Read and write a String from text file
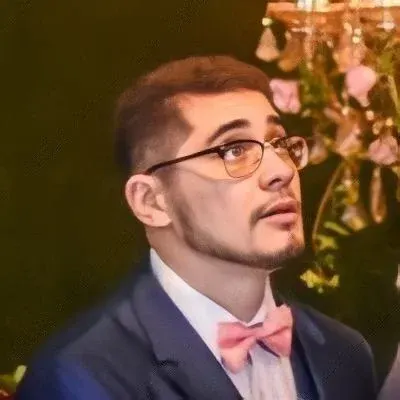
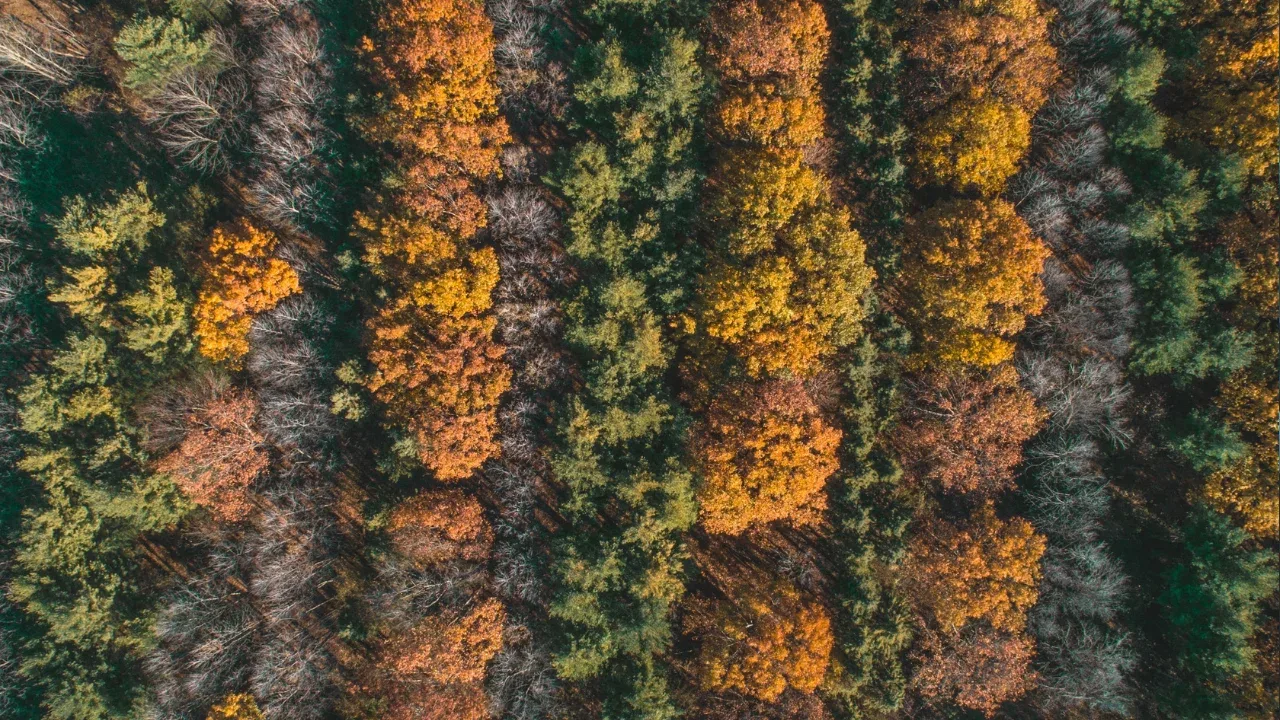
š Tech Blog Post: Easy Ways to Read and Write a String from a Text File in Swift š
Hello there, tech enthusiasts! š
Are you struggling to read and write data from a text file in Swift? Don't worry, you're not alone! Many developers face this issue when working with file operations. But fear not, as I'll guide you through the process in this blog post, providing easy solutions to help you overcome this challenge. š
š Understanding the Problem
The given context mentions the need to read and write data to a text file but lacks information about the desired file operations. In Swift, we can accomplish this using file handling techniques and the built-in Filemanager
class.
