How do I print the type or class of a variable in Swift?
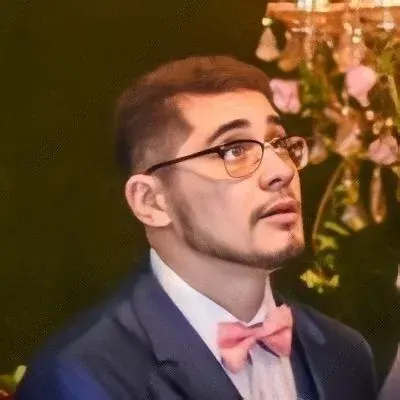
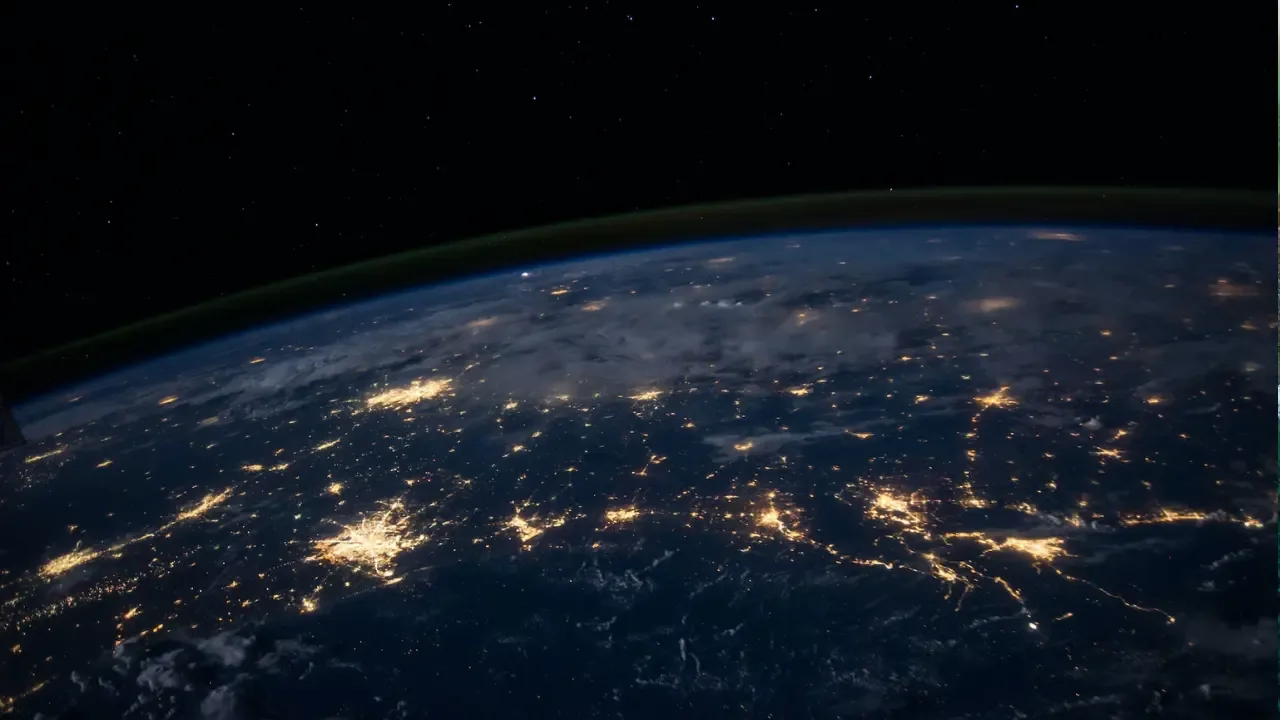
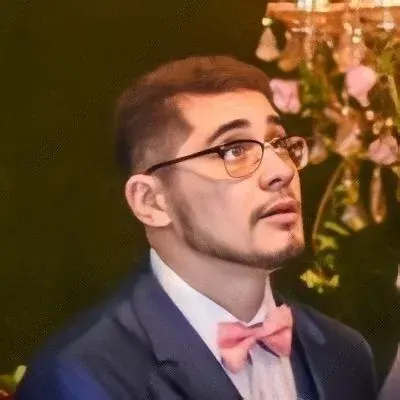
🖨️🔍🤔How do I print the type or class of a variable in Swift?🤔🔍🖨️
Have you ever found yourself scratching your head and wondering how to print the type or class of a variable in Swift? 🤔 It may seem like a simple task, but it can be surprisingly tricky. Luckily, I'm here to help you solve this problem in a snap! 💪💡
So, let's dive right in! Here's an example of the kind of code you might be working with:
var now = Date()
var soon = now.addingTimeInterval(5.0)
print("\(type(of: now))")
// Prints "Date"
print("\(type(of: soon))")
// Prints "Date"
print("\(type(of: soon))?")
// Prints "Optional<Date>"
print("\(soon as Any)")
// Prints "Optional(2022-10-20 10:00:00 +0000)"
print("\(soon as! Date)")
// Prints "2022-10-20 10:00:00 +0000"
In the example above, we have two variables: now
and soon
. We want to know their precise types when debugging or for any other purpose. Let's explore a few solutions together!
🔍 Solution 1: Using type(of:)
The simplest and most straightforward way to print the type of a variable is to use the type(of:)
function. This will return the type of the variable as a Type
object. Just pass your variable as an argument to type(of:)
and print the result. Easy peasy! 😎
📝 Example usage:
print("\(type(of: now))")
// Output: "Date"
🔍 Solution 2: Using as Any
Another useful technique is using the as Any
cast. This casts your variable as the universal Any
type, allowing you to print it without explicitly specifying the type. This can come in handy when you're not sure about the exact type of your variable. 💡
📝 Example usage:
print("\(soon as Any)")
// Output: "Optional(2022-10-20 10:00:00 +0000)"
🔍 Solution 3: Using Optional
type notation
If you're working with optional types, you might want to print both the base type and the optional type. To do this, use type(of:)
in combination with the ?
operator. This will give you the type of the variable followed by a question mark, indicating it's an optional type. 🙌
📝 Example usage:
print("\(type(of: soon))?")
// Output: "Optional<Date>"
🚀 Now that you know how to print the type or class of a variable in Swift, you can debug your code more effectively and understand your data with ease! So go ahead and try out these solutions, and let me know in the comments which one worked best for you! 💬💪
Remember, understanding the type of your variables can be a game-changer when it comes to debugging and writing clean, error-free code. So don't forget to make use of these techniques in your future Swift projects! 🚀👩💻
And if you found this blog post helpful, don't be shy – share it with your fellow Swift developers! Let's spread the knowledge and make everyone's coding lives a little bit easier. 😉📢
Happy coding! 🎉🚀
— Your friendly neighborhood tech writer