Enforcing the type of the indexed members of a Typescript object?
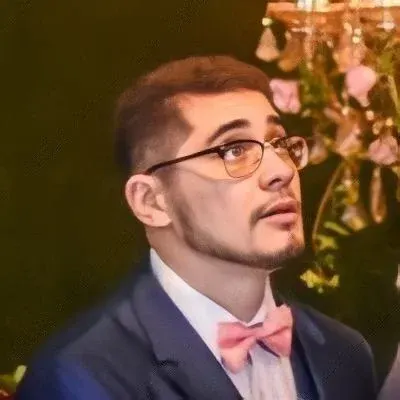
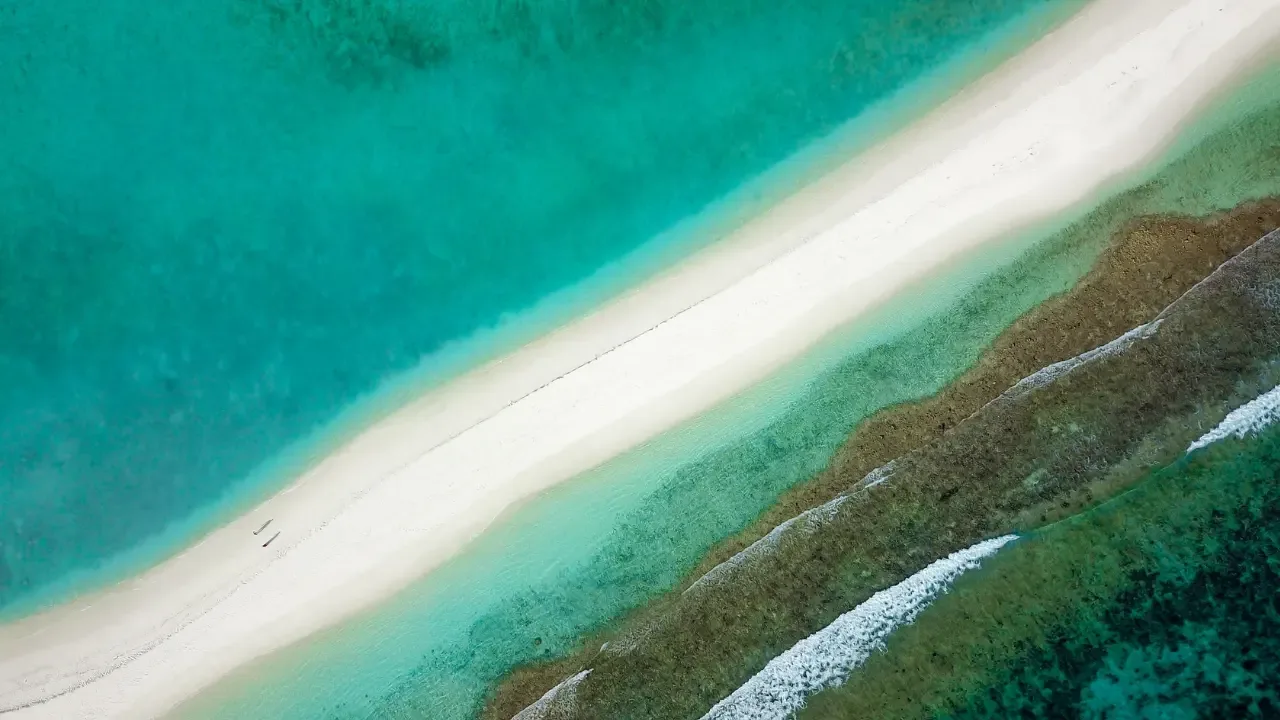
Enforcing the Type of Indexed Members in a TypeScript Object
Are you tired of debugging errors caused by assigning the wrong data type to the members of your TypeScript object? 😫 Don't worry, we've got you covered! In this blog post, we'll explore how to enforce the type of indexed members in a TypeScript object, ensuring that all values adhere to a specific data type. Let's dive in! 🚀
The Problem
Imagine you want to store a mapping of strings. In TypeScript, you can achieve this by defining an object and assigning values to its indexed members, like so:
var stuff = {};
stuff["a"] = "foo"; // okay
stuff["b"] = "bar"; // okay
stuff["c"] = false; // ERROR! bool != string
As you can see, TypeScript doesn't prevent you from assigning a boolean value (false
) to the indexed member "c," even though we want all values to be strings. This can lead to runtime errors and unexpected behavior, making our code difficult to maintain. 😓
The Solution
Fortunately, there's a way to enforce the type of indexed members in our TypeScript object. We can achieve this by leveraging the power of TypeScript's index signatures. 🎉 Let's see how it's done:
