Printing a variable memory address in swift
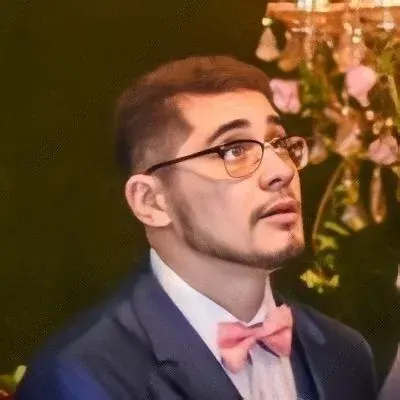
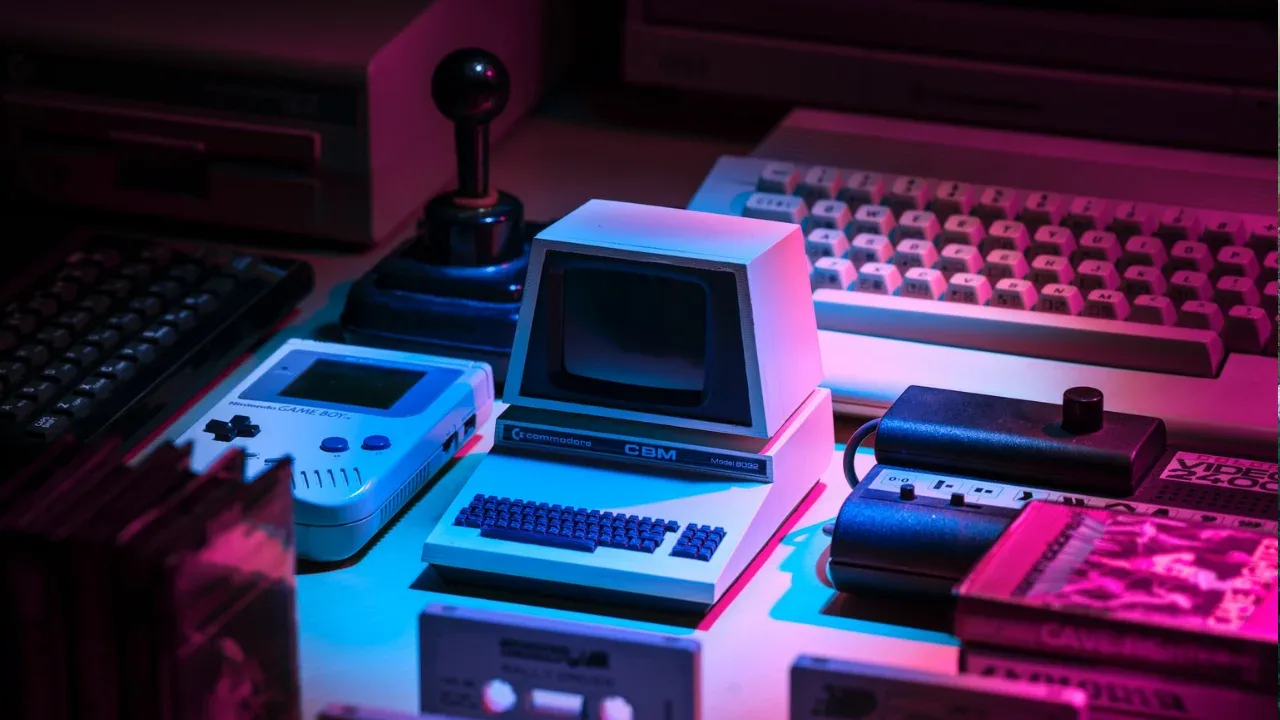
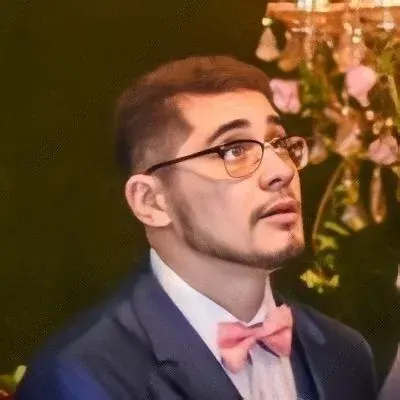
🖨️ Printing a Variable Memory Address in Swift
Have you ever wondered how to print the memory address of a variable in Swift? It's a useful technique when debugging or understanding how memory is allocated within your code. In this blog post, we will explore common issues related to printing variable memory addresses, provide easy solutions, and encourage reader engagement. Let's dive in! 🏊♀️
The Problem
Many developers are familiar with the %p
format specifier in Objective-C, which allows us to print the memory address of a variable using NSString stringWithFormat
. However, in Swift, there is no built-in equivalent. So how can we achieve the same functionality in Swift? 🤔
The Solution
Fortunately, there is a simple solution to this problem. We can make use of the Unmanaged
type in Swift, along with the bitPattern
property. Here's an example:
let str = "A String"
let strAddress = Unmanaged.passUnretained(str as AnyObject).toOpaque()
print("str value \(str) has address: \(strAddress)")
In the above code, we create an instance of Unmanaged
, passing the string object using passUnretained
. Then, we access the toOpaque()
method to obtain the memory address as a pointer value. Finally, we print the address along with the original string value.
By using Unmanaged
and toOpaque()
, we can effectively print the memory address of any variable in Swift! 🎉
Common Issues
1. Printing the Memory Address of Non-Reference Types
Keep in mind that the above solution works only for reference types (classes) and not value types (structs, enums, and basic types like Int
or Double
). For value types, the memory address isn't directly accessible, as they are usually stored inline within the stack frame.
To print the memory address of a value type, you need to box it as a reference type using NSNumber
, NSString
, or similar classes. Then, you can apply the same technique described above to obtain the memory address.
2. Mutability and Lifetime Considerations
Be cautious when printing the memory address of a variable, especially if it's a mutable reference type. The memory address may change during the lifetime of an object, especially if it undergoes reassignment or memory management operations. Therefore, the memory address may not be the same throughout your code execution.
To ensure the accurate representation of a variable's memory address, consider capturing it within a closure or taking a snapshot at a specific point in your code.
Conclusion
Printing the memory address of a variable in Swift provides valuable insights into how memory is managed within your code. By using the Unmanaged
type and toOpaque()
, you can easily print the memory address and pair it with the original value.
Next time you encounter a tricky memory-related issue, try printing the memory address of the variable to gain a deeper understanding of what's happening under the hood. 🚀
Now it's your turn! Have you ever used memory addresses in your Swift code? Share your experiences, tips, or questions in the comments section below. Let's continue the conversation! 💬