Swift - How to convert String to Double
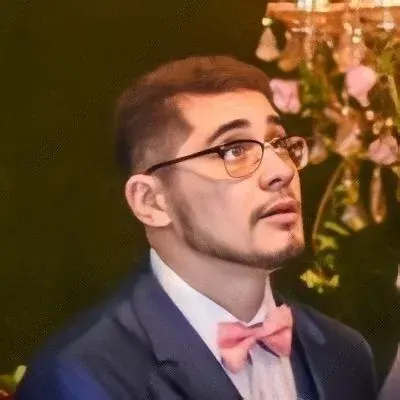
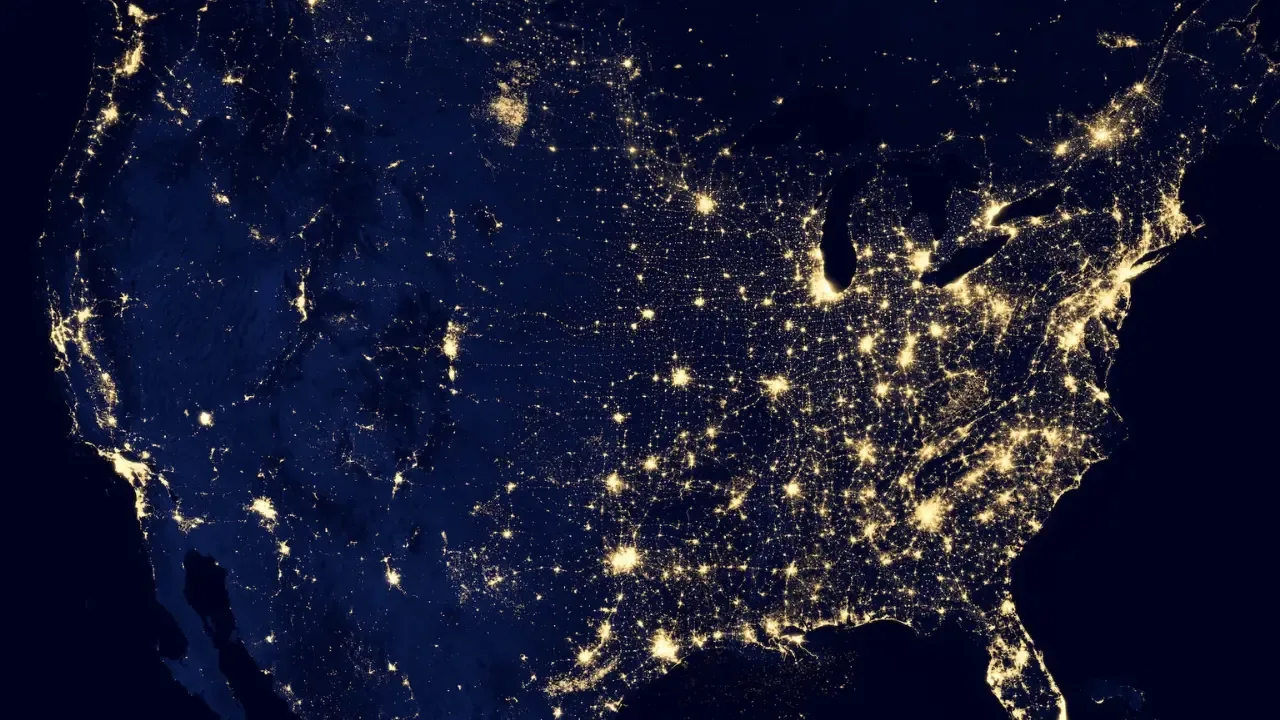
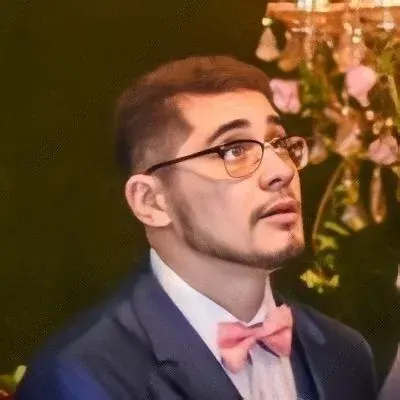
How to Convert String to Double in Swift
š Hey there, š©āš» tech enthusiasts! Are you trying to create a BMI program in Swift and facing the problem of converting a String to a Double? Well, you've come to the right place! In this blog post, we'll discuss some common issues around converting a String to a Double in Swift and provide you with easy solutions. Let's dive in! š¦
The Problem
š¤ So, you want to convert a String to a Double in Swift, just like you could do in Objective-C with [myString doubleValue]
, but you're not sure how to achieve the same in Swift. Don't worry, it's a common challenge when you're transitioning from Objective-C to Swift. š
Solution 1: Using the Double()
Initializer
⨠In Swift, you can convert a String to a Double using the Double()
initializer. Here's how you can do it:
let myString = "3.14"
if let myDouble = Double(myString) {
// Use myDouble here
} else {
// Handle the case if the conversion fails
}
In the above code snippet, we try to initialize a Double instance myDouble
with the value of the myString
String. If the conversion succeeds, the if
block will be executed and you can use myDouble
within that block. Otherwise, if the conversion fails, the else
block will be executed, and you can handle the failure scenario accordingly. š
Solution 2: Using the NSNumberFormatter
š Another way to convert a String to a Double in Swift is by using the NSNumberFormatter
, which provides more flexibility for customizing the conversion process. Here's an example:
let myString = "3.14"
let formatter = NumberFormatter()
if let myNumber = formatter.number(from: myString) {
let myDouble = myNumber.doubleValue
// Use myDouble here
} else {
// Handle the case if the conversion fails
}
In this code snippet, we create an instance of the NumberFormatter()
and then use its number(from:)
method to convert the myString
String to an NSNumber
object. From there, we can access the doubleValue
property of the NSNumber
object and store it in myDouble
. Again, you can handle the failure scenario in the else
block if the conversion fails. š
Conclusion
š There you have it! You now know two easy ways to convert a String to a Double in Swift. Whether you choose to use the Double()
initializer or the NSNumberFormatter
, you'll be able to perform this conversion seamlessly. Feel free to experiment and choose the approach that suits your needs best. š¬
š If you found this blog post helpful or have any further questions or tips related to converting Strings to Doubles in Swift, please share them with us in the comments below. Let's learn and grow together! š±āØ