What is the difference between `let` and `var` in Swift?
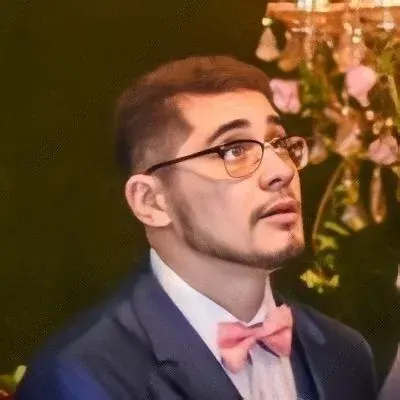
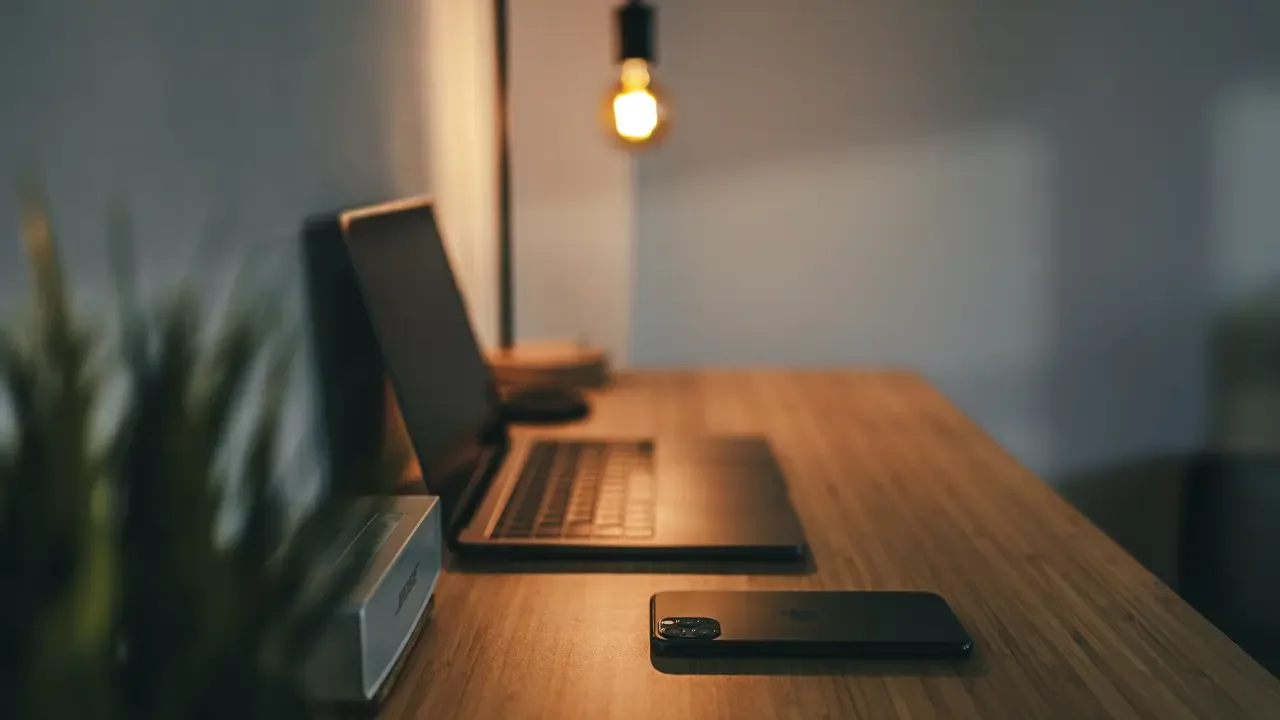
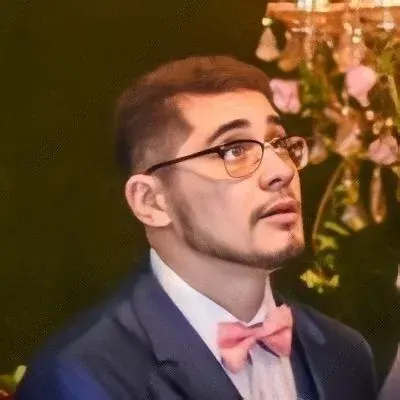
Understanding the Difference between let
and var
in Swift π€π
So, you're diving into the exciting world of Apple's Swift language, but you're running into a common question: "What is the difference between let
and var
in Swift?" π€·ββοΈ
Don't worry! Many beginners and even experienced developers have been puzzled by this too. π§
In a nutshell, the main difference between let
and var
in Swift boils down to mutability. Let me explain further! π
π let
- Immutable Constants
The keyword let
in Swift is used to define constants, which means that once you assign a value to a let
variable, you cannot change it later. It's like locking the value and throwing away the key. π
For example, let's say we have a let
variable called name
assigned to the value "John Doe":
let name = "John Doe"
Now, imagine trying to change the value of name
to "Jane Smith" later in your code:
name = "Jane Smith" //βοΈ ERROR! This will result in a compile-time error.
By using let
, Swift protects you from accidentally modifying a value that should remain unchanged. It ensures immutability and prevents unintended side effects in your code. π‘
π var
- Mutable Variables
On the other hand, using the keyword var
in Swift allows you to define mutable variables. These variables can have their values changed throughout your code. They're like keys that can open a door to new possibilities! πͺπ
Let's take a look at an example of using var
:
var age = 25
With this, we've defined a variable called age
and assigned it the value of 25. Now, if we want to update the age
later on, we can do so without any issues!
age = 30 // Perfectly fine!
Variables declared with var
are mutable, which can be handy when you expect a value to change over time. Just remember, though, that with great power comes great responsibility! ππ¦ΈββοΈ
π¨βπ» Compiler and Type Safety
Now, you might be wondering about the type safety aspect and how the compiler handles it. Swift is indeed a compiled language that checks types at compile-time, but there's a bit more to it. π§βπ¬
When you assign a value to a let
constant or var
variable, Swift infers the type based on the initial value you provide. This means that the compiler automatically figures out the type behind the scenes, so you don't have to worry about explicitly specifying it. π΅οΈββοΈπ
For example:
let pi = 3.14159 // Swift infers that 'pi' is of type Double
var score = 100 // Swift infers that 'score' is of type Int
If you try assigning a value of a different type to a let
constant, you'll get a clear error message from the compiler, like this:
let name: String = "John"
name = 123 //βοΈ ERROR! Cannot assign value of type 'Int' to type 'String'
So, rest assured, Swift's type inference combined with the compiler's strictness helps catch these type errors early on, before they can cause any issues in your production environment. π¨π©βπ»
π Summary
To sum it all up, here's quick comparison between let
and var
in Swift:
let
is used for defining immutable constants.var
is used for defining mutable variables.Swift infers the type based on the initial value you provide.
The compiler catches type errors to ensure a safer coding experience.
π Take Action!
Now that you've grasped the difference between let
and var
, it's time to put your knowledge into practice! πͺ
Open up your favorite Swift playground or project, and try declaring some let
constants and var
variables. Experiment with assigning different values and modifying them throughout your code.
The more you practice, the more Swift becomes second nature to you! Remember, the journey to mastery starts with a single let
and var
. ππ
If you have any more questions or want to share your experiences with let
and var
, drop a comment below! Let's engage and learn from each other! ππ©βπ»
Happy coding! ππ»