Swift: print() vs println() vs NSLog()
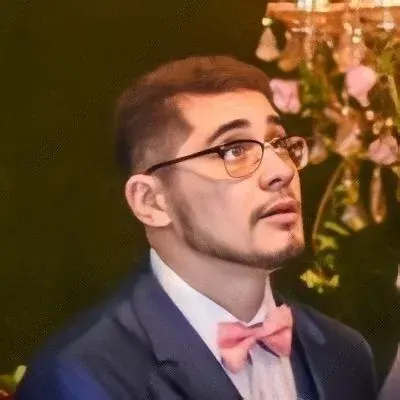
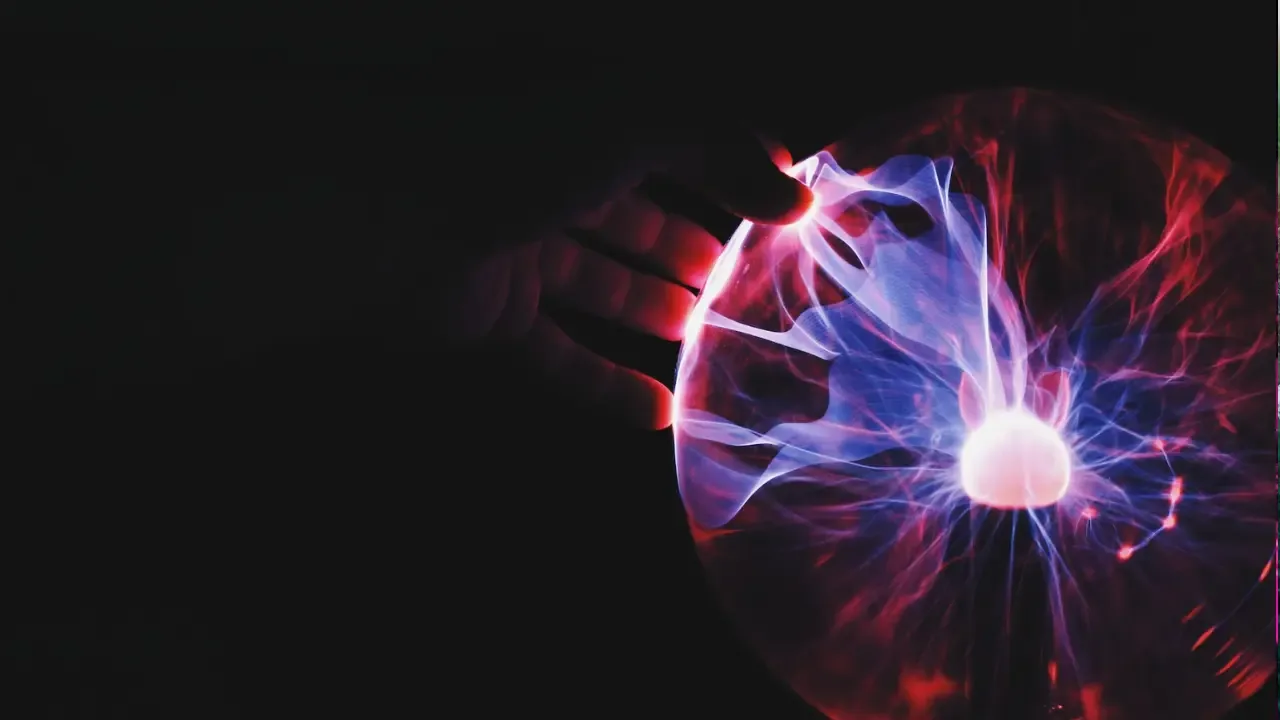
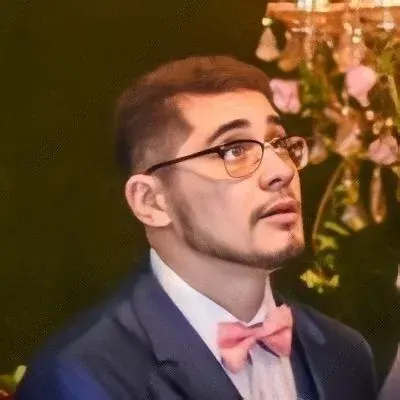
Swift: print() vs println() vs NSLog()
So you're new to Swift and wondering what's the deal with these three printing functions: print()
, println()
, and NSLog()
.💭
Let's break it down!🔍
print()
The print()
function is a built-in function in Swift that prints the specified values you provide. It is similar to the print()
function in other programming languages like Python, JavaScript, and Ruby.👍
Here's an example:
let message = "Hello, World!"
print(message)
This will simply print "Hello, World!"
in the console.
Now, what about the other two functions?🤔
println()
The println()
function was available in earlier versions of Swift but has been deprecated since Swift 2.⚠️
In Swift 2 and later versions, you should use print()
instead. So forget about println()
!🙅♂️
NSLog()
NSLog()
is not actually a Swift function, but rather a function from the Objective-C runtime library. It is used to output log messages that can be helpful for debugging purposes.🐞
Here's an example:
let message = "Debugging info"
NSLog("%@", message)
This will output the message "Debugging info"
along with additional information like the date, time, and process ID.
So in summary, use print()
for general-purpose printing, and NSLog()
for more advanced debugging or logging purposes.
Now that you know the differences, you can confidently choose the appropriate printing function in Swift.🎉
Conclusion
Printing values in your Swift code is essential for debugging and understanding your program's behavior. By utilizing the print()
function for general-purpose printing and NSLog()
for advanced debugging, you'll have the tools you need to navigate any printing scenario.💪
Remember, println()
is a thing of the past, so always stick with print()
in your Swift code.
Now go forth and print away!🚀
Have any questions or other Swift topics you'd like me to cover? Let me know in the comments below!📝