Does Typescript support the ?. operator? (And, what"s it called?)
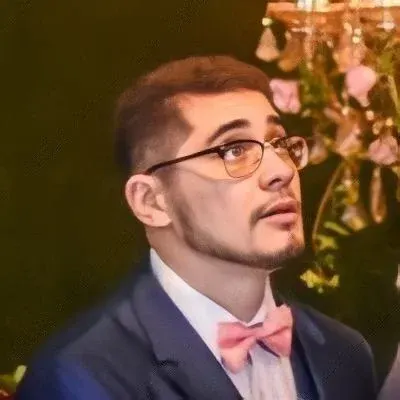
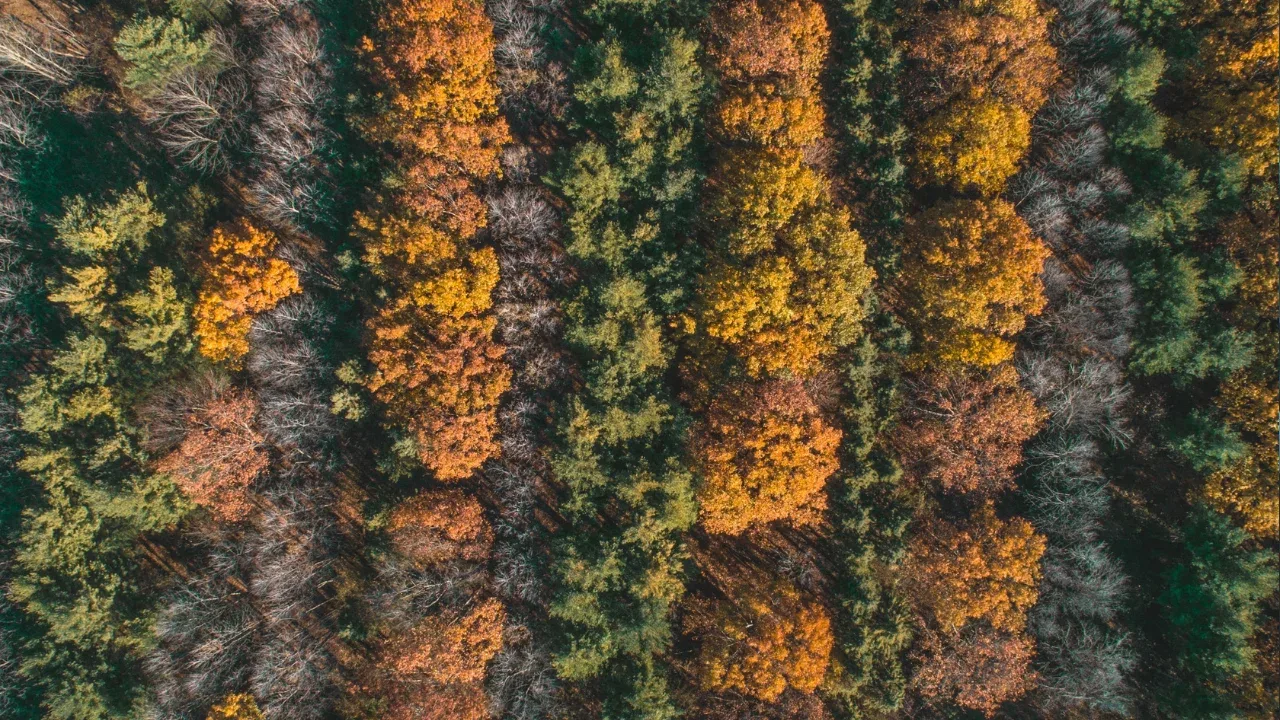
Does Typescript support the ?.
operator? (And, what's it called?)
You know those pesky JavaScript errors that pop up when you try to access properties or methods on a null or undefined value? 😤 They can drive any developer insane! But fear not, because TypeScript has the perfect solution for this issue – the ?.
operator, also known as the optional chaining operator. 🎉
What is the ?.
operator?
