How do I check if a string contains another string in Swift?
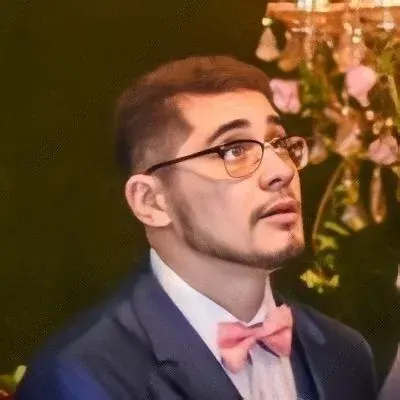
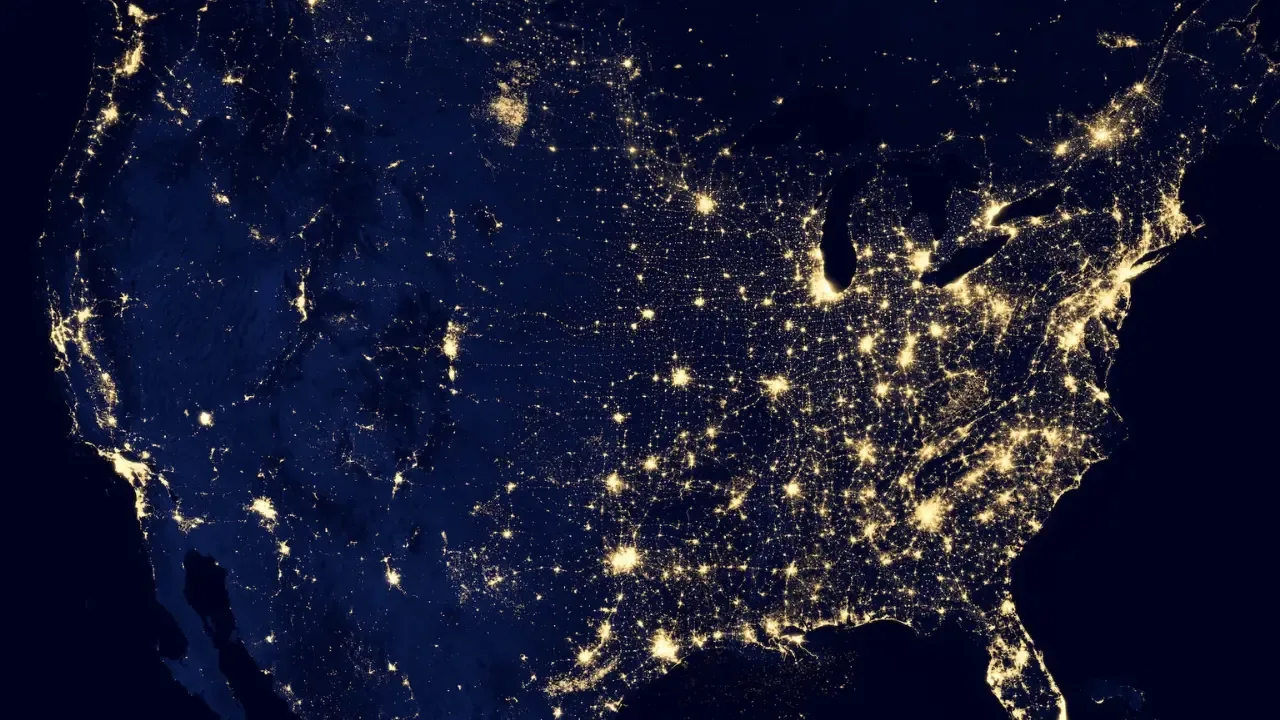
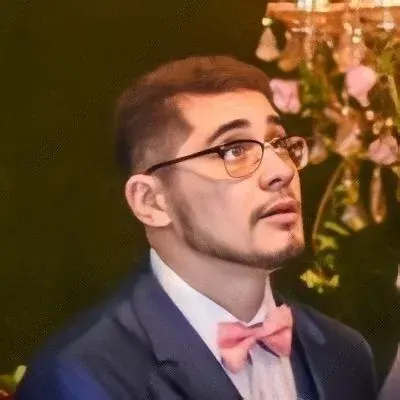
Checking if a String Contains Another String in Swift: A Comprehensive Guide! 🚀
Are you starting your Swift journey and wondering how to check if a string contains another string? Fear not, fellow Swift explorer! In this blog post, we'll walk through common issues and provide easy solutions to help you level up your Swift string manipulation game. Let's dive right in! 💪
The Objective-C Way
Before we venture into Swift, let's take a quick look at how it's done in Objective-C. In Objective-C, you can use the rangeOfString:
method of the NSString
class to check if a substring exists within a string. The code snippet you provided demonstrates this approach.
NSString *string = @"hello Swift";
NSRange textRange =[string rangeOfString:@"Swift"];
if(textRange.location != NSNotFound)
{
NSLog(@"exists");
}
Fetching Substrings in Swift! 🧲
Now, let's unleash the power of Swift and discover how easy it is to check if a string contains another string using Swift's string manipulation capabilities.
Solution 1: The contains
Method 🕵️♀️
Swift provides a handy method called contains
which allows you to check if a string contains another string. Let's see it in action:
let string = "hello Swift"
if string.contains("Swift") {
print("Exists")
}
In this example, we use the contains
method on the string
object and pass the substring we want to check for, which is "Swift" in this case. If the substring is found within the string, the code inside the if
block gets executed. Easy, right? 😉
Solution 2: The range(of:)
Method 🔍
If you need more control or want to access additional information about the range of the substring within the string, Swift provides the range(of:)
method. Here's how you can use it:
let string = "hello Swift"
if let textRange = string.range(of: "Swift") {
print("Exists")
}
The range(of:)
method returns an optional Range
object that represents the range of the substring within the string. If the substring is found, it returns a valid range; otherwise, it returns nil
. By using the optional binding with if let
, we ensure that we only execute the code inside the if
block when the substring is found.
Call to Adventure! 🏔️
Now that you have mastered the art of checking if a string contains another string in Swift, it's time to put your newfound knowledge to use! Try incorporating these techniques into your own projects or check out more Swift string manipulation methods. The possibilities are endless! 🎉
If you found this guide helpful, don't forget to share it with your fellow Swift enthusiasts and leave a comment below sharing your thoughts and experiences. Let's ignite a lively conversation! 🔥
Happy coding! 👩💻👨💻