What is an optional value in Swift?
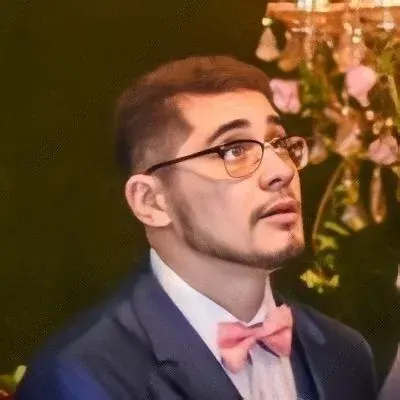
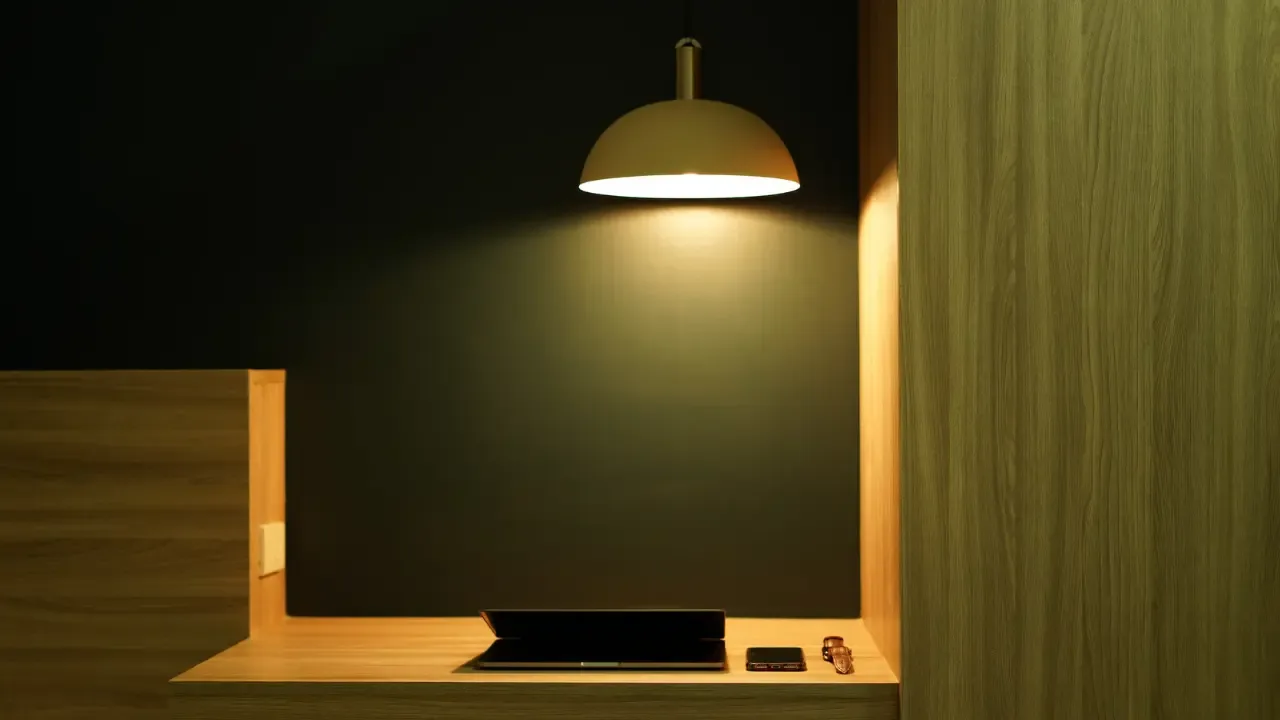
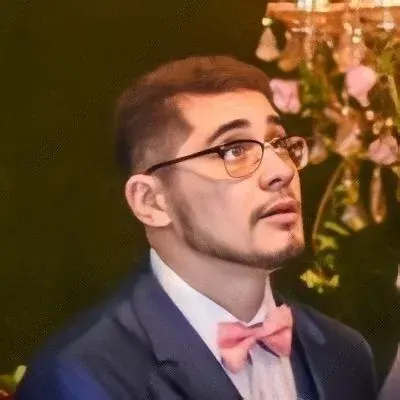
📝 Blog Post: Understanding Optional Values in Swift: A Complete Guide 🚀
Introduction:
Welcome to another exciting edition of our tech blog! Today, we're diving into the fascinating world of optional values in Swift 🌟. But what exactly are optional values, and why would you want to use them? Let's find out!
What are Optional Values?
According to Apple's documentation, optional values in Swift allow us to work with values that might be missing 🎯. They are represented as objects that either contain a value or indicate the absence of a value by using nil
🤔.
To mark a value as optional, all you need to do is add a question mark (?
) after its type declaration, like this:
var name: String?
Common Issues and Problems:
Now that we know what optional values are, let's explore some common issues and problems that developers often encounter when working with them 😓.
Unwrapping Optionals:
One of the most common challenges is unwrapping optional values to access the underlying value. If you try to access an optional value without unwrapping it, you'll end up with a compilation error 😱.
To safely unwrap an optional, you can use an if let
statement. For example:
if let unwrappedName = name {
// Safely use unwrappedName here
} else {
// Handle the case when the optional value is nil
}
Forced Unwrapping:
Another mistake developers tend to make is forcefully unwrapping an optional value using the exclamation mark (!
). Although this can be useful in some cases, it can lead to runtime errors if the optional value is nil
😨.
To avoid these errors, it's always a good practice to use optional binding and conditional statements whenever possible 🧠.
Easy Solutions:
Now, let's explore some easy solutions to these common problems so that you can work smoothly with optional values 🚀.
Default Values:
In situations where the optional value might be nil
, you can provide a default value using the nil-coalescing operator (??
). This ensures that you always have a valid value to work with 🎉.
let fullName = name ?? "Unknown"
Optional Chaining:
Swift provides a powerful feature called optional chaining that allows you to call methods, access properties, and subscript optional values without the need for unwrapping them. This can significantly reduce your code's complexity and make it more readable 😍.
let message = user?.profile?.bio ?? "No bio available"
Call-to-Action:
Optional values in Swift can be a bit tricky, but understanding how to handle them is essential for writing safe and robust code. 🧩
So, don't shy away from embracing optional values in your projects! Dive into the Swift documentation, experiment with the code examples provided, and level up your Swift programming skills! 💪
Share your experiences with optional values in the comments below 👇. Have you encountered any challenges or come up with innovative solutions? We'd love to hear from you!
Happy coding! 😊💻
With this engaging and informative blog post, readers will not only grasp the concept of optional values in Swift but also learn how to tackle common issues and find easy solutions. It encourages them to share their experiences and engage in a conversation, fostering a sense of community among your readers. Happy writing! 🌈🚀