The ?.
operator allows you to safely access nested properties or methods without worrying about encountering a TypeError
if any intermediate value along the chain happens to be null or undefined. It's like a protective shield for your code! 🛡️
Let's take a look at an example:
interface Foo {
bar: {
baz: number;
} | null;
}
const foo: Foo = {
bar: {
baz: 42
}
};
// Without optional chaining
const baz = foo.bar.baz; // Works fine
foo.bar = null;
// Without optional chaining
const baz = foo.bar.baz; // Throws a TypeError 🚨
In the above example, we have an interface called Foo
, which contains a bar
property that can either be an object with a baz
property or null. If the bar
property is null, attempting to access baz
directly without the ?.
operator will result in a TypeError
. 😱
How does the ?.
operator work?
Using the ?.
operator is simple. Instead of directly accessing nested properties or methods, you can chain them together and append ?.
after each dot.
const baz = foo?.bar?.baz;
In the example above, foo?.bar?.baz
will return the value of baz
if all the intermediate values (foo
, bar
, and baz
) are not null or undefined. If any of them are null or undefined, the expression will short-circuit and evaluate to undefined
without throwing an error. Isn't that neat? 😎
Is there a more common name for the ?.
operator?
You might be wondering if there's a more commonly used name for the ?.
operator. Well, you're not alone! It's indeed quite challenging to find information about it by searching for the operator itself. 🤔
Fear not! We got you covered. The ?.
operator is most commonly referred to as the safe navigation operator. 🧭 This name accurately communicates its purpose of safely navigating through nested properties or methods.
Wrapping up
With the ?.
operator (a.k.a. the safe navigation operator) in TypeScript, you can finally bid farewell to those annoying TypeError
issues caused by accessing properties or methods on null or undefined values. 🙌
So go ahead and start implementing the ?.
operator in your TypeScript projects to make your code much more robust and error-free! 💪 And if you encounter any issues or have any questions along the way, feel free to comment below. Let's help each other out! 🤝
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
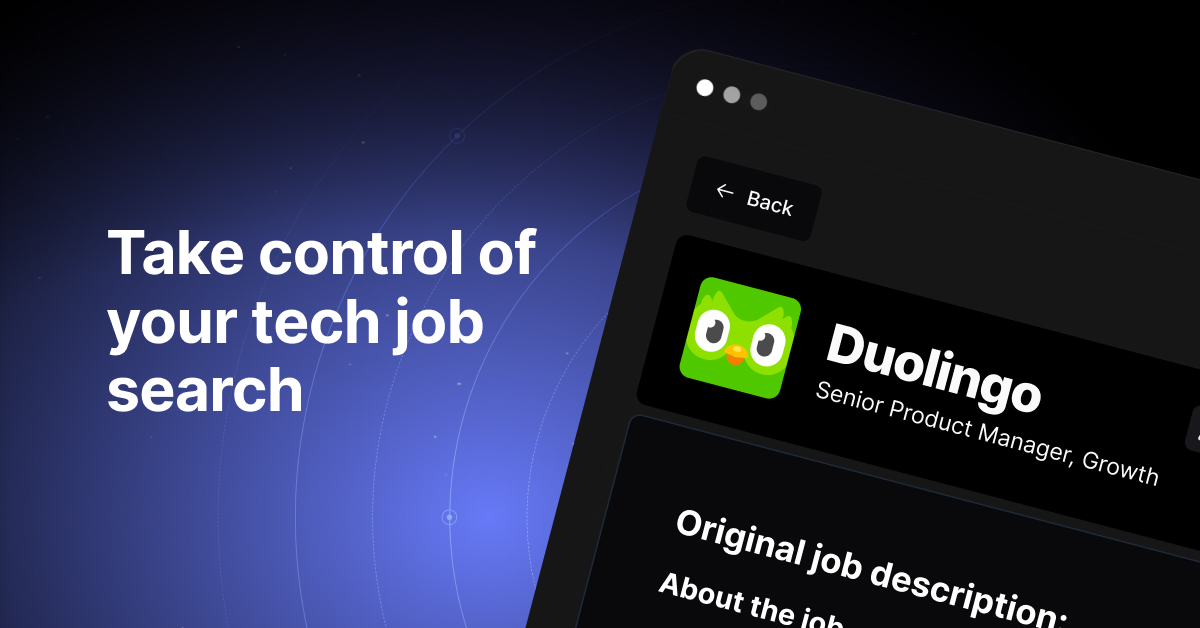