š” Easy Solutions
Reading data from a text file:
To read data from a text file, you can use the String
initializer, passing the contents of the file as the parameter. Here's an example:
if let filepath = Bundle.main.path(forResource: "filename", ofType: "txt") {
do {
let contents = try String(contentsOfFile: filepath)
print(contents)
} catch {
print("Error reading file: \(error)")
}
}
In this code snippet:
Replace
"filename"
with the actual name of your text file.Specify the file type in place of
"txt"
, such as"csv"
or"json"
, depending on your file's format.
Writing data to a text file:
To write data to a text file, you can use the write
method of the String
class. Here's a straightforward example:
let text = "Some data to write to the file."
let filePath = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask)[0].appendingPathComponent("filename.txt")
do {
try text.write(to: filePath, atomically: true, encoding: .utf8)
print("Data written successfully.")
} catch {
print("Error writing data: \(error)")
}
Just remember:
Replace
"filename.txt"
with the desired name for your text file.Customize the
text
variable to contain the data you want to write.
š£ Take Action! Engage with our Community
Congratulations! š You've learned easy ways to read and write data from a text file in Swift. Now it's your turn to put this knowledge into practice and share your experience with the community.
š Try reading and writing data to different file types. š Experiment with larger data sets or complex file structures. š Share your findings and ask questions on our tech forum or in the comments section below.
Remember, the tech world thrives on collaboration and knowledge sharing. By engaging with others, you not only expand your own skills but also help fellow developers facing similar challenges. Let's learn together! šŖ
So, what are you waiting for? Grab your code editor, unleash your creativity, and start exploring the fascinating world of file operations in Swift. š
I hope this blog post has empowered you to seamlessly read and write strings from files in Swift. If you have any questions or suggestions for future blog posts, feel free to reach out. Happy coding! šš»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
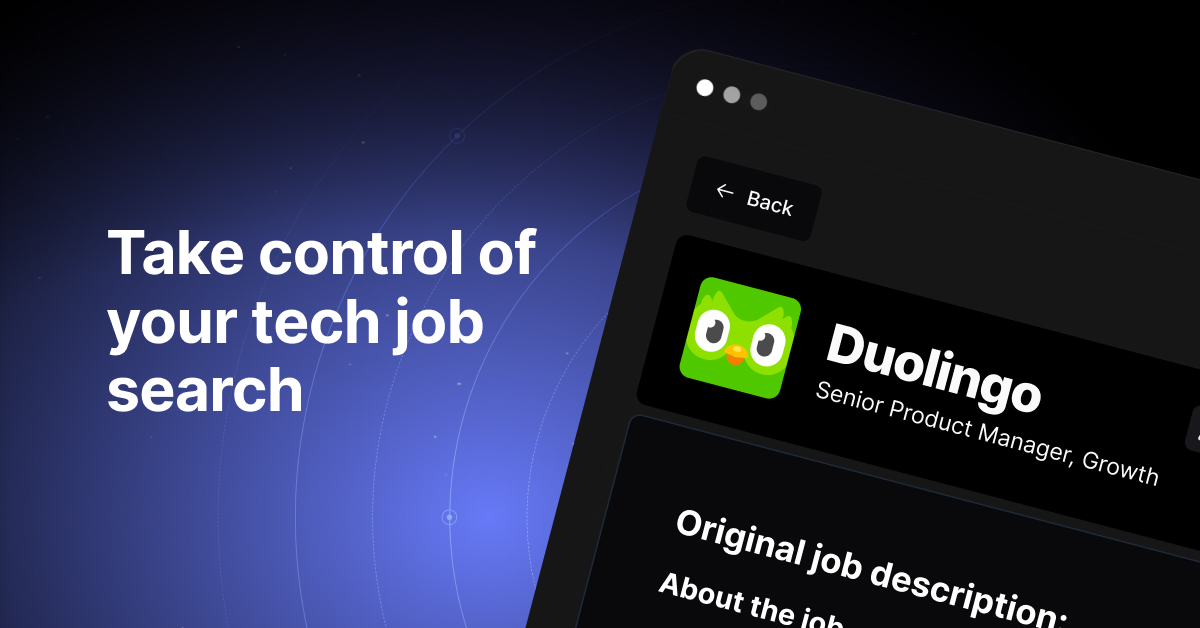