First, we need to define an interface that represents our desired object structure. In this case, we want all the values to be of type string. Here's how we can define our interface:
interface StringMap {
[key: string]: string;
}
Next, we can create an object that adheres to our
StringMap
interface and assign values accordingly:
var stuff: StringMap = {};
stuff["a"] = "foo"; // okay
stuff["b"] = "bar"; // okay
stuff["c"] = false; // ERROR! bool != string
By specifying the StringMap
type when declaring our stuff
object, TypeScript will now enforce that all indexed members must have string values. 🙌
When to Use This Approach
Enforcing the type of indexed members using TypeScript's index signatures is particularly useful in scenarios where you need stricter data type validation. It provides an additional layer of type safety, preventing potential bugs caused by assigning incorrect data types. 🐛🚫
Call to Action
Now that you know how to enforce the type of indexed members in a TypeScript object, why not give it a try in your next TypeScript project? Share your thoughts, experiences, or any other TypeScript tips and tricks in the comments below. We'd love to hear from you! 😄💬
Remember, TypeScript is all about making our lives as developers easier and more enjoyable. Let's embrace its powerful features and write robust, bug-free code together. Happy typing! ✍️💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
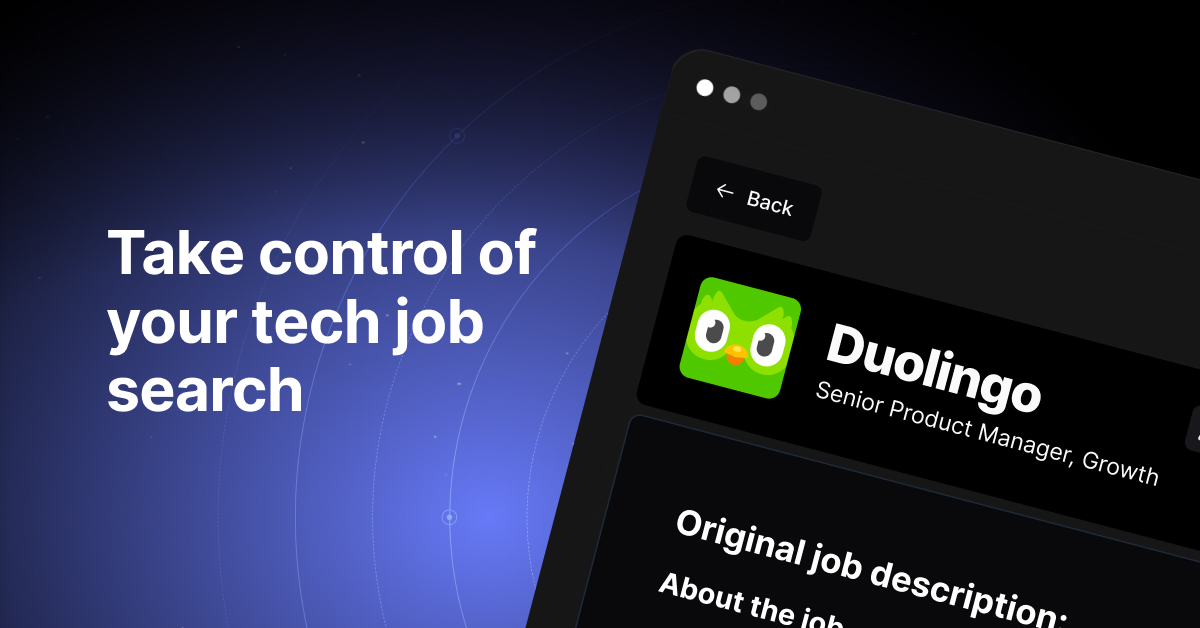