Declaring abstract method in TypeScript
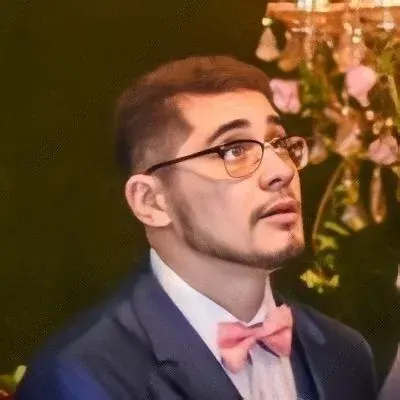
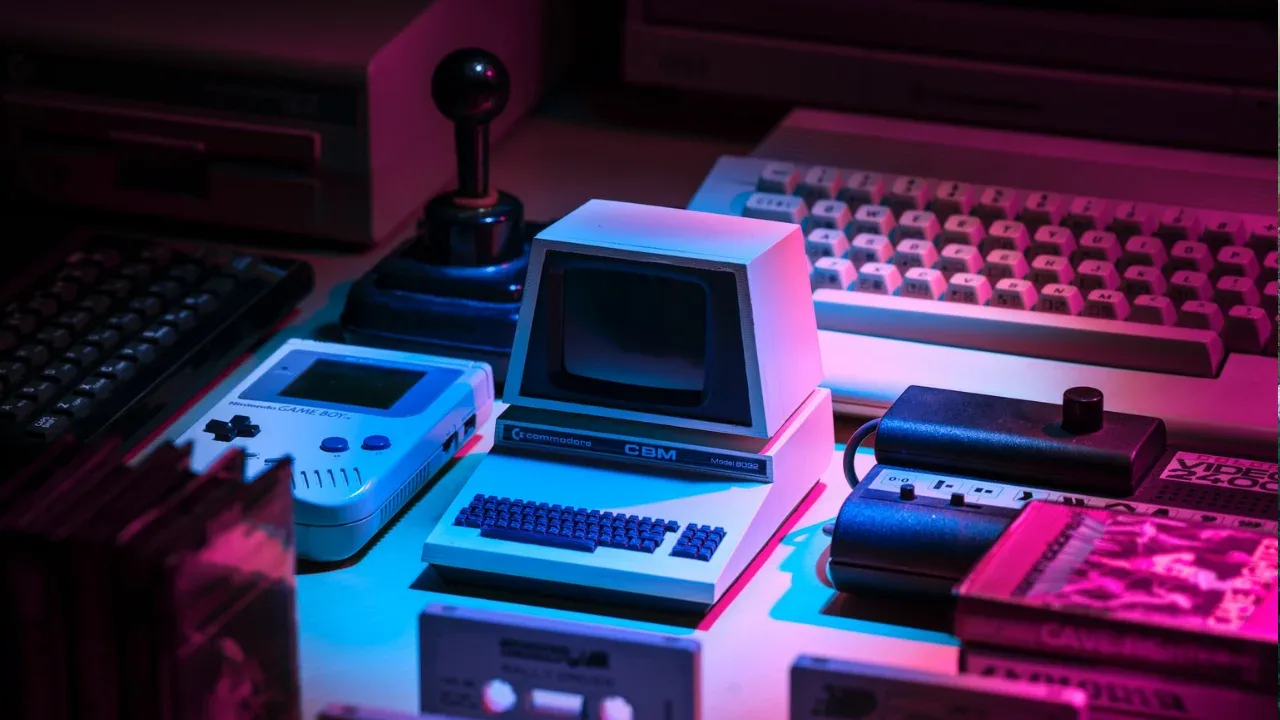
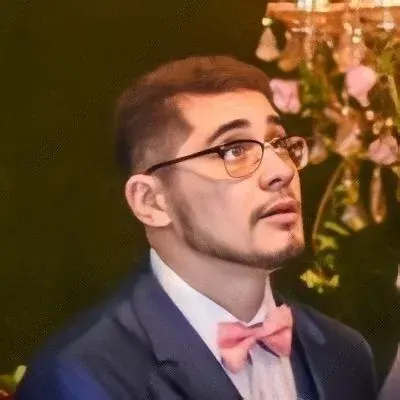
Declaring Abstract Methods in TypeScript: A Complete Guide 🚀
Are you struggling to correctly define abstract methods in TypeScript? 😓 Don't worry, you're not alone! In this blog post, we will explore common issues and provide easy solutions to help you declare abstract methods like a pro. So let's dive in and enhance your TypeScript knowledge! 🎉
Understanding Abstract Methods
Abstract methods are methods that must be implemented by any class that inherits from the abstract class. They provide a blueprint for child classes, ensuring they define specific functionalities required by the parent class. In TypeScript, abstract methods are declared using the abstract
keyword. 🔄
Declaring Abstract Methods
To declare an abstract method, follow these three simple steps:
Start by creating an abstract class using the
abstract
keyword. This class will serve as the blueprint for child classes. For example:abstract class Animal { constructor(public name: string) {} abstract makeSound(input: string): string; move(meters: number) { alert(this.name + " moved " + meters + "m."); } }
Inside the abstract class, declare the desired abstract method(s) using the
abstract
keyword without providing an implementation.abstract makeSound(input: string): string;
It's important to note that the abstract method(s) cannot have a body. They only declare the method's signature.
Once the abstract class is set up, create a child class that extends the abstract class and provides an implementation for the abstract method(s).
class Snake extends Animal { constructor(name: string) { super(name); } makeSound(input: string): string { return "sssss" + input; } move() { alert("Slithering..."); super.move(5); } }
By following these steps, you can correctly declare abstract methods in TypeScript. 🎉
Defining Protected Methods
Now let's address your concern about defining protected
methods correctly. When it comes to protecting methods in TypeScript, the protected
keyword does play a crucial role.
To define a protected method, follow these steps:
Inside the abstract or concrete class, declare the desired protected method(s) using the
protected
keyword.protected myProtectedMethod() { // code goes here }
The
protected
keyword ensures that the method is only accessible within the class and its subclasses. It provides a level of visibility that restricts access from outside the class hierarchy.class Animal { protected move(meters: number) { // code goes here } }
With these steps, you can correctly define and access protected
methods in TypeScript. 😄
Engage with the Community!
Congratulations! 🎉 You now possess the knowledge to declare abstract methods and define protected methods in TypeScript. If you found this blog post helpful, make sure to share it with your fellow developers and encourage them to overcome these challenges as well. Together, we can create a stronger TypeScript community! 💪
Do you have any other TypeScript questions or topics you'd like us to cover? Let us know in the comments below. We love hearing from our readers! 😊