Using a dispatch_once singleton model in Swift
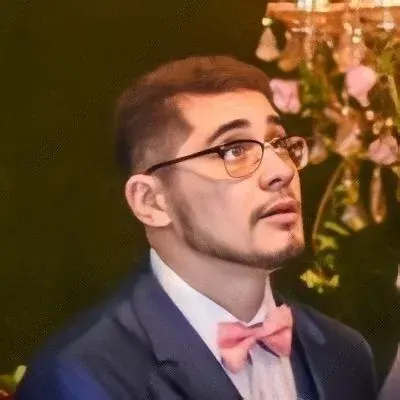
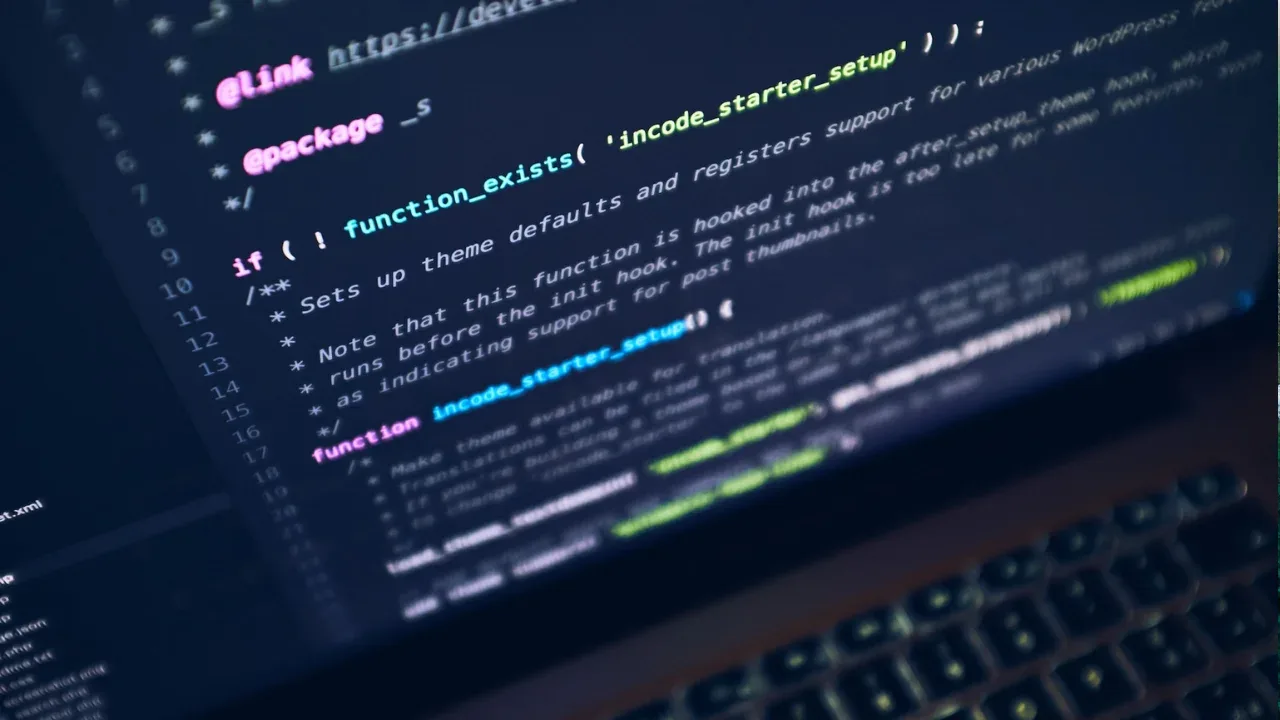
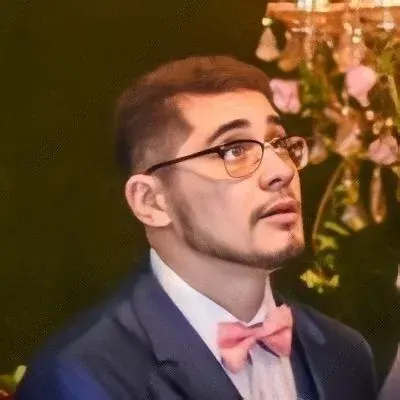
Swift Dispatch_once Singleton Model 🚀💻
Have you ever come across the need to create a singleton instance in your Swift project? Singletons are a great way to ensure a class has only one instance throughout the application. They are commonly used for managing shared resources or maintaining global state.
In this blog post, we will dive into implementing a thread-safe singleton model using dispatch_once
in Swift. We'll address the common issues faced and provide you with an easy-to-understand solution.
The Problem 😫
The code snippet you provided shows a non-thread safe singleton implementation. While it works well in most cases, it fails to ensure thread safety. Multiple threads could potentially create different instances of the singleton, leading to unexpected behavior and inconsistent state.
The Solution 🌟
To make your singleton thread-safe, you can leverage the power of the dispatch_once
function provided by Apple's Grand Central Dispatch (GCD) framework. This function ensures that a block of code is executed only once during the entire lifespan of an application, no matter how many times it is called.
To implement dispatch_once
in your singleton model, consider the following code:
class TPScopeManager {
static let sharedInstance = TPScopeManager()
// Other properties and methods
private init() {
// Initialization code
}
}
This approach takes advantage of Swift's static constants and guarantees that the sharedInstance
is initialized only once, regardless of the thread calling it.
How it works 🛠️
When generating the static constant, Swift guarantees that the initialization code is executed atomically and lazily. This means that the sharedInstance
is created the first time it is needed and is thread-safe by default, thanks to Swift's lazy property initialization.
Addressing the Compiler Error 🚦
Now, let's tackle the compiler error you encountered while using dispatch_once
.
The error message Cannot convert the expression's type 'Void' to type '()'
is due to the mismatch in the signature of the closure accepted by dispatch_once
and the closure you provided. In Swift, Void
and ()
are interchangeable, representing an empty tuple.
To resolve this error, use the following syntax:
class var sharedInstance: TPScopeManager {
struct Static {
static let instance: TPScopeManager = TPScopeManager()
static var token: dispatch_once_t = 0
}
dispatch_once(&Static.token) {
Static.instance = TPScopeManager()
}
return Static.instance
}
Notice that we changed var
to let
for instance
, as the singleton instance should be immutable once initialized. Additionally, we passed the address of the token
to dispatch_once
using the &
operator.
Conclusion 🎉
By implementing a thread-safe singleton using dispatch_once
and Swift's static constants, you can rest assured that your singleton instance will be created only once, eliminating any concurrency issues.
Remember, it's crucial to understand the potential threading concerns when working with singletons. Singleton usage should always be weighed against alternative solutions, such as dependency injection, to ensure the best design for your project.
Now that you have a solid understanding of dispatch_once in Swift, go ahead and give it a try in your own projects! Feel free to reach out if you have any questions or want to share your experiences. Happy coding! 😊✨
Keep learning, keep building, and keep sharing! 🚀