Precision String Format Specifier In Swift
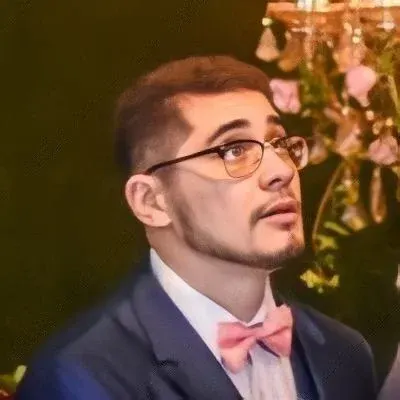
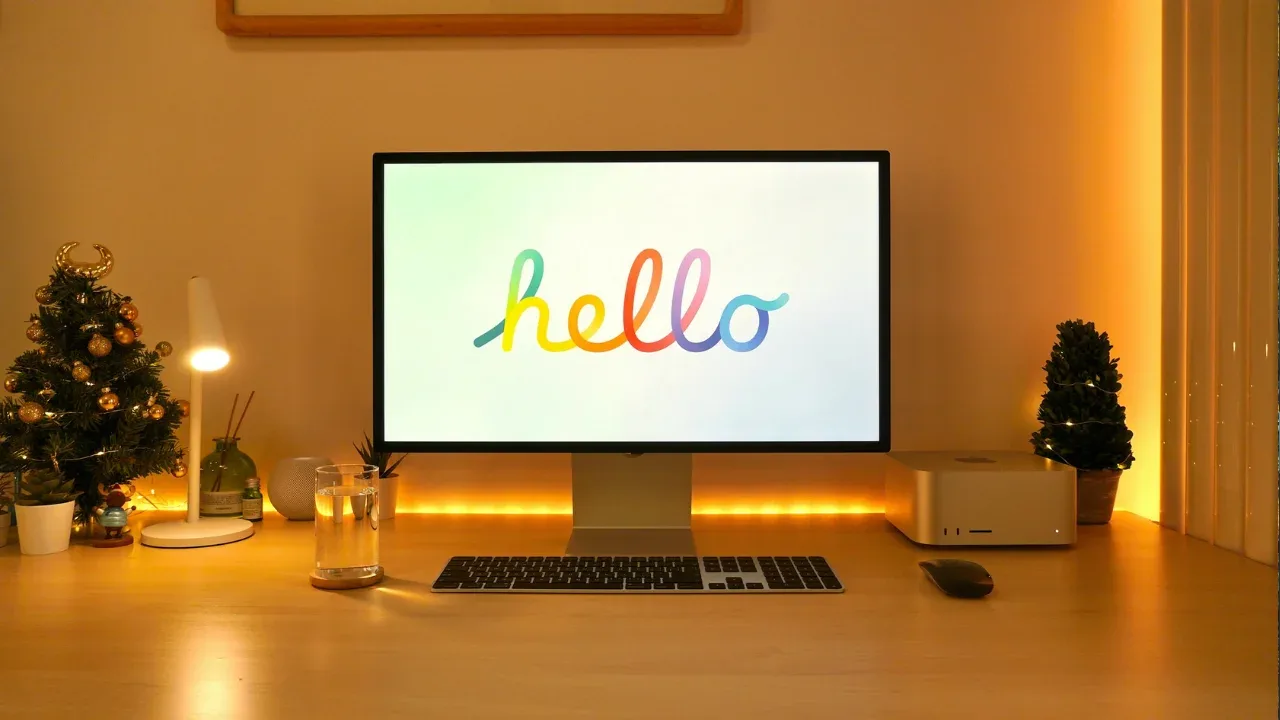
Precision String Format Specifier in Swift: A Guide to Formatting Floating-Point Numbers in Style!
Have you ever struggled with formatting floating-point numbers in Swift, wanting to truncate or round them to a specific number of decimal places? 🤔 Well, worry no more! In this guide, we will explore the precision string format specifier in Swift and provide you with easy solutions to common formatting issues.
Understanding the Problem:
The initial question mentioned using the NSLog
function in Objective-C to truncate a float to two decimal places. While that solution works in Objective-C, the syntax in Swift is a bit different. But fret not, because Swift offers an even more powerful and concise way to achieve the desired result.
