Defining TypeScript callback type
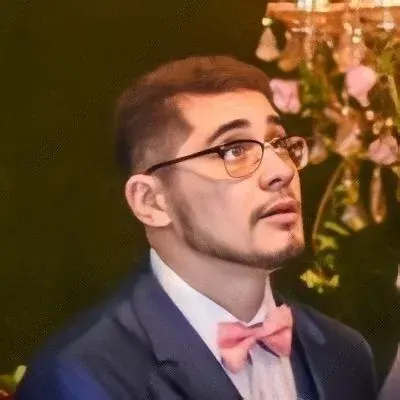
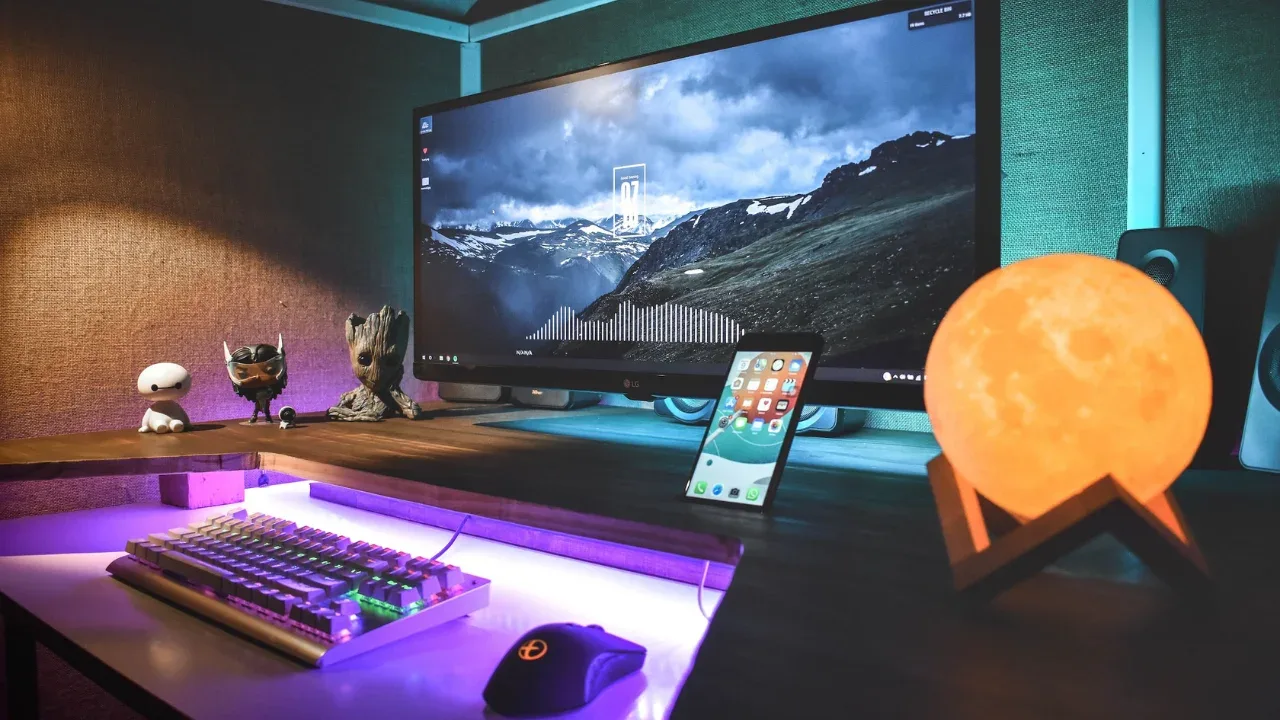
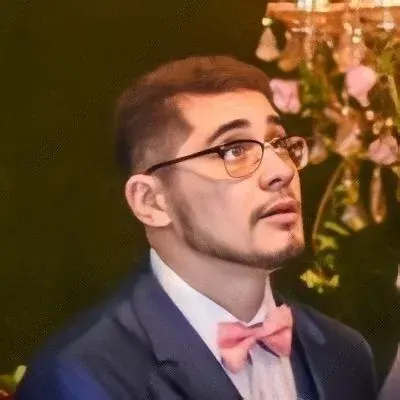
Defining TypeScript Callback Type: The Ultimate Guide
š Hey there! Are you struggling to define the type of a callback in TypeScript? Don't worry, you're not alone. This can be quite a confusing topic for many developers. But fear not, my friend! In this blog post, we'll dive deep into the world of TypeScript callback types and help you find easy solutions to define them. Let's get started! š
Understanding the Problem
So, you have a class in TypeScript with a public field called myCallback
, and you want to define its type. Currently, it's set as any
, but you're wondering if there's a way to specify the method signature of the callback.
In your attempts, you tried defining the callback type as public myCallback: ();
or public myCallback: function;
, but unfortunately, you ran into compile-time errors. š«
TypeScript Callback Type Solution
The good news is that TypeScript provides a couple of ways to define callback types. Let's explore them:
1. Using Function Type Syntax
To define the type of your callback, you can use the function type syntax. Here's how you can do it:
public myCallback: () => void;
In this example, () => void
represents a callback that takes no arguments and doesn't return a value. Replace void
with the appropriate return type if your callback should return something.
2. Using Function Interface
Alternatively, you can use a function interface to define your callback type. Here's an example:
interface MyCallbackType {
(): void;
}
You can then use this interface to define the type of your callback in your class:
public myCallback: MyCallbackType;
Feel free to add parameters and specify return types in your function interface as needed.
Practical Example
Let's put it all together with a practical example using your initial code snippet:
class CallbackTest {
public myCallback: () => void;
public doWork(): void {
// doing some work...
this.myCallback(); // calling callback
}
}
const test = new CallbackTest();
test.myCallback = () => alert("done");
test.doWork();
In this example, we created a CallbackTest
class with a doWork
method that calls the callback defined by myCallback
. We then assigned a callback function to myCallback
and triggered the doWork
method, which alerts "done" as expected.
Conclusion
Defining TypeScript callback types might seem tricky at first, but with the right syntax and approach, it becomes much simpler. By using either the function type syntax or function interfaces, you can easily define the signature of your callbacks.
So, go ahead and leverage TypeScript's powerful type system to make your code more robust and maintainable!
If you found this blog post helpful, don't forget to share it with your fellow developers. And if you have any questions or suggestions, we'd love to hear from you in the comments below.
Happy coding! šš