How to generate a random number in Swift?
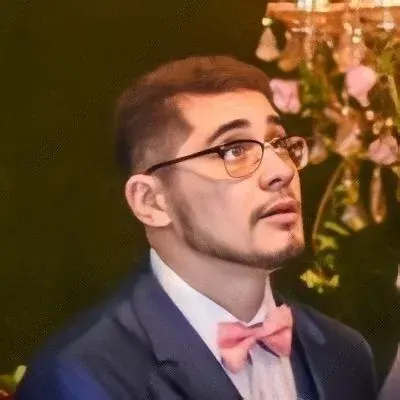
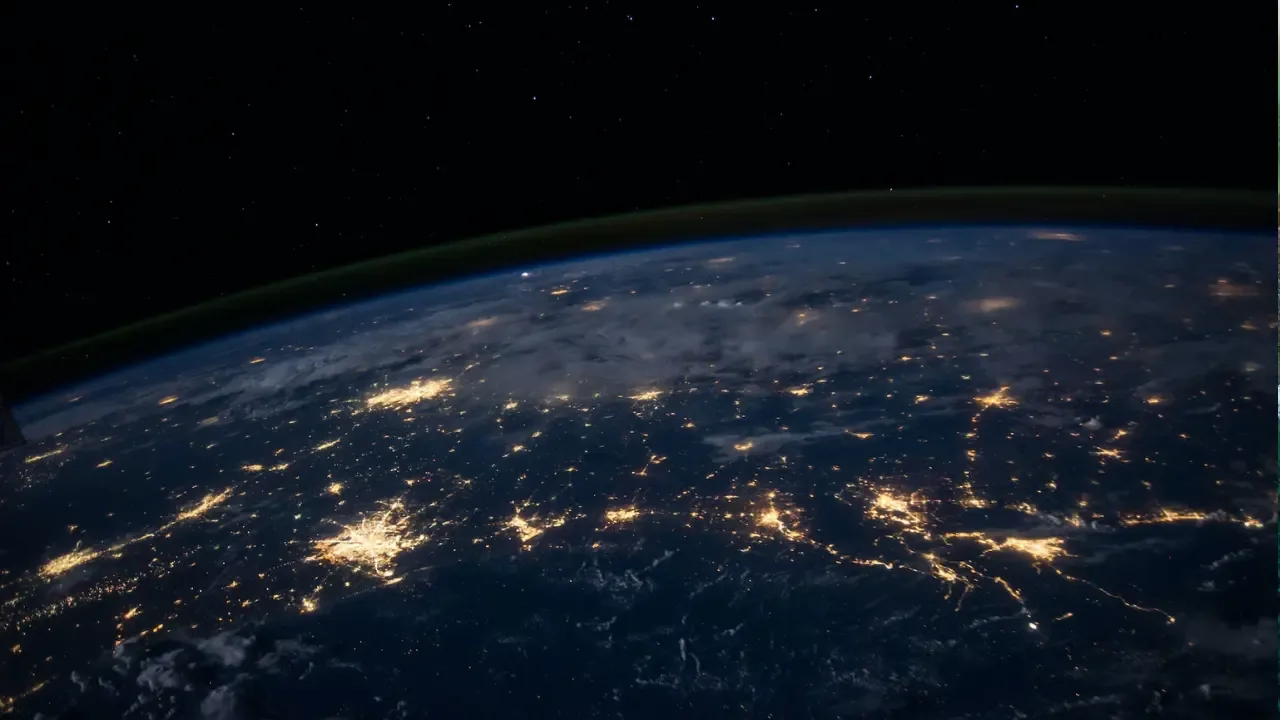
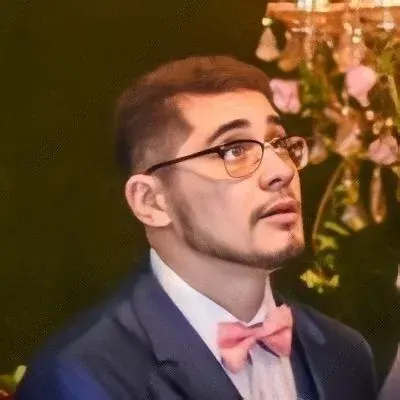
๐ฒ๐ฑHow to Generate Random Numbers in Swift
Have you ever wanted to add a touch of unpredictability to your Swift app? Maybe you're working on a game and need random values for enemy placement or generating loot. Or perhaps you're developing a decision-making app that needs to make random choices. Whatever the case may be, generating random numbers in Swift is a common task that every developer should know how to do.
While the Swift book provides an implementation for a random number generator, you might be wondering if there's a better way to go about it. ๐ค Should you simply copy and paste the code from the book, or is there a library that provides a more robust solution? Let's dive into it! ๐ฅ
The Swift Standard Library Solution
As of Swift 4.2, you can use the arc4random_uniform
function from the Darwin module to generate random numbers. Here's how you can use it:
import Darwin
let randomNum = arc4random_uniform(100) // Generates a random number between 0 and 99
This solution works, but it has a couple of drawbacks. First, arc4random_uniform
only works with integers and you need to specify the upper bound yourself. Second, it returns a UInt32
value, so if you need a different type of number, you'll have to cast it yourself.
The GameplayKit Approach
If you're looking for more flexibility and control, ๐ฎ GameplayKit provides a more robust solution for generating random numbers. Here's how you can do it:
import GameplayKit
let randomNum = GKRandomSource.sharedRandom().nextInt(upperBound: 100) // Generates a random number between 0 and 99
Not only does GameplayKit offer a range of random number generation methods, but it also supports different distributions, allowing you to generate numbers according to specific probability distributions. This is perfect for simulating dice rolls or any scenario where certain outcomes are more likely than others.
Conclusion
Generating random numbers in Swift doesn't have to be a complicated process. While the Swift standard library provides a basic solution with arc4random_uniform
, consider using GameplayKit for a more powerful and flexible approach.
So next time you need to add a sprinkle of randomness to your app, remember these options and choose the one that suits your needs best. ๐คฉ
What are you waiting for? ๐ Start incorporating random numbers into your Swift app today and watch your app come to life! Share your experiences and thoughts in the comments below. Happy coding! ๐ป๐กโจ