The Precision String Format Specifier in Swift:
In Swift, the precision string format specifier is represented by %.<num>f
, where <num>
represents the number of decimal places you want to display. Let's see how you can utilize this specifier to format floating-point numbers in Swift.
Truncating a Floating-Point Number:
To truncate a floating-point number to a specific number of decimal places, you can use the precision specifier in string interpolation. Here's an example:
let floatValue = 3.14159265
let truncatedString = String(format: "%.2f", floatValue)
print(truncatedString) // Output: 3.14
In the code snippet above, we use the String(format: _, _)
constructor to format the floatValue
. The format string %.2f
specifies that we want the number truncated to two decimal places.
Rounding a Floating-Point Number:
If you need to round a floating-point number instead of truncating it, you can leverage the NumberFormatter
class in Swift. Here's how you can achieve rounding to a specific number of decimal places:
let floatValue = 3.14159265
let numberFormatter = NumberFormatter()
numberFormatter.numberStyle = .decimal
numberFormatter.roundingMode = .halfUp
numberFormatter.maximumFractionDigits = 2
if let roundedString = numberFormatter.string(from: NSNumber(value: floatValue)) {
print(roundedString) // Output: 3.14
}
In this example, we're creating a NumberFormatter
instance and setting its properties: numberStyle
, roundingMode
, and maximumFractionDigits
. Finally, we're using numberFormatter.string(from: _)
to format the floatValue
and obtain the rounded string.
Your Turn:
Now that you have learned about the precision string format specifier in Swift, how about trying it out in your own projects? Whether it's truncating or rounding floating-point numbers, this knowledge will surely come in handy. 🚀
If you have any questions or want to share your thoughts on this topic, feel free to leave a comment below. Let's dive into the world of precision number formatting together! 💡
Keep coding and stay tuned for more exciting tech tips! Until next time, happy coding! 😄
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
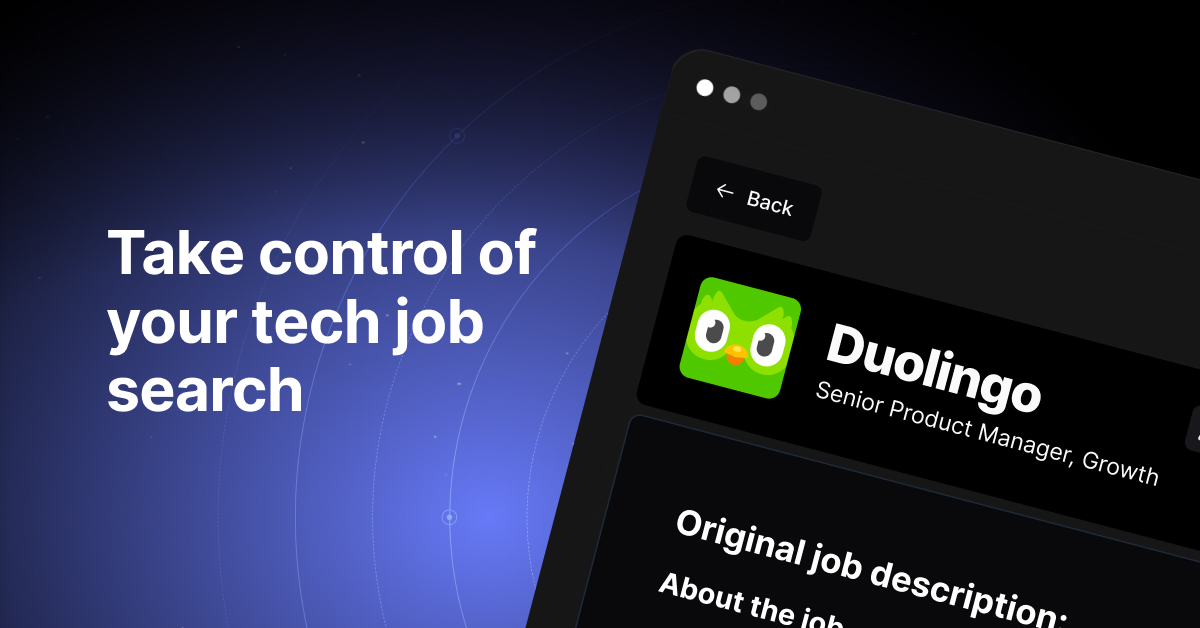