How do you use String.substringWithRange? (or, how do Ranges work in Swift?)
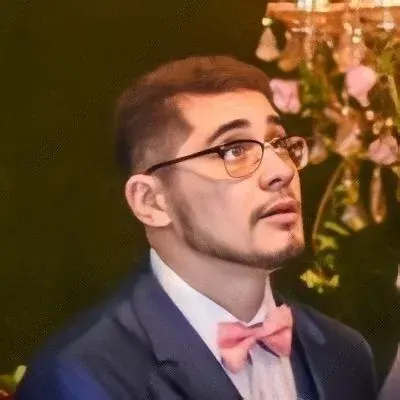
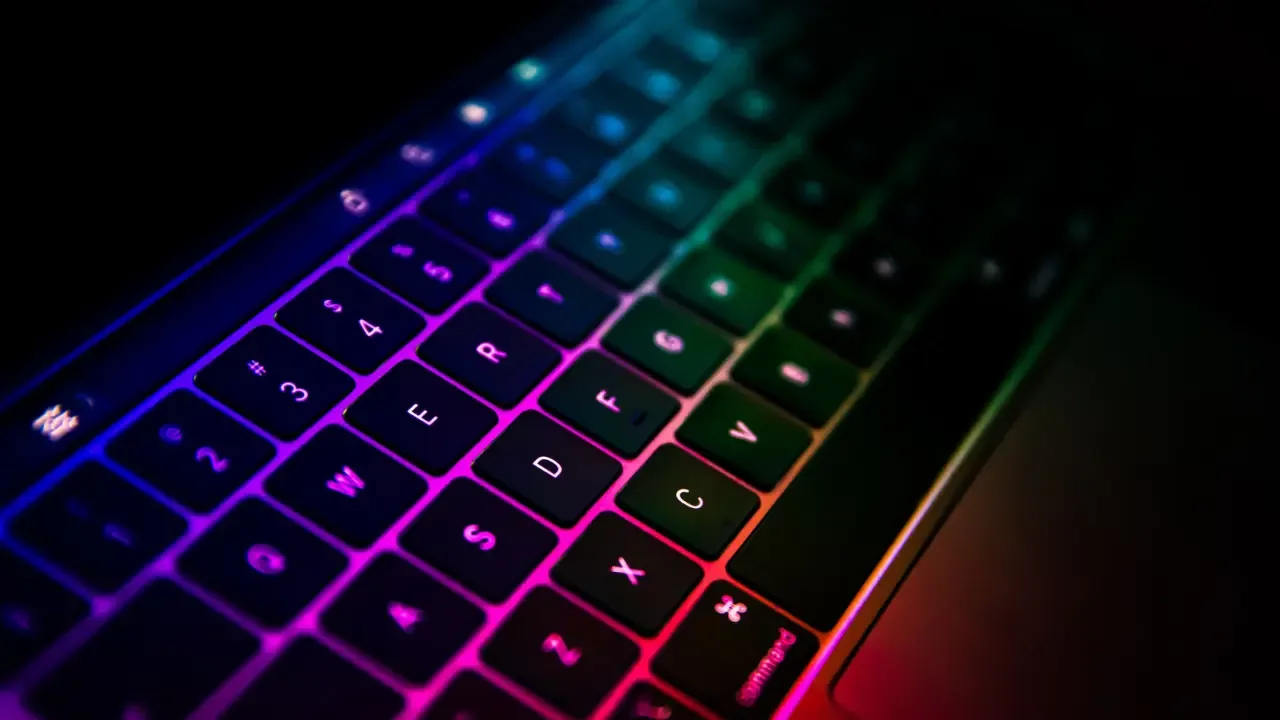
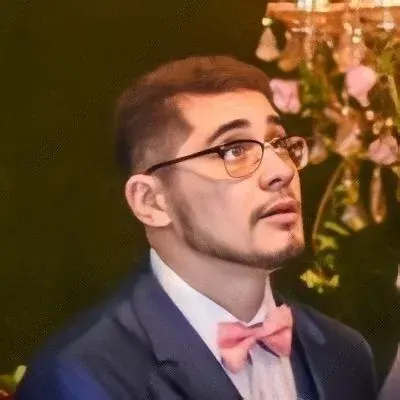
How Do You Use String.substringWithRange? (or, How Do Ranges Work in Swift?)
Have you ever tried to get a substring from a String
in Swift and ended up frustrated and confused? Don't worry, you're not alone. The String.substringWithRange
method and Range concept in Swift can be tricky to grasp initially. But fear not, we're here to help you demystify it and provide easy solutions to your problems.
The Challenge with Range Syntax
Let's start by addressing the specific issue mentioned in the context. You were attempting to use the String.substringWithRange
method but struggled with the correct syntax for creating a Range
. Autocomplete in the Playground may not always provide the most helpful suggestions, leaving you scratching your head.
In your attempts, you used variations such as:
// Attempt 1
str.substringWithRange( /* What goes here? */ )
// Attempt 2
str.substringWithRange(aRange: Range<String.Index>)
// Attempt 3
str.substringWithRange(Range(0, 1))
// Attempt 4
let r: Range<String.Index> = Range<String.Index>(start: 0, end: 2)
str.substringWithRange(r)
These attempts show your determination to find the correct syntax, but unfortunately, they are not the right solutions.
Understanding Range Syntax
To use String.substringWithRange
or work with ranges in Swift, you need to understand the syntax for creating ranges. A range represents a portion or subset of a collection. In our case, we want a range of indices to extract a substring from a String
.
The correct syntax for creating a range is:
let range = startIndex..<endIndex
Here, startIndex
represents the first index of the collection, and endIndex
represents one past the last index. Using this syntax, we can create a range to indicate the portion of the string we want to extract.
Solving the Substring Challenge
Now that we understand the range syntax, let's solve the original challenge and extract substrings from a String
.
var str = "Hello, playground"
let startIndex = str.startIndex
let endIndex = str.index(startIndex, offsetBy: 5)
let substring = str[startIndex..<endIndex]
print(substring) // Output: Hello
In this example, we first retrieve the startIndex
of the string using the startIndex
property. Then, we calculate the endIndex
by using the index(_:offsetBy:)
method with an offset of 5, indicating that we want a substring of length 5. Finally, we use the range syntax to extract the desired substring from the String
.
Bridging with NSString
If you've come across answers mentioning the use of NSString
methods, you may wonder if they can be applied to String
as well. Since Swift's String
bridges to NSString
, some old methods can still be used. Here's an example:
let x = (str as NSString).substring(with: NSMakeRange(0, 5))
print(x) // Output: Hello
In this case, we're casting the String
as an NSString
using the as
keyword and then calling the substring(with:)
method. We pass in a NSRange
created using the NSMakeRange
function.
Engage in the Swift String Conversation
Now that you've learned how to use String.substringWithRange
and explored range syntax in Swift, it's time to engage with the Swift community and share your thoughts.
Have you encountered any challenges or interesting use cases involving strings and ranges in Swift? Share your experiences or ask questions in the comments below. Let's learn together!
Don't forget to subscribe to our newsletter or follow us on social media to stay updated with the latest Swift tips, tricks, and tutorials.
Remember, range syntax may initially appear confusing, but with practice and understanding, it becomes a powerful tool in your Swift programming arsenal. Happy coding! ✨💻🚀