Constructor overload in TypeScript
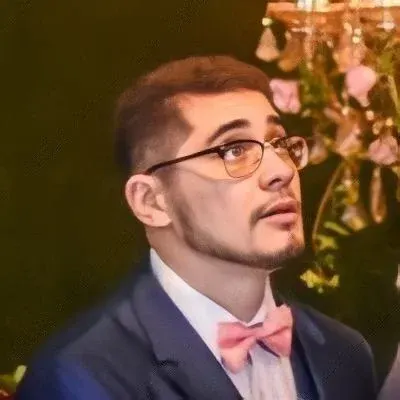
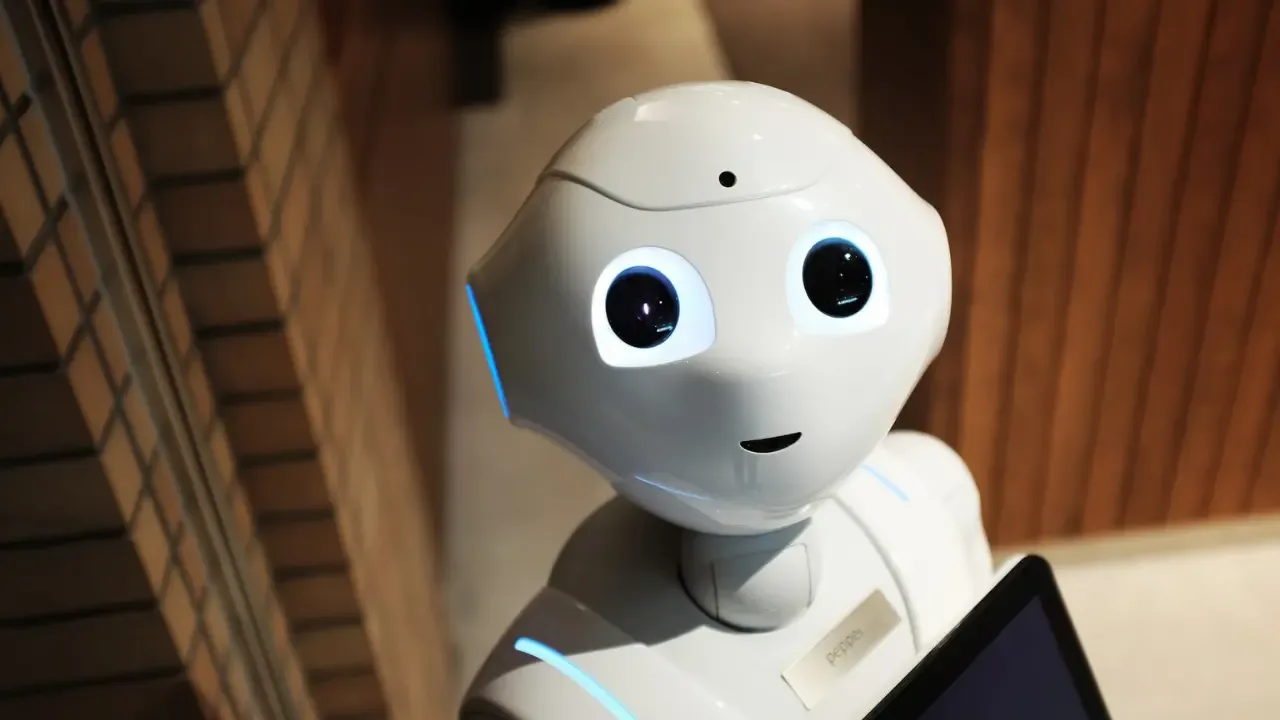
📝 Title: Constructor Overload in TypeScript: A Simple Guide
Hey there, fellow TypeScript enthusiasts! 👋 Are you running into trouble with constructor overloading? 🤔 Don't fret! In this blog post, we'll demystify this concept with clear explanations and provide easy solutions to common issues. So, let's get started and level up our TypeScript skills! 💪
Understanding the Problem
The context for this question revolves around constructor overloading in TypeScript. The language specification refers to constructor overloads on page 64, but there's no sample code provided. 😕 Our friend here attempted a basic class declaration with constructor overloads, but encountered an error when running the TypeScript compiler.
