How do I dispatch_sync, dispatch_async, dispatch_after, etc in Swift 3, Swift 4, and beyond?
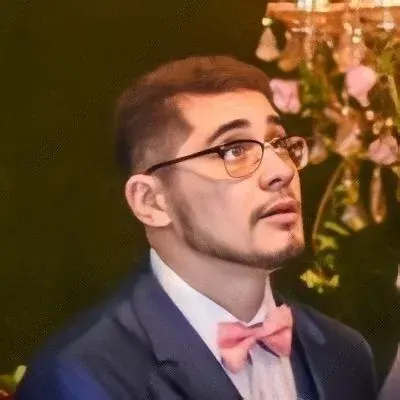
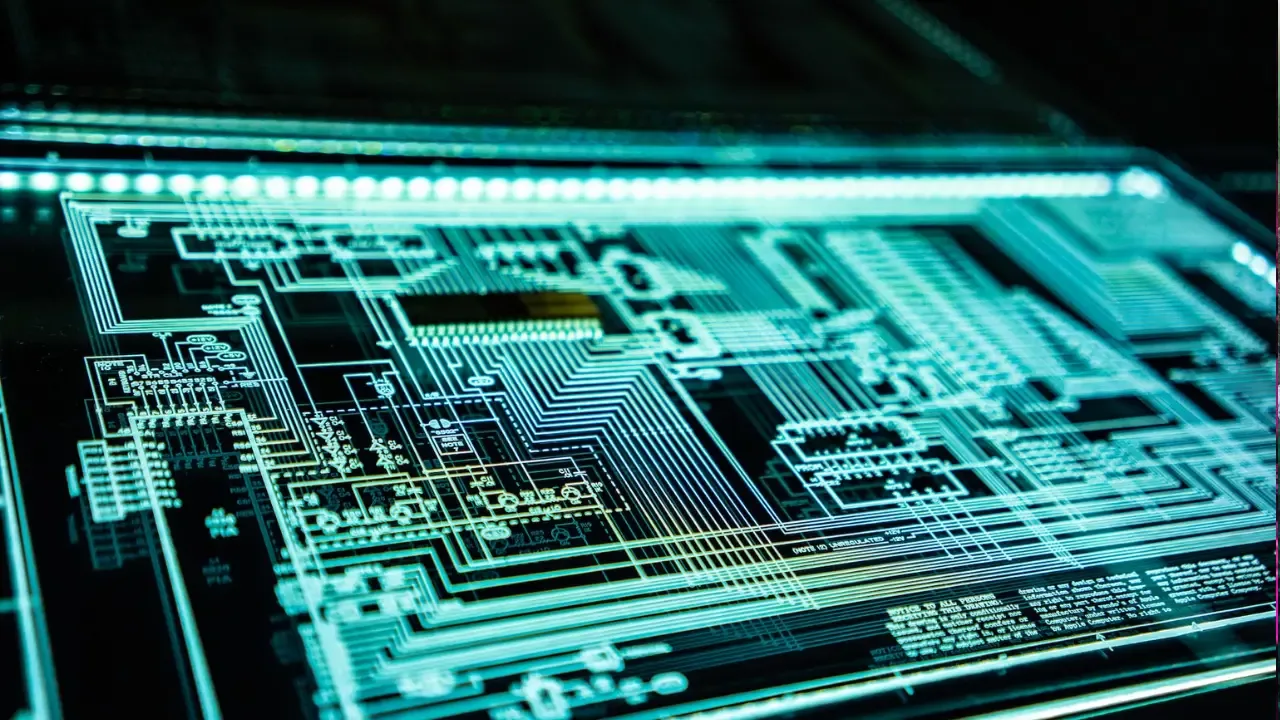
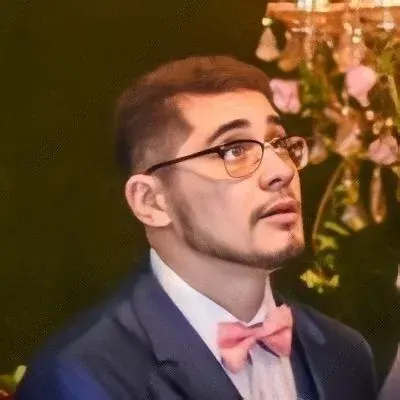
š Dispatching with Ease in Swift 3, Swift 4, and Beyond! š
š Hey there, fellow Swift developers! Are you struggling with migrating your code from Swift 2.x to the mighty Swift 3 and beyond? Are you facing errors and issues with dispatching tasks using the Grand Central Dispatch (GCD) API? Well, worry no more! In this blog post, I'm going to guide you through the process of upgrading your dispatch operations and show you some easy solutions to common problems. Let's dive right in! šŖ
š From dispatch_async to dispatch(queue:execute:)
In Swift 3 and onwards, Apple introduced a more Swift-like syntax for dispatching tasks. The old dispatch_async
method has been replaced with the new dispatch(queue:execute:)
method. To migrate your existing code, you can simply follow this pattern:
DispatchQueue.global(qos: .default).async {
// Perform your long running work here
let image = self.loadOrGenerateAnImage()
DispatchQueue.main.async {
// Update UI on the main thread
self.imageView.image = image
}
}
š§ dispatch_after is now DispatchWorkItem
Remember the old dispatch_after
method? In Swift 3, it has been replaced with DispatchWorkItem
. Here's how you can achieve the same delay execution effect:
let delayInSeconds: TimeInterval = 0.5
let dispatchTime = DispatchTime.now() + delayInSeconds
let workItem = DispatchWorkItem {
print("test")
}
DispatchQueue.main.asyncAfter(deadline: dispatchTime, execute: workItem)
š Handling Common Errors and Issues
1ļøā£ Error: "Cannot invoke 'async' with an argument list of type '(dispatch_time_t, dispatch_queue_t, () -> Void)'"
This error occurs because the arguments of dispatch_after
have changed in Swift 3. To fix this, replace the old code with the new Dispatch API, as shown in the previous example.
2ļøā£ Error: "Task may not be executed concurrently"
Swift 3 introduced stricter thread safety rules. If you encounter this error, it means you are trying to execute a task concurrently on the same queue. To resolve this, ensure that your tasks are dispatched on separate queues or use appropriate synchronization techniques like semaphores or barriers.
3ļøā£ Error: "Value of type 'DispatchQueue.GlobalQueuePriority' has no member 'Default'"
In Swift 3, the DISPATCH_QUEUE_PRIORITY_DEFAULT
constant has been replaced by the DispatchQoS.default
enum case. Simply update your code with the new constant, and you're good to go!
4ļøā£ Error: "Cannot convert value of type '(void) -> void' to expected argument type 'dispatch_block_t!'"
This error occurs when you pass nil
as the completion block for dispatch_async
. In Swift 3, completion blocks are now optional, so you can easily fix this by removing the completion block parameter if it's unnecessary.
š Your Call to Actions!
Congratulations! You've learned how to migrate your dispatch operations to Swift 3 and beyond. Now it's time to put your newfound knowledge into action! Update your code using the provided solutions, and feel the power of the latest Swift versions. š
1ļøā£ Share your success story or ask questions in the comments section below. Let's build a supportive community to help each other grow!
2ļøā£ If you found this blog post helpful, give it a like and share it with your fellow Swift enthusiasts. Let's spread the knowledge! š
3ļøā£ Stay tuned for more exciting tech content by subscribing to our newsletter. Don't miss the chance to level up your Swift skills! š§
That's a wrap, folks! Happy dispatching, and may your code flow smoothly like a well-synchronized dance! ššŗ