How to program a delay in Swift 3
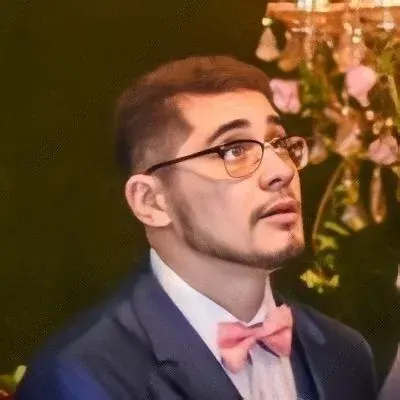
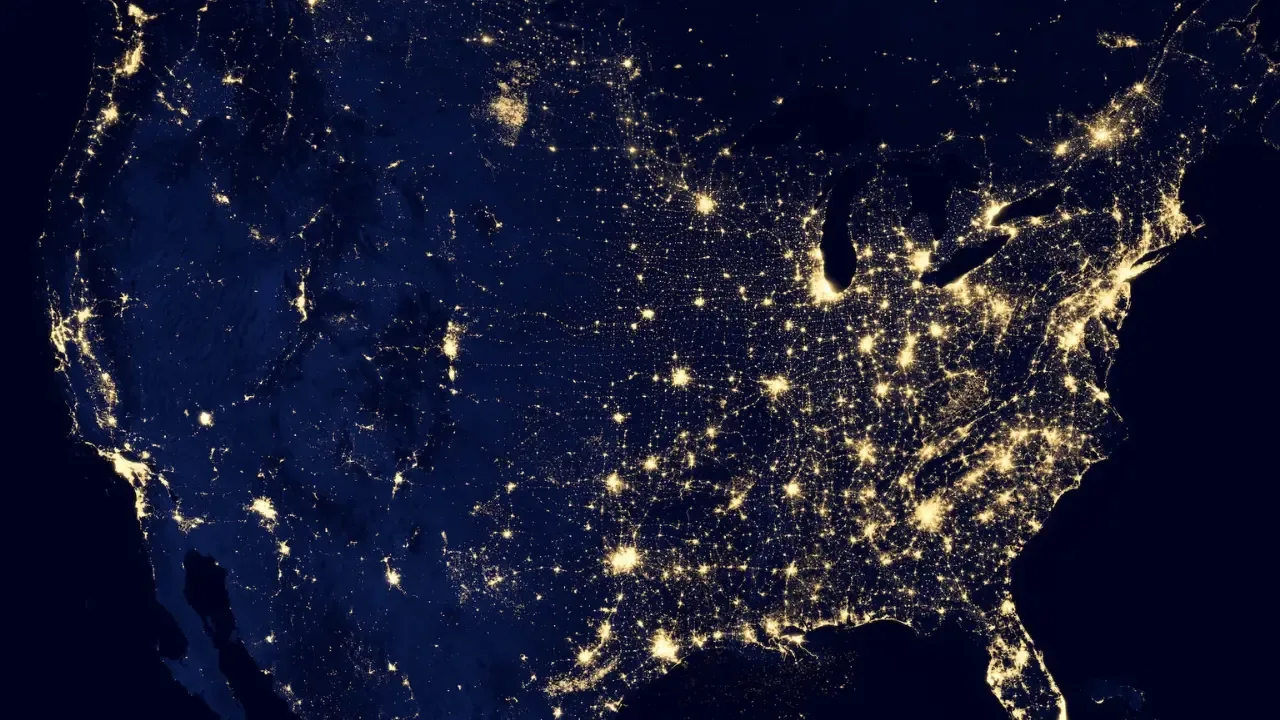
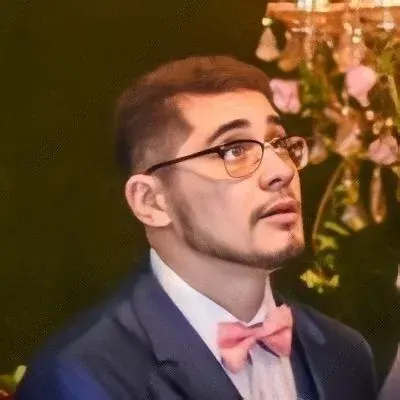
How to Program a Delay in Swift 3: Solving the Dispatcher Dilemma 🕐🚀
Have you ever needed to create a delay before running a sequence of code in Swift 3? 🤔
In earlier versions of Swift, the solution was as simple as using the dispatch_after
function. However, with the introduction of Swift 3 and Xcode's automatic code migration, a common problem arises: the dreaded "Cannot convert DispatchTime.now
to expected value dispatch_time_t
aka UInt64
" error. 😱
But fear not, fellow developers! We have come to your rescue with an easy and straightforward solution to this dilemma. 👊💥
The Obstacle: Dispatcher vs. DispatchTime ⏲️❌⌛
In Swift 3, the dispatch_
prefix has been replaced with Dispatch
for improved readability and clarity. This shift has led to changes in code that once worked like a charm. 😢
Before diving into the solution, let's quickly recap how we used to create a delay in earlier versions of Swift:
let time = dispatch_time(dispatch_time_t(DISPATCH_TIME_NOW), 4 * Int64(NSEC_PER_SEC))
dispatch_after(time, dispatch_get_main_queue()) {
//put your code which should be executed with a delay here
}
This code served us well until we migrated to Swift 3, where Xcode automatically refactors the code in 6 different ways. Unfortunately, this refactoring unexpectedly generates an error. 🙅♂️❌
The Solution: The Awaited Dispatch 🤞✨
So, how do we create a delay in Swift 3 without encountering the "Cannot convert" error? 🤔
The answer lies in embracing the new Swift 3 syntax and using the DispatchQueue
class, specifically its asyncAfter
method. This method allows us to execute a block of code after a specified delay. 💡📝
Here's the updated and correct syntax for Swift 3:
DispatchQueue.main.asyncAfter(deadline: .now() + 4) {
//put your code which should be executed with a delay here
}
Now, isn't that sleek and concise? 🤗
Putting It All Together: Solving the Delay Dilemma 🔄🔁
To summarize, here's a step-by-step guide to creating a delay in Swift 3:
Replace the old
dispatch_time
function withDispatchQueue.main.asyncAfter
.Use the
deadline
parameter and set it to.now() + <desired delay>
.Insert your code within the closure that follows the
asyncAfter
method.
With these changes, you'll be able to create a delay and execute your code without any errors. 🎉
Join the Delay Party! 🎈🎊
Now that you've mastered the art of creating a delay in Swift 3, it's time to put your newly acquired knowledge into action! 💪
Challenge yourself to find creative ways to leverage delays in your projects and share your experiences with us in the comments below. 📝 We can't wait to hear about your exciting delay-driven adventures! 🚀
Happy coding! 😊👩💻👨💻