Expression ___ has changed after it was checked
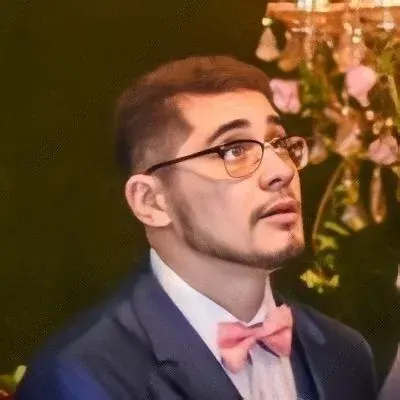
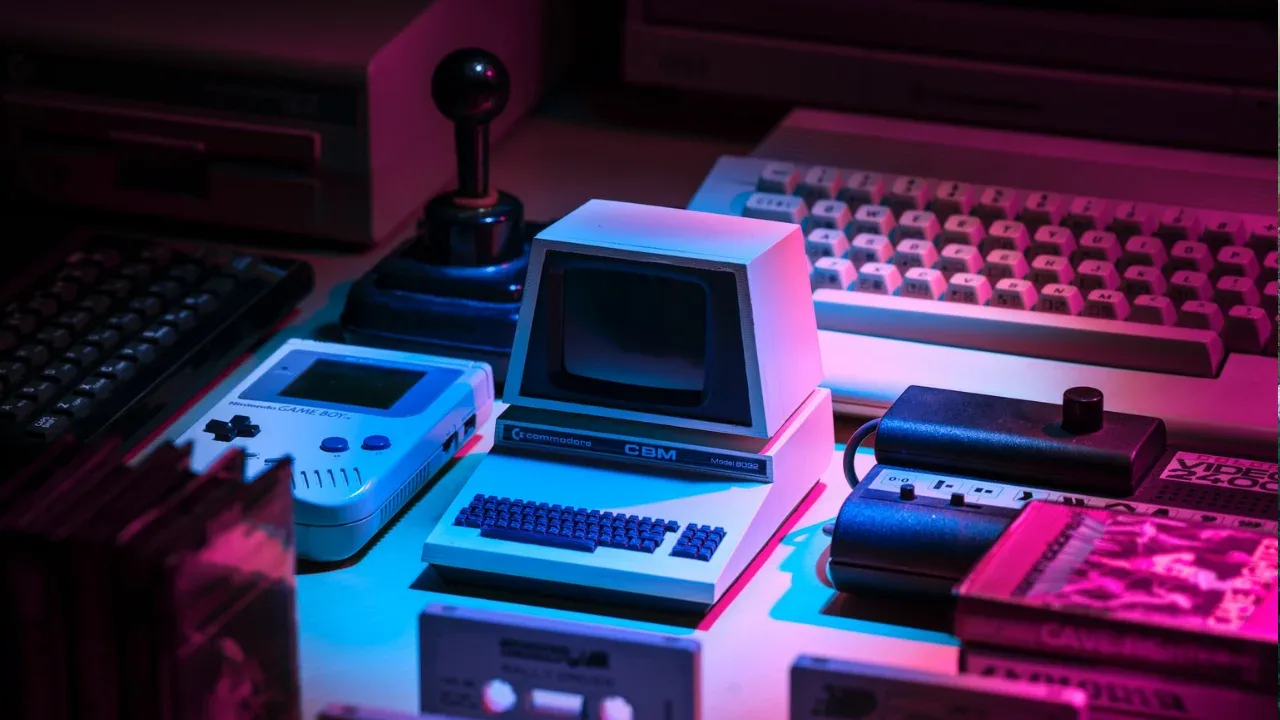
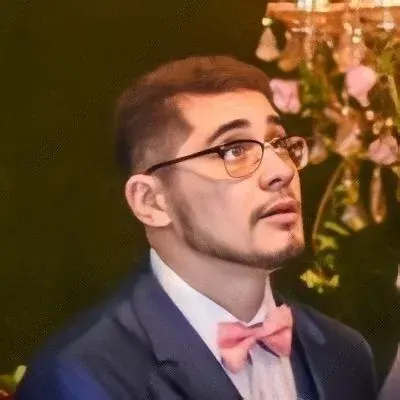
Understanding the Expression ___ has changed after it was checked Error in Angular
You're ready to launch your Angular app, but suddenly you encounter an error that says: "Expression '___' has changed after it was checked". 😱 Don't worry, you're not alone in facing this issue! This error is quite common in Angular development and can be easily resolved. In this blog post, we will dive deep into this error, understand its causes, and provide easy-to-follow solutions. So, let's get started! 🚀
What Causes the "Expression ___ has changed after it was checked" Error?
To understand this error, let's take a look at the code snippet you provided:
@Component({
selector: 'my-app',
template: `<div>I'm {{message}}</div>`,
})
export class App {
message: string = 'loading :(';
ngAfterViewInit() {
this.updateMessage();
}
updateMessage() {
this.message = 'all done loading :)'
}
}
In this code, you have a component named App
with a template that includes the expression I'm {{message}}
. The ngAfterViewInit
lifecycle hook is used to update the value of message
to 'all done loading :)'
. However, this is where the problem arises.
In Angular, change detection is triggered before and after the lifecycle hooks, including ngAfterViewInit
. During the change detection process, Angular checks for any changes in the component's properties and updates the view accordingly. However, if any changes are detected after the view has been checked, Angular throws the "Expression has changed after it was checked" error.
Solution 1: Use the setTimeout() Hack
One way to solve this error is by using the setTimeout()
function. By wrapping the code inside ngAfterViewInit
in a setTimeout()
, we can delay the update and ensure it happens after the view has been checked. 😎
To implement this solution, modify your code as follows:
ngAfterViewInit() {
setTimeout(() => {
this.updateMessage();
});
}
By using this workaround, you are essentially queueing the updateMessage()
method to run in the next JavaScript event loop. This way, it will run after the view has been checked, preventing the error from occurring.
Solution 2: Use the ChangeDetectorRef Service
Another solution involves using the ChangeDetectorRef
service provided by Angular. 💡 This service allows you to manually trigger change detection within your component.
First, import ChangeDetectorRef
in your component:
import { Component, ChangeDetectorRef } from '@angular/core';
Next, inject ChangeDetectorRef
into your component's constructor:
constructor(private cdr: ChangeDetectorRef) {}
Now, you can call the detectChanges()
method of the ChangeDetectorRef
service after updating the message
property:
ngAfterViewInit() {
this.updateMessage();
this.cdr.detectChanges();
}
By calling detectChanges()
, you explicitly tell Angular to run change detection on your component, bypassing the automatic check that triggered the error. This ensures that the view is updated correctly.
Time to Fix the Error and Launch Your App!
Now that you have learned two easy solutions to resolve the "Expression has changed after it was checked" error in Angular, it's time to implement them in your code and launch your app without any hiccups! 🎉
If you're facing this error, give one of these solutions a try and let us know how it goes. Also, if you have any other Angular-related questions or need further assistance, drop a comment below. We would love to help you out! 👍
Keep coding! 💻
🎉 Call-to-Action: Join the Conversation!
Have you ever encountered the "Expression has changed after it was checked" error in your Angular projects? How did you solve it? Comment below and let's help each other out. Don't forget to share this blog post with your Angular buddies to save them from the same headache! 😉✨