How do I get a reference to the AppDelegate in Swift?
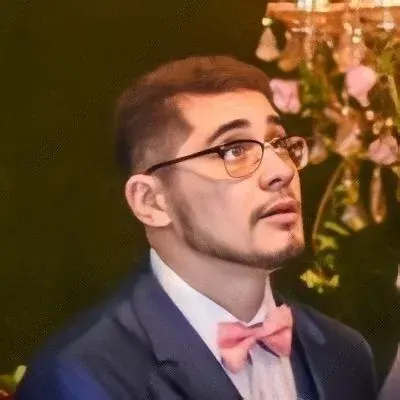
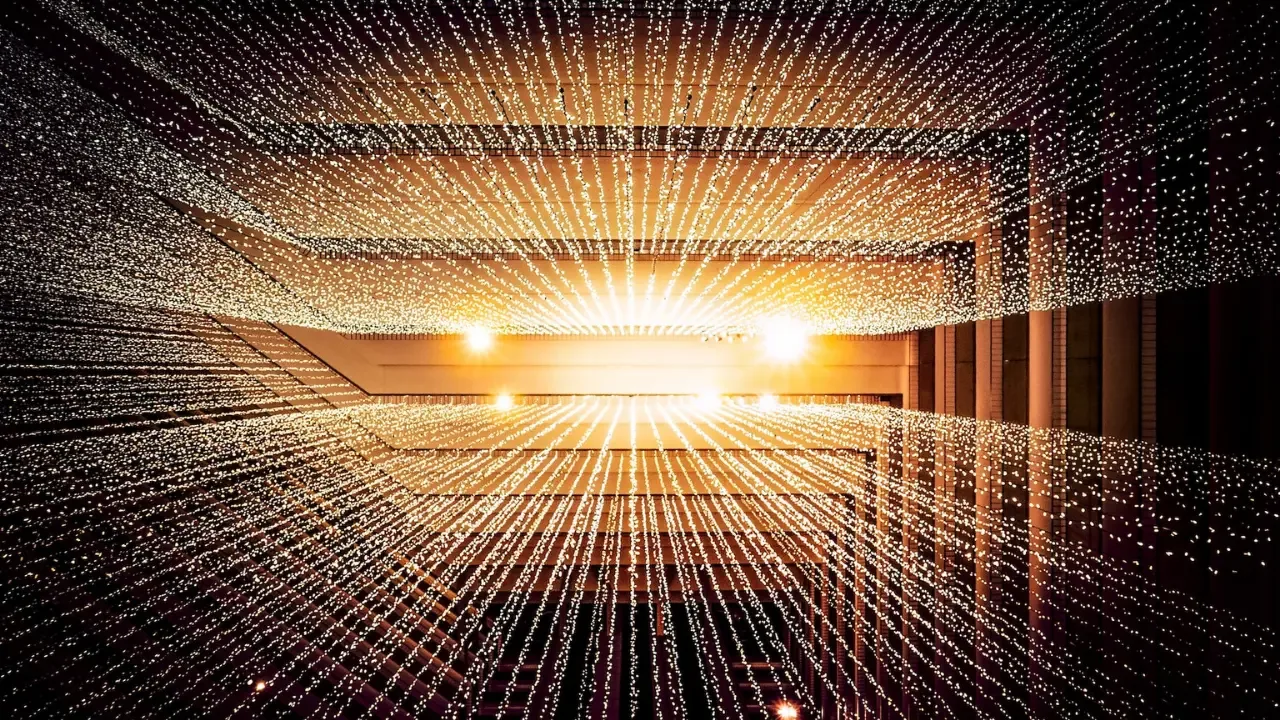
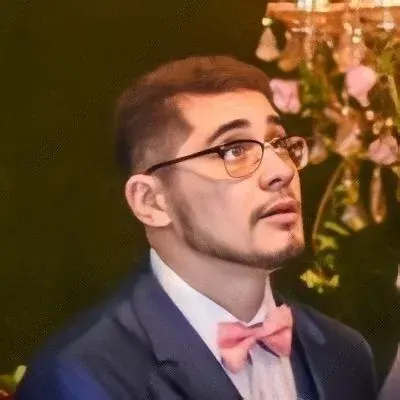
📱 How to Get a Reference to AppDelegate in Swift?
So, you want to get a reference to the AppDelegate
in Swift, huh? No worries! I got you covered. 😎 In this guide, I will show you how to easily get that reference and access the managed object context. Let's dive right in! 🏊♂️
Understanding the AppDelegate
Before we jump into the solution, let's quickly discuss what the AppDelegate
actually is. In iOS development, the AppDelegate
is a crucial class that acts as the entry point for your app. It handles important app-level events and provides a central place for managing app-wide functionality. 🚀
Common Solution
To get a reference to the AppDelegate
in Swift, you can use the UIApplication.shared.delegate
property. This property returns an object that conforms to the UIApplicationDelegate
protocol, which is typically your AppDelegate
class. Here's how you do it:
if let appDelegate = UIApplication.shared.delegate as? AppDelegate {
// Access the managed object context
let managedObjectContext = appDelegate.persistentContainer.viewContext
// Do whatever you need to do with the managed object context
} else {
// Unable to get AppDelegate reference
// Handle the error gracefully
}
In the above example, we first attempt to retrieve the shared
instance of UIApplication
, which represents the current app. Then, we cast the delegate
property to the AppDelegate
class using the as?
operator. If the cast is successful, we can access the AppDelegate
instance and perform any necessary operations, such as accessing the managed object context. 📚
Dealing with Errors
It's important to handle potential errors when attempting to get the AppDelegate
reference. If the cast fails, it means something went wrong, and we couldn't retrieve the reference. In such cases, you should handle the error gracefully, ensuring a smooth user experience.
Conclusion
And that's it! Now you know how to get a reference to the AppDelegate
in Swift and access the managed object context. You're one step closer to creating amazing iOS apps! 🥳
Go ahead and give it a try in your own project. If you have any questions, feel free to leave a comment below. Happy coding! 💻✨
👉 Check out our other tech tutorials
📣 Let's connect on Twitter and Instagram for more tech goodness!