How to enumerate an enum with String type?
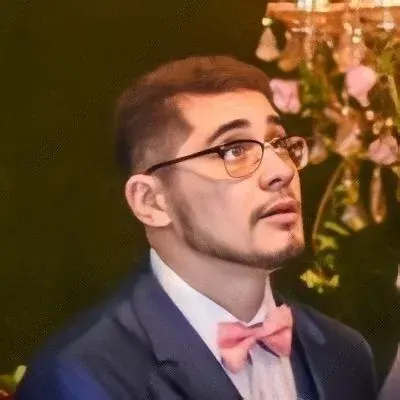
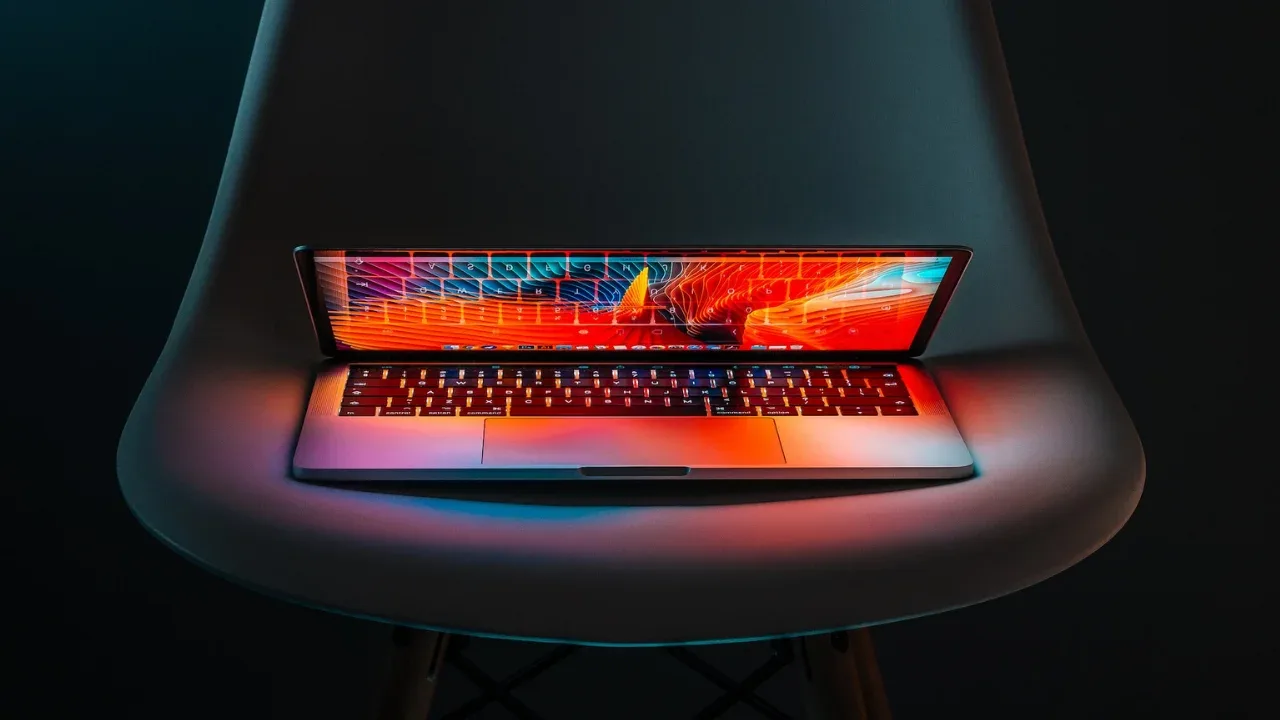
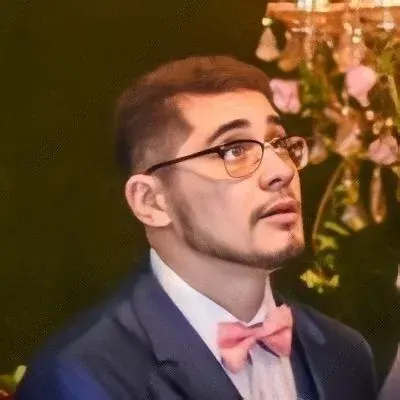
🃏 How to Enumerate an Enum with String Type in Swift? 🎴
Do you ever find yourself with an enum that has string values and wonder how to iterate over each case? 🤔 Fear not, because in this guide, we will show you how to enumerate an enum with a string type in Swift and provide you with an easy solution! 💪
The Enum Dilemma 😱
Let's say we have the following enum called Suit
with string values representing different card suits: ♠️, ♥️, ♦️, and ♣️.
enum Suit: String {
case spades = "♠️"
case hearts = "♥️"
case diamonds = "♦️"
case clubs = "♣️"
}
Now, imagine you want to perform some operation or print all the raw values of each suit in a loop. You might initially think that using a for-in
loop would do the trick, just like in the following code snippet:
for suit in Suit {
// do something with suit
print(suit.rawValue)
}
But, wait! When you try to compile this, you'll encounter a compiler error that says, "Enum case 'Suit' not found in type 'Suit.Type'". Oh no, what went wrong? 😱
The Solution 🎉
The error occurs because Suit
itself is the type, and you cannot iterate over the type itself. 😕 However, we can easily solve this problem by using the allCases
property that is automatically generated for enums that conform to the CaseIterable
protocol. 🙌
To make Suit
conform to CaseIterable
, we just need to add CaseIterable
to the enum declaration, like this:
enum Suit: String, CaseIterable { // 🎉 Added CaseIterable here! 🎉
case spades = "♠️"
case hearts = "♥️"
case diamonds = "♦️"
case clubs = "♣️"
}
Now that Suit
conforms to CaseIterable
, we can use the allCases
property to iterate over each case of the Suit
enum. 🎈
Here's the updated code snippet that does exactly that:
for suit in Suit.allCases { // 🎉 Using allCases property here! 🎉
// do something with suit
print(suit.rawValue)
}
And voilà! 🎩 You will now see the expected result in your console:
♠️
♥️
♦️
♣️
Wrapping Up 🎁
Enumerating through an enum with string type in Swift is a common problem, but with the CaseIterable
protocol and the allCases
property, it becomes an easy task. 🚀
Now that you know this handy solution, you can confidently iterate over string-based enums like a pro! 😎
But wait, let's take a moment to connect! 😊 Share your experience with enumerating string enums in the comments below. Have you encountered any challenges, or do you have alternative approaches to share? Let's learn from each other! 💬👇
Happy coding! 💻💙