How can I use String substring in Swift 4? "substring(to:)" is deprecated: Please use String slicing subscript with a "partial range from" operator
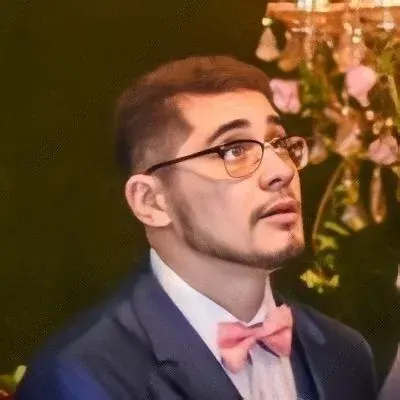
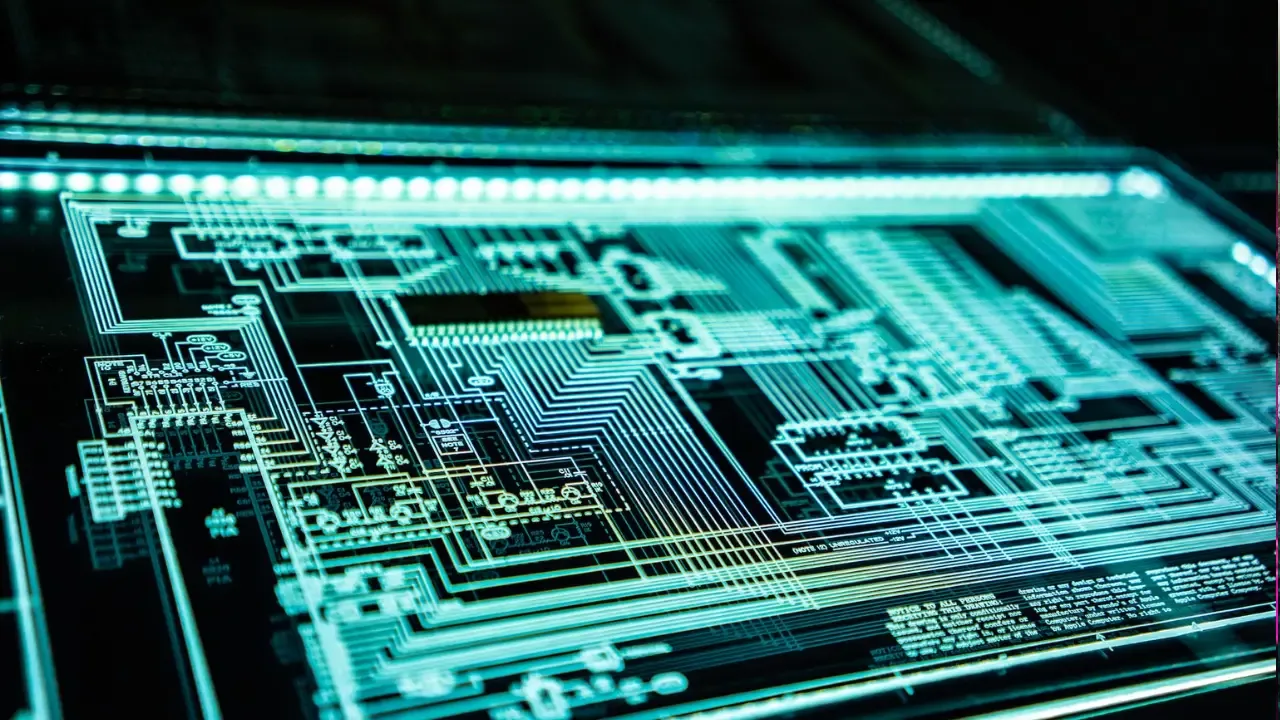
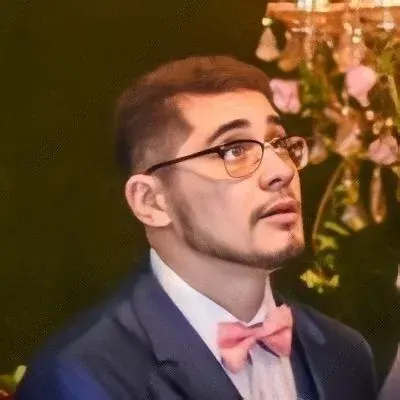
How to Use String Slicing Subscript with Partial Range From in Swift 4 😎
So you want to use the substring(to:)
method in Swift 4, but you're getting a deprecation warning? No worries! Swift 4 has a cool alternative for you called the String Slicing Subscript with Partial Range From. 🚀
Let's dive in and see how you can replace the deprecated method with the new slicing subscript. 💪
Understanding the Problem 🤔
The deprecation warning states that the substring(to:)
method is deprecated in Swift 4. This means that you need to find an alternative method to achieve the same functionality.
The Old Way ⏳
In Swift 3, you might have used the following code to get a substring up to a specified index:
let str = "Hello, playground"
let index = str.index(of: ",")!
let newStr = str.substring(to: index)
This code is simple and effective, but it triggers the deprecation warning in Swift 4.
The New Way 🆕
To achieve the same result in Swift 4, you can use the String Slicing Subscript with Partial Range From. 🥳
Here's how you can do it:
let str = "Hello, playground"
let index = str.index(of: ",") ?? str.endIndex
let newStr = str[..<index]
In the new code, we first set the index
variable to either the index of the comma or to the end index of the string if no comma is found. Then, we use the slicing subscript with the partial range from operator ..<
to get the substring from the start of the string up to the index
.
By using this new approach, you can achieve the same functionality without triggering any deprecation warnings. 🎉
Apply What You've Learned 🤓
Now that you know how to use the String Slicing Subscript with Partial Range From, go ahead and update your code to utilize this cool new feature. You'll have cleaner code and stay up to date with the latest improvements in Swift.
Conclusion 🌟
In this blog post, we explored the deprecation of the substring(to:)
method in Swift 4 and learned how to use the String Slicing Subscript with Partial Range From as a replacement. Now it's time for you to start using this new feature in your own code!
Do you have any questions or faced any issues while implementing this change? Feel free to share your thoughts and experiences in the comments below. Let's engage in a discussion about Swift 4 and its awesome features! 😃✨