Angular 2 @ViewChild annotation returns undefined
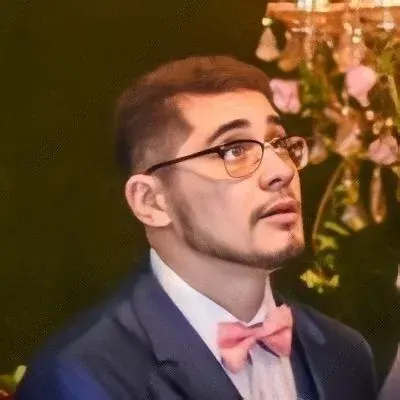
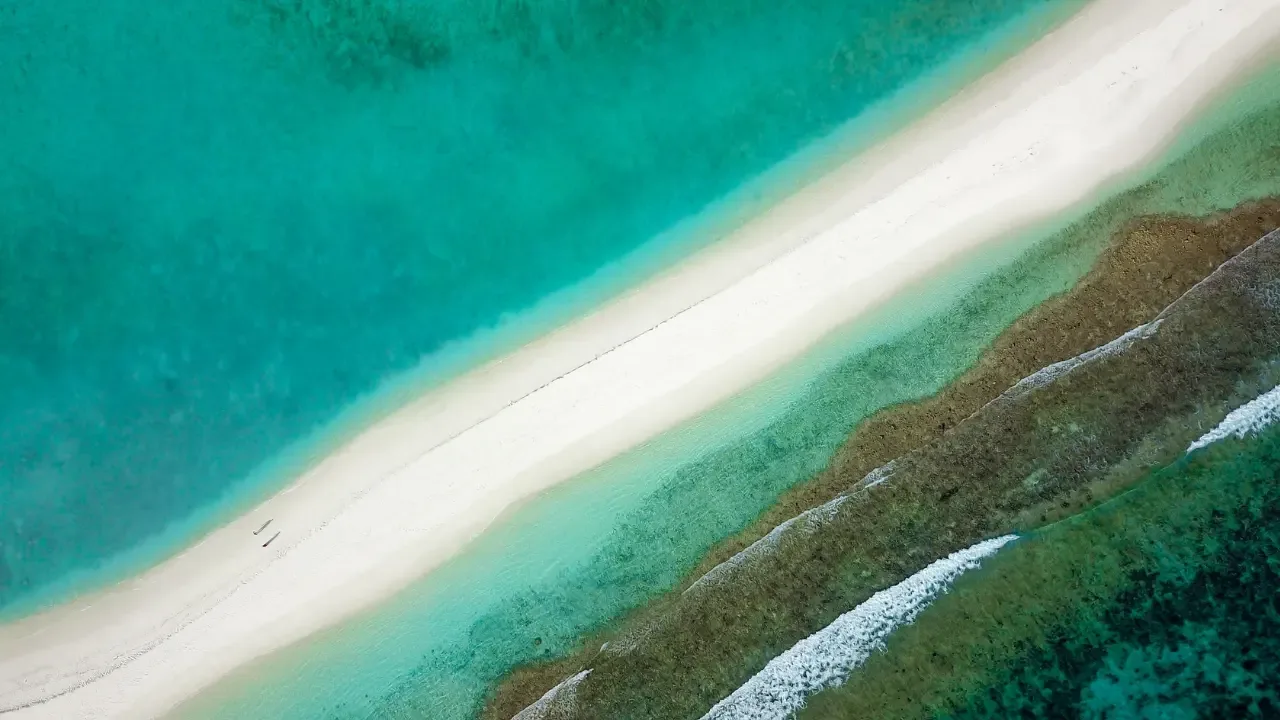
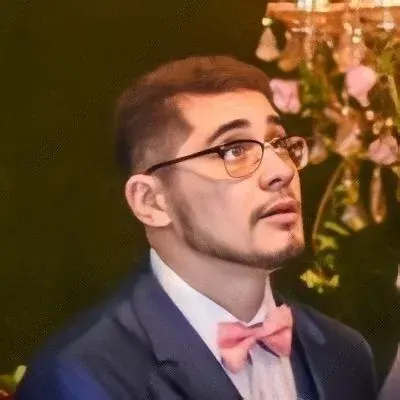
Angular 2 @ViewChild annotation returns undefined
Are you learning Angular 2 and trying to access a child component from a parent component using the @ViewChild Annotation? 🤔 It can be frustrating when the @ViewChild annotation returns undefined and you encounter issues with accessing properties or methods of the child component. But don't worry, we've got you covered! Here's a step-by-step guide to help you resolve this problem. 💪🏼
The Problem
As you mentioned, you're trying to access the FilterTiles
component from the BodyContent
component using the @ViewChild annotation. However, when you log this.ft
(which is the reference to the FilterTiles
component), it returns undefined. This leads to an exception when you try to push something inside the tiles
array.
The Solution
The reason why the @ViewChild annotation returns undefined in this case is because the FilterTiles
component is not yet rendered or initialized at the time you're trying to access it.
To handle this issue, you can use the Angular 2 lifecycle hook called ngAfterViewInit
. This hook is called after the view and child components are initialized, ensuring that you can safely access the child component.
Here's how you can modify your code to make it work:
import { ViewChild, Component, AfterViewInit } from 'angular2/core';
import { FilterTiles } from '../Components/FilterTiles/FilterTiles';
@Component({
selector: 'ico-body-content',
templateUrl: 'App/Pages/Filters/BodyContent/BodyContent.html',
directives: [FilterTiles]
})
export class BodyContent implements AfterViewInit {
@ViewChild(FilterTiles) ft: FilterTiles;
ngAfterViewInit() {
console.log(this.ft);
// Now you can safely access and modify the child component
var startingFilter = {
title: 'cognomi',
values: ['griffin', 'simpson']
};
this.ft.tiles.push(startingFilter);
}
public onClickSidebar(clickedElement: string) {
// You can also access the child component here, but make sure it's already initialized
}
}
By implementing the ngAfterViewInit
hook and moving your code that accesses the child component inside it, you ensure that the FilterTiles
component is properly initialized before you try to access it.
Now, when you run the code, you should see the expected behavior without any errors. 😄
Conclusion
In this guide, we addressed the common issue of the @ViewChild annotation returning undefined in Angular 2. We provided a step-by-step solution by utilizing the ngAfterViewInit
lifecycle hook to ensure that the child component is initialized before accessing it.
Go ahead and give it a try! If you have any further questions or suggestions, feel free to leave a comment below. Happy coding! 💻🚀