What is the purpose of willSet and didSet in Swift?
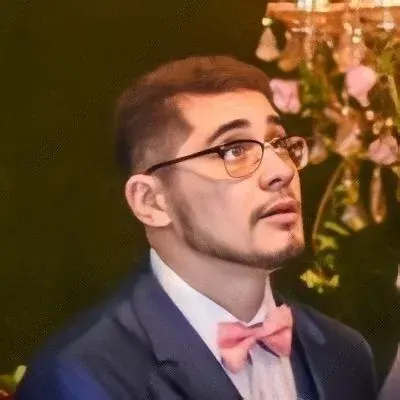
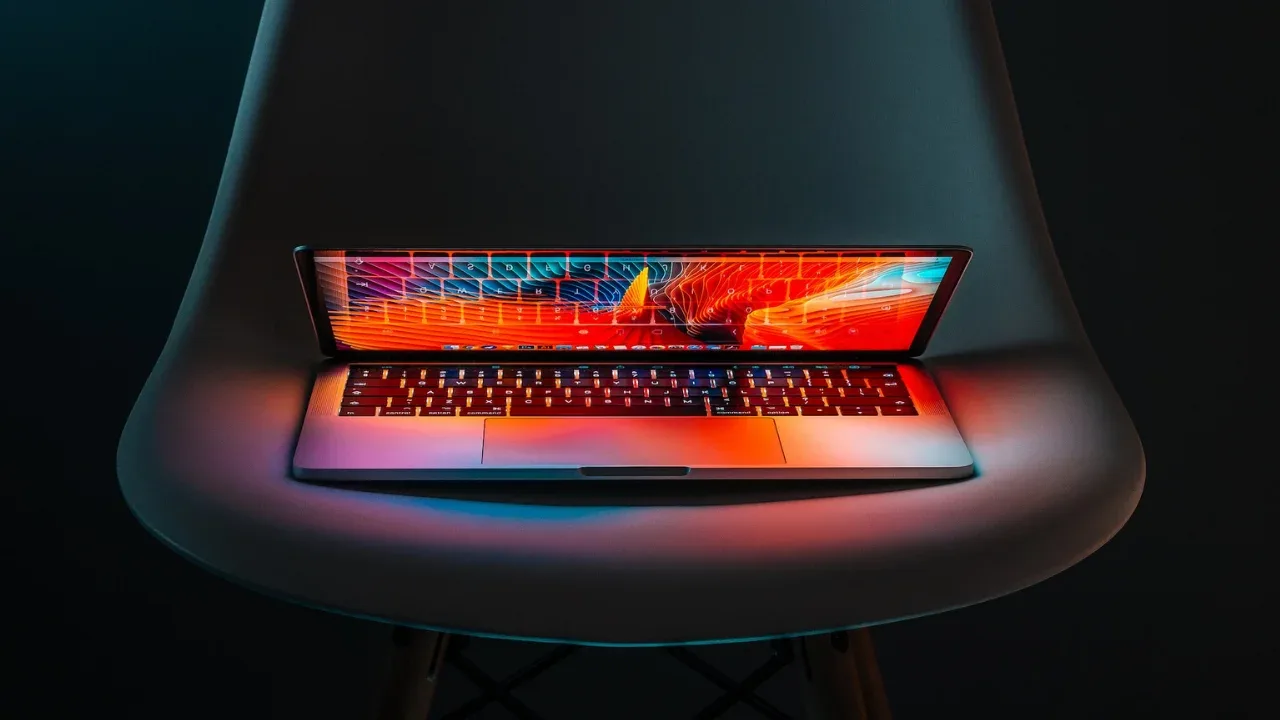
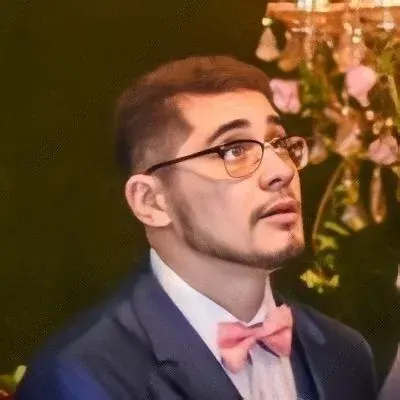
Understanding the Purpose of willSet and didSet in Swift
If you're a Swift developer, you might have come across the terms "willSet" and "didSet" while working with properties. 📝
At first glance, their purpose might seem confusing, especially considering that you could achieve the same functionality by simply placing the relevant code inside the setter. 🤔
In this article, we'll dive into the true essence of willSet and didSet, explore use cases, address common issues, and provide easy solutions. Let's get started! 🚀
The Essence of willSet and didSet
willSet and didSet are property observers in Swift that allow you to execute code before and after a property's value is set. They provide a mechanism to observe and respond to value changes.
willSet
The willSet property observer is called just before a new value is assigned to the property.
It receives a parameter with an implicit name newValue by default, which represents the new value that will be assigned to the property. You can give it a custom name if desired. 📥
Here's an example that demonstrates the willSet property observer in action:
var score: Int = 0 {
willSet(newScore) {
print("Current score: \(score). Next score: \(newScore).")
}
}
In this example, every time the score property is about to be updated, the willSet observer prints a message with the current score and the next score. 📢
didSet
The didSet property observer is called immediately after a new value is assigned to the property.
It receives a parameter with an implicit name oldValue by default, which represents the old value that was replaced. You can provide it with a custom name if desired. 📤
Let's see an example that demonstrates the didSet property observer:
var isLoggedIn: Bool = false {
didSet {
if isLoggedIn {
print("User has logged in!")
} else {
print("User has logged out!")
}
}
}
In this example, whenever the isLoggedIn property is assigned a new value, the didSet observer checks if the user has logged in or logged out, and prints a corresponding message. 🚪
Use Cases and Benefits
Now that we understand the purpose of willSet and didSet, let's explore some common use cases and their benefits:
Validation and Side Effects
Property observers are useful for performing validation on new values before they are set, ensuring that they meet certain requirements. This can include checking boundaries, avoiding invalid states, or preserving data integrity. 🛡️
Additionally, you can leverage these property observers to trigger side effects, such as updating UI elements, saving data to disk, or notifying other components about value changes.
Logging and Debugging
By using willSet and didSet property observers, you can easily log and track property changes during the runtime of your app. This can be instrumental in troubleshooting or understanding how values are being modified. 🐞
Common Issues and Solutions
While working with willSet and didSet, you might come across some common issues. Let's address a specific problem and provide an easy solution:
Problem: What if you want to access both the old and new values within didSet?
Solution: Swift provides an elegant solution by allowing you to access both the old and new values directly within the didSet property observer. You can use the oldValue and newValue properties to retrieve their respective values.
Here's an example that demonstrates accessing both values:
var age: Int = 30 {
didSet {
if oldValue != age {
print("Age changed from \(oldValue) to \(age).")
}
}
}
In this example, the didSet observer checks if the age property has changed and prints a message only if it has indeed changed.
Take Your Swift Skills to the Next Level! 🚀
Now that you've grasped the purpose of willSet and didSet, it's time to apply this knowledge in your own Swift projects. Start harnessing the power of property observers to create more robust, maintainable, and debuggable code! 💪
If you have any questions or insights to share, I'd love to hear from you. Let's continue the conversation in the comments section below! 👇
Happy coding! 🎉