interface IBox {
x: number;
y: number;
height: number;
width: number;
}
class Box {
public x: number;
public y: number;
public height: number;
public width: number;
constructor(obj: IBox) {
this.x = obj.x;
this.y = obj.y;
this.height = obj.height;
this.width = obj.width;
}
constructor() {
this.x = 0;
this.y = 0;
this.width = 0;
this.height = 0;
}
}
When running tsc BoxSample.ts
, an error is thrown due to the duplicate constructor definition. But worry not, we're here to help! 🚀
Solution: Method Overloading in TypeScript Constructors
TypeScript allows method overloading, but it's crucial to note that constructor overloading doesn't work in the same way. Unlike methods, constructors can't be overloaded purely based on parameters. TypeScript only supports a single constructor implementation.
However, there's a neat workaround! 😎 We can achieve similar functionality using optional or default parameter values. Let's modify our code accordingly:
class Box {
public x: number;
public y: number;
public height: number;
public width: number;
constructor(obj?: IBox) { // Use optional parameter
if (obj) {
this.x = obj.x;
this.y = obj.y;
this.height = obj.height;
this.width = obj.width;
} else {
this.x = 0;
this.y = 0;
this.width = 0;
this.height = 0;
}
}
}
By marking the obj
parameter as optional using the ?
symbol, we create a single constructor that can handle both scenarios: when an IBox
object is provided and when no arguments are passed. 🎉
Conclusion and Call-to-Action
Constructor overloading may not be available in TypeScript, but we can achieve similar functionality with optional parameters. Using the example provided, we successfully addressed the common issue of duplicate constructor definitions and offered a straightforward solution.
Now it's your turn! ✨ Experiment with constructor overloads and optional parameters in TypeScript. If you encounter any issues or have questions, feel free to share them in the comments below. Let's learn and grow together as a TypeScript community! 🌟✨
Happy coding! 💻🔥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
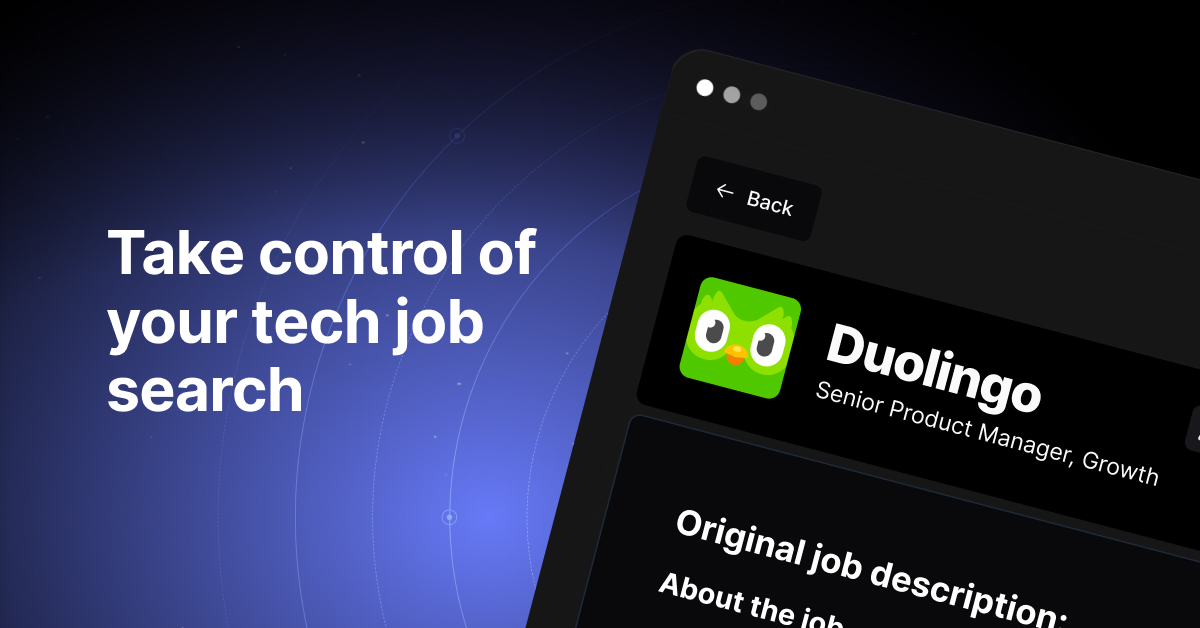