Rounding a double value to x number of decimal places in swift
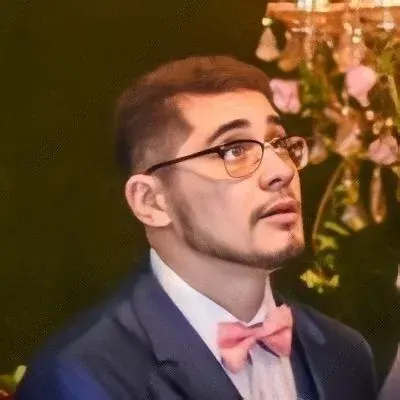
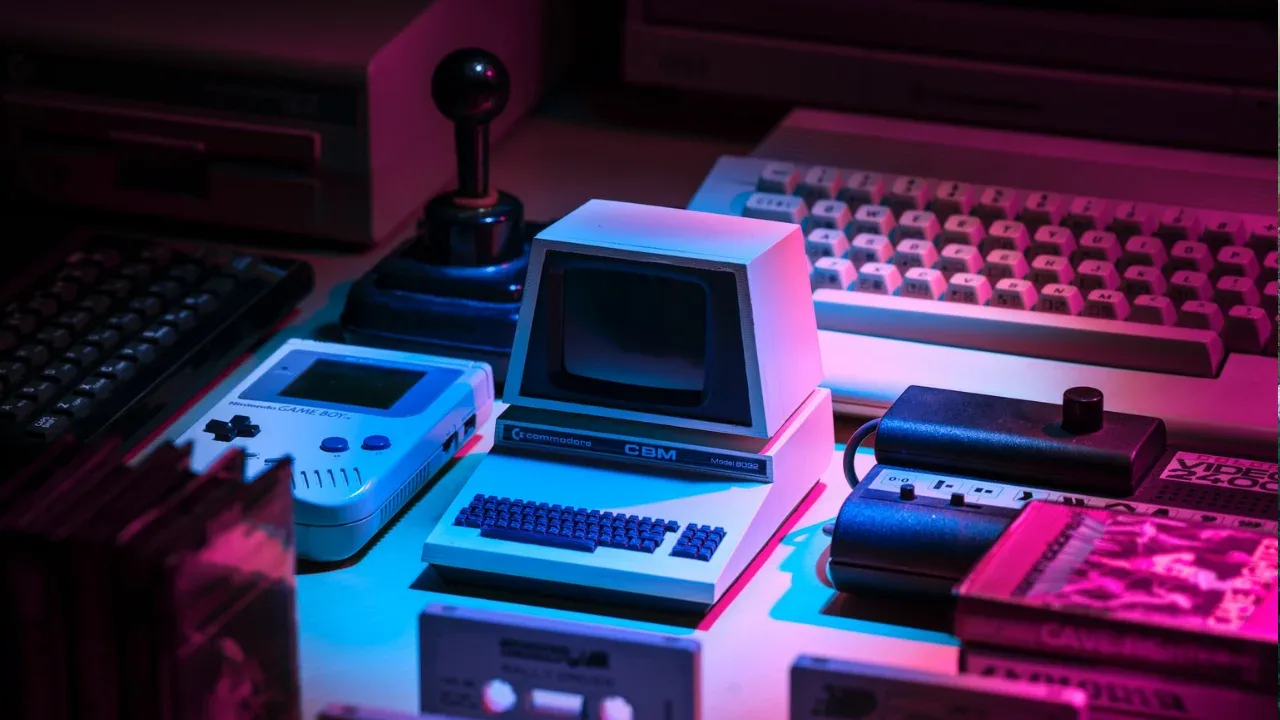
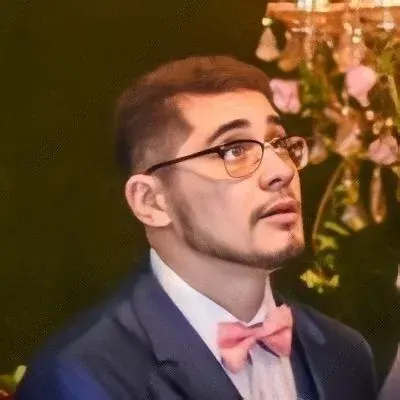
How to Round a Double Value in Swift
š Hey there! Are you struggling with rounding a double value to a specific number of decimal places in Swift? Don't worry, we've got you covered! In this blog post, we'll discuss a common issue that developers face and provide easy solutions to help you round your double values like a pro. Let's get started, shall we? š
The Problem
So, you have a double value (totalWorkTime
) that represents an NSTimeInterval in seconds and you want to round it down to a specific number of decimal places. In your case, you want to round it to three decimal places. Let's take a closer look at your code:
var totalWorkTimeInHours = (totalWorkTime/60/60)
š¤ When you print totalWorkTimeInHours
, you get a long and precise number like 1.543240952039...
instead of the rounded value you desire (1.543
). Don't worry, we've got some solutions for you!
Solution #1: Using the String(format:)
Method
One way to round your double value to a specific number of decimal places is by converting it to a string using the String(format:)
method. Here's how you can do it:
let roundedValue = String(format: "%.3f", totalWorkTimeInHours)
print(roundedValue) // Output: 1.543
In the above code, "%.3f"
specifies that we want to format the double value (totalWorkTimeInHours
) with three decimal places. The String(format:)
method takes care of rounding and formatting the value for us. Pretty cool, right? š
Solution #2: Using the Decimal
Type
Another approach to rounding a double value is by utilizing the Decimal
type in Swift. Here's an example:
let decimalValue = Decimal(totalWorkTimeInHours)
let roundedValue = decimalValue.rounding(accordingToBehavior: NSDecimalNumberHandler(roundingMode: .plain, scale: 3, raiseOnExactness: false, raiseOnOverflow: false, raiseOnUnderflow: false, raiseOnDivideByZero: false))
print(roundedValue) // Output: 1.543
In this solution, we create a Decimal
value from our double value (totalWorkTimeInHours
). Then, we use the rounding(accordingToBehavior:)
method to specify the rounding behavior with the desired number of decimal places (3 in our case). The result is the rounded value we were looking for. š
Conclusion
And there you have it! Two easy solutions to round a double value to a specific number of decimal places in Swift. You can choose the method that best fits your needs and integrate it into your code with ease.
Now it's your turn to try it out and let us know how it goes! Do you have any other clever approaches to rounding double values? Share them in the comments below. We'd love to hear from you! š
Stay tuned for more informative and engaging blog posts. Don't forget to hit that share button to spread the knowledge with your fellow developers. Happy coding! āļø