How does String substring work in Swift
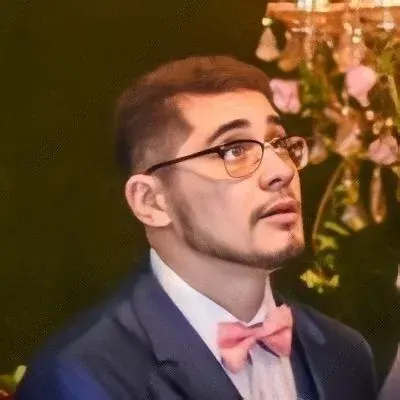
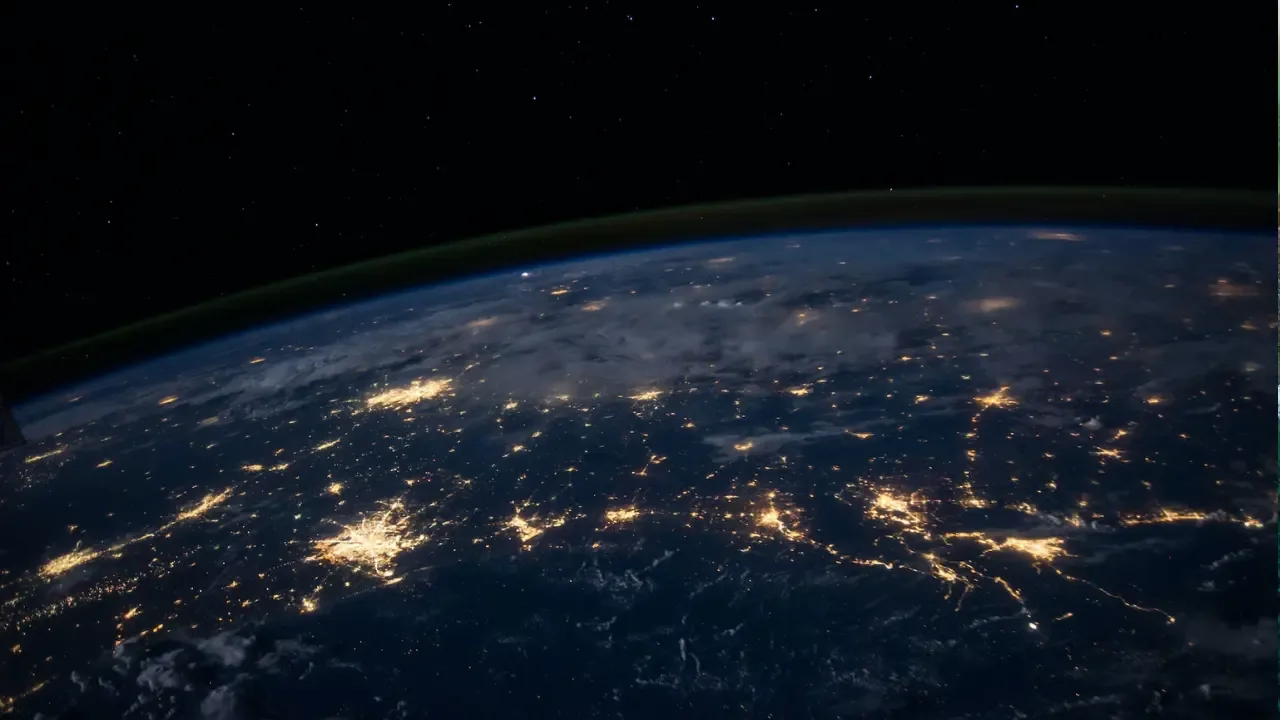
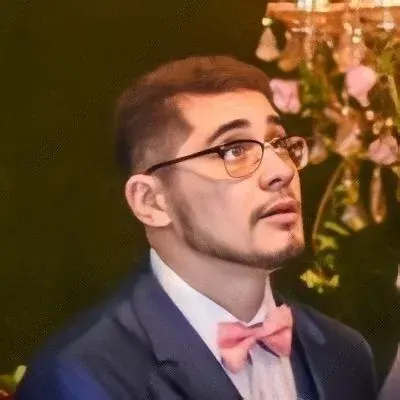
How does String substring work in Swift? 🤔
If you're familiar with Swift programming language and have been updating your code to Swift 3, you might have encountered some confusion when it comes to working with substrings. Specifically, the introduction of new methods for string indexing can be a little overwhelming.
Let's take a look at a specific issue that a developer faced, and then we'll dive into the solutions and provide a comprehensive guide on how String substring works in Swift.
The Problem 🚫
The developer attempted the following code snippet:
let str = "Hello, playground"
let prefixRange = str.startIndex..<str.startIndex.advancedBy(5)
let prefix = str.substringWithRange(prefixRange)
However, an error occurred on the second line:
Value of type 'String' has no member 'substringWithRange'
This error message implies that the substringWithRange
method is no longer available for the String
type. So what happened? 🤔
The Solution 💡
In Swift 3, the substringWithRange
method is deprecated and replaced with three new methods:
substring(to: String.Index)
substring(from: String.Index)
substring(with: Range<String.Index>)
Here's how they work:
1. substring(to: String.Index)
✂️
This method allows you to extract a substring from the beginning of your original string up to a specified index. For example:
let str = "Hello, playground"
let endIndex = str.index(str.startIndex, offsetBy: 5)
let prefix = str.substring(to: endIndex)
print(prefix) // Output: "Hello"
2. substring(from: String.Index)
✂️
With this method, you can extract a substring starting from a specified index to the end of your original string. Here's an example:
let str = "Hello, playground"
let startIndex = str.index(str.startIndex, offsetBy: 7)
let suffix = str.substring(from: startIndex)
print(suffix) // Output: "playground"
3. substring(with: Range<String.Index>)
✂️
This method allows you to extract a substring using a range of indices. The range can span from the beginning to the end of your original string or any specific section within it. Here's an example:
let str = "Hello, playground"
let range = str.index(str.startIndex, offsetBy: 7)..<str.endIndex
let substring = str.substring(with: range)
print(substring) // Output: "playground"
A Comprehensive Guide to Working with Substrings 📚
To use the substring
methods effectively, it's necessary to understand how Swift handles indexing and ranges. Here's a quick overview:
String.Index represents a position within a string. It's important to note that not every integer value can be a valid index due to Swift's Unicode correctness.
Range<String.Index> is a range of indices that define a section of a string.
You can obtain a valid index by using the
index(_:offsetBy:)
method. The first parameter is the starting index, and the second parameter is the offset.Additionally, you can use the
startIndex
andendIndex
properties of the string to refer to the beginning and end of the string, respectively.
By understanding these concepts, you can leverage the substring methods to manipulate strings more effectively.
Conclusion 🎉
Working with substrings in Swift can be a bit tricky, especially when migrating from older versions. However, by embracing the new methods and understanding how indices and ranges work, you'll be able to extract the desired sections of your strings confidently.
If you found this guide helpful, be sure to share it with your fellow Swift developers who might also be grappling with string manipulation. Happy coding! 💻✨
Still confused? Have any questions about string substrings in Swift? Feel free to leave a comment below, and I'll be more than happy to help you out! 😊