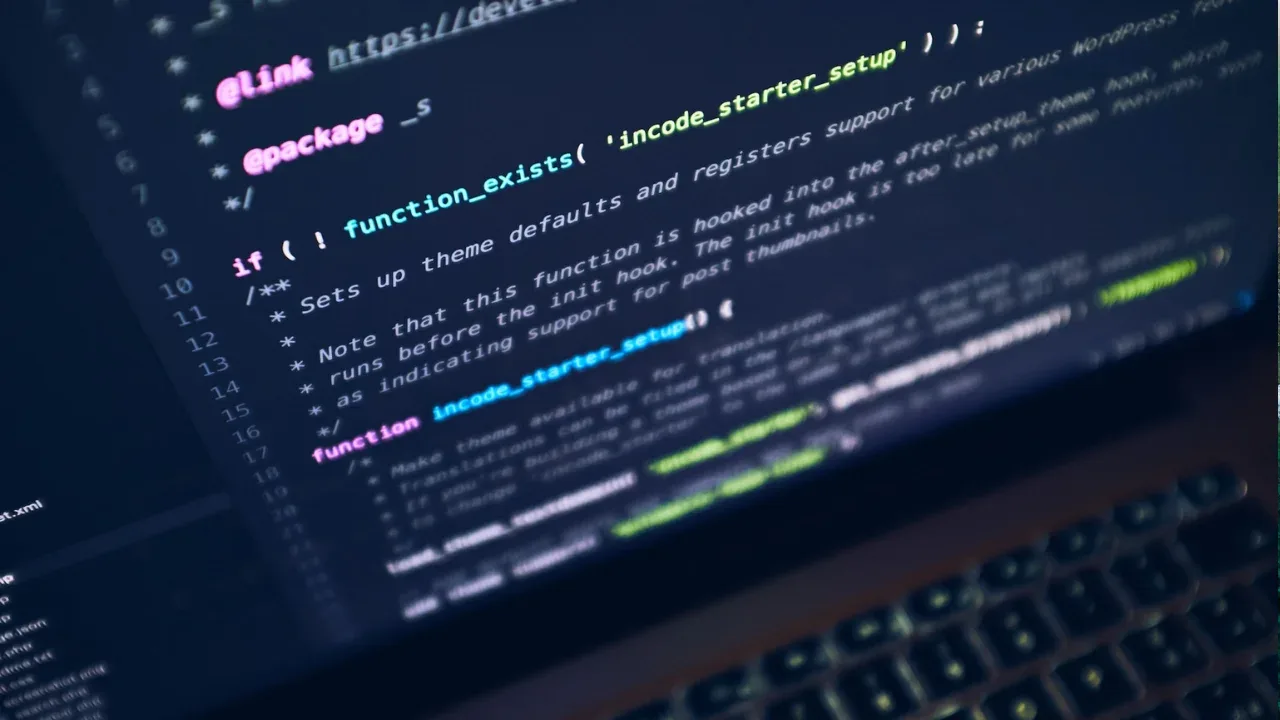
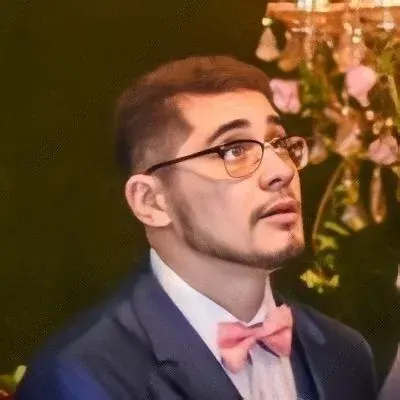
How to Prevent the "Index signature of object type implicitly has an 'any' type" Error in TypeScript with noImplicitAny Flag Enabled 😕
So you're trying to compile your TypeScript code with the --noImplicitAny
flag enabled, but you keep getting this pesky error:
Index signature of object type implicitly has an 'any' type
Don't worry, you're not alone! This error typically occurs when you have an object with an index signature but haven't explicitly assigned a type to it. Luckily, there are a few easy solutions to help you overcome this error. Let's dive in! 💪
Understanding the Problem
In your case, you have an object someObject
with properties firstKey
, secondKey
, and thirdKey
. You also have a variable key
that can hold any of the possible keys in someObject
. You're trying to access the value associated with key
, but TypeScript is throwing the error.
Solution 1: Type Assertion
One possible solution is to use a type assertion to explicitly tell TypeScript the type of the value you expect. Here's an example:
let secondValue: string = <string>someObject[key];
By using the type assertion <string>
, you're telling TypeScript that someObject[key]
should be of type string
. This can help resolve the error and make TypeScript happy.
Solution 2: Use an Index Signature
Another option is to define an index signature on your interface ISomeObject
. This allows TypeScript to infer the type of the index and prevent the error. Here's how you can modify your interface:
interface ISomeObject {
[key: string]: string;
firstKey: string;
secondKey: string;
thirdKey: string;
}
Now, TypeScript knows that any key used to access someObject
should return a value of type string
. This aligns with your intention of having the key dynamically assigned from elsewhere in your application.
Solution 3: Type Assertion with as keyword
If you prefer a more modern syntax, you can also use the as
keyword for type assertions:
let secondValue: string = someObject[key] as string;
This achieves the same result as the first solution, but with syntactic sugar.
Is it possible with --noImplicitAny
?
Yes, it's definitely possible to use the --noImplicitAny
flag while addressing this error. By applying one of the solutions mentioned above, you can ensure that every type is explicitly defined, preventing any implicit any
types.
Conclusion and Reader Engagement 🎉
Congratulations! You now have a few easy solutions to prevent the "Index signature of object type implicitly has an 'any' type" error when compiling TypeScript with the --noImplicitAny
flag enabled. Remember to apply either a type assertion or an index signature to make TypeScript happy.
Try out these solutions in your code and let me know in the comments which one worked best for you! 👇
If you found this blog post helpful, please consider sharing it with your fellow TypeScript developers. Happy coding! 💻✨