SwiftUI: How to implement a custom init with @Binding variables
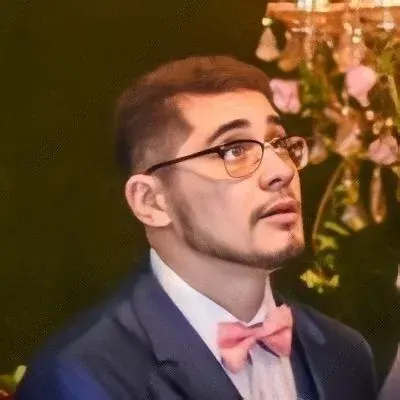
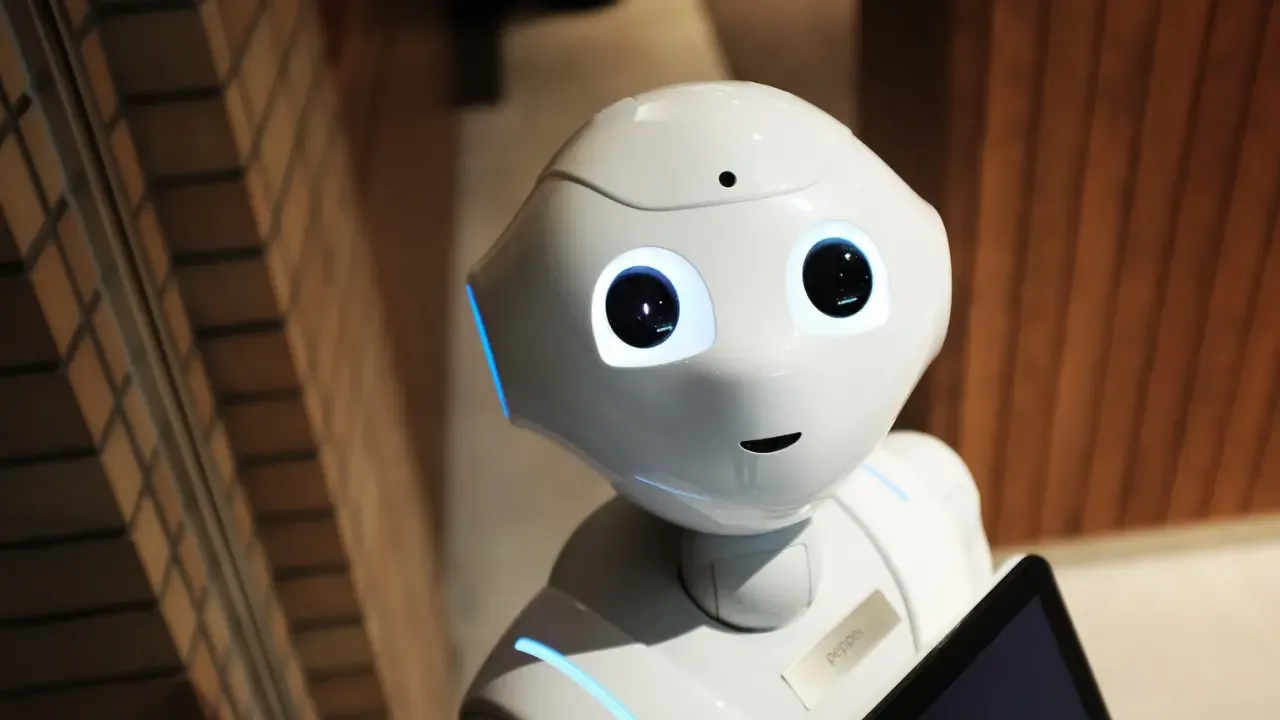
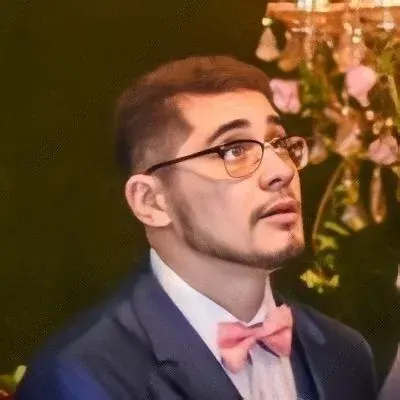
SwiftUI: How to implement a custom init with @Binding variables 😎💡
Are you working on a money input screen using SwiftUI and facing difficulties in implementing a custom init
method with @Binding
variables? Don't worry, we've got you covered! In this blog post, we'll address the common issue of initializing a state variable based on the passed in amount and provide you with easy solutions. Let's dive in! 💪
The Problem 🧐
You encountered a compiler error when trying to implement the custom init
method in your AmountView
struct. The error message states: "Cannot assign value of type 'Binding' to type 'Double'". This error occurs because you cannot directly assign a value of type Binding
to a property of type Double
. But don't lose hope! We have a solution for you. 🚀
The Solution 🎉
To resolve the error and implement a custom init
method with @Binding
variables, you need to create a temporary variable to store the value of the Binding
and then assign it to the desired property. Here's the updated code snippet:
struct AmountView : View {
@Binding var amount: Double
@State var includeDecimal = false
init(amount: Binding<Double>) {
self._amount = amount // Use the underscore prefix to access the wrapped value of the Binding
self._includeDecimal = State(initialValue: round(amount.wrappedValue) - amount.wrappedValue > 0)
}
}
In the updated code, we use the underscore prefix (_amount
) to access the wrapped value of the Binding
and assign it to the amount
property. Similarly, we use the underscore prefix (_includeDecimal
) to access the wrapped value of the State
property.
By making these changes, we resolve the compiler error and correctly initialize the includeDecimal
state variable based on the initialized amount.
Test It Out! ✅
Don't just take our word for it; give it a try! Implement these changes in your code and see how it works for yourself. We would love to hear your feedback! 😄
If you have any questions or face any issues, feel free to reach out to us via the comments section or contact us on our website. We are here to support you every step of the way!
Conclusion 📝
In this blog post, we discussed how to implement a custom init
method with @Binding
variables in SwiftUI. By creating a temporary variable to store the value of the Binding
and then assigning it to the desired property, we resolved the compiler error and successfully initialized the state variable.
Remember, SwiftUI is all about flexibility and easy customization. Don't be afraid to experiment and explore different solutions! Happy coding! 👩💻👨💻
Share Your Experience! 📣
We would love to hear about your experiences with implementing a custom init
method with @Binding
variables in SwiftUI. Share your thoughts, challenges, and solutions with our vibrant community in the comments below. Let's learn and grow together! 💪💬