How can I make a weak protocol reference in "pure" Swift (without @objc)
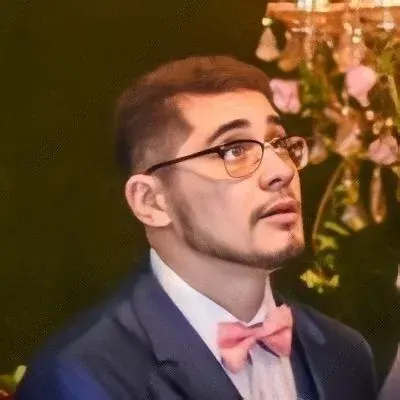
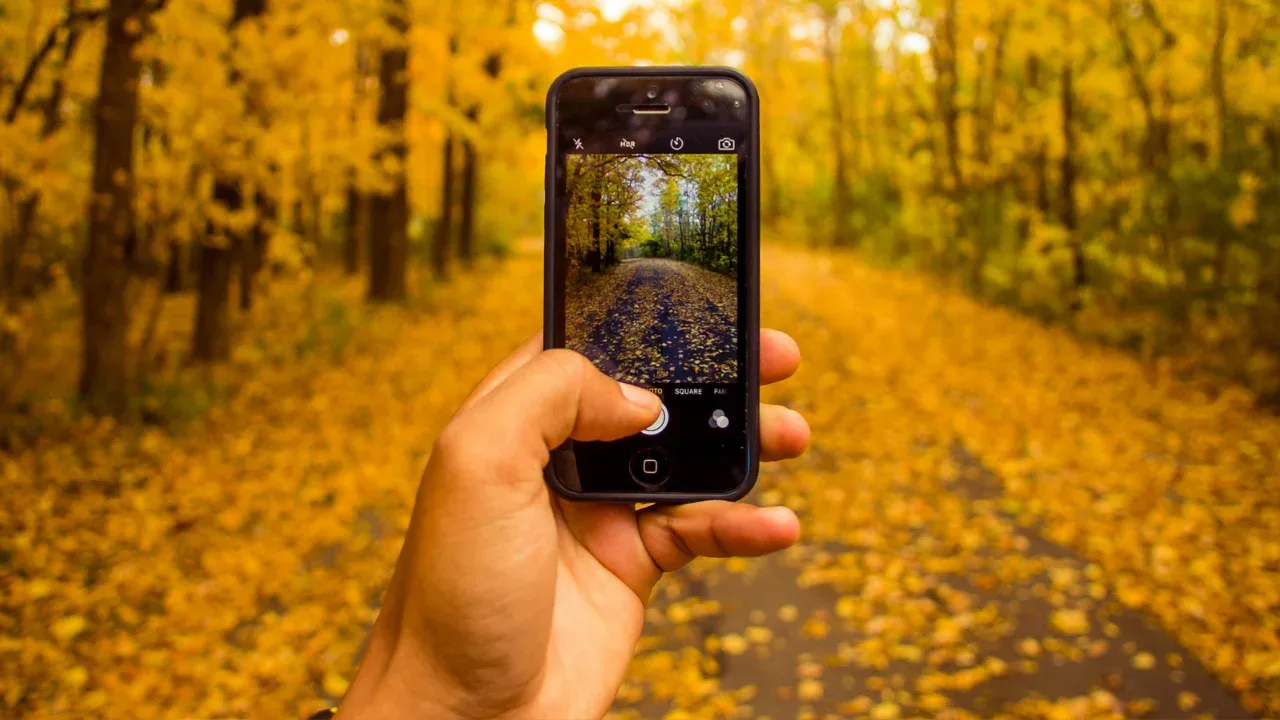
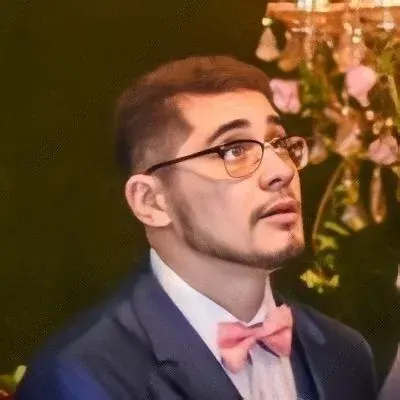
Making a Weak Protocol Reference in 'Pure' Swift: The Definitive Guide
Are you tired of dealing with strong reference cycles in your Swift code? Wondering how to make a weak protocol reference without resorting to the @objc
attribute? You've come to the right place! In this blog post, we'll explore the ins and outs of creating a weak protocol reference in 'pure' Swift.
The Problem: Weak References and Non-Class Types
Let's start by addressing the common issue at hand. You may have noticed that weak references don't work in Swift when a protocol is not declared with the @objc
attribute. This limitation can be frustrating, especially if you're building a purely Swift app.
Consider the following code snippet:
class MyClass {
weak var delegate: MyClassDelegate?
}
protocol MyClassDelegate {
}
This seemingly innocent code triggers a compile error: "weak cannot be applied to non-class type MyClassDelegate." What gives?
The Solution: A Pure Swift Approach
Fortunately, we can still achieve a weak delegate reference in 'pure' Swift without relying on the @objc
attribute. Here's how:
Add the
AnyObject
Protocol Requirement: Modify your protocol declaration by addingAnyObject
as a requirement. This indicates that the protocol can only be adopted by class types:
protocol MyClassDelegate: AnyObject {
}
Declare the Delegate Property as Weak: With the
AnyObject
requirement in place, you can now declare your delegate property as weak without any compiler errors:
class MyClass {
weak var delegate: MyClassDelegate?
}
Voila! You now have a weak delegate reference in 'pure' Swift, successfully avoiding the usage of @objc
.
Example: Implementing a Weak Delegate
To better illustrate this solution, let's create a simple example. Imagine you're building a photo editing app, and you want to implement a weak delegate pattern for notifying the parent view controller when a photo editing operation is completed.
First, define your delegate protocol with the AnyObject
requirement:
protocol PhotoEditorDelegate: AnyObject {
func didFinishEditingPhoto()
}
Next, declare a weak delegate property in your PhotoEditor
class:
class PhotoEditor {
weak var delegate: PhotoEditorDelegate?
func finishEditing() {
// Editing logic here
delegate?.didFinishEditingPhoto()
}
}
Whenever the editing process is complete, you can call the delegate method without worrying about strong reference cycles. Just make sure to handle the optional delegate properly.
Call to Action: Join the 'Pure' Swift Revolution!
Now that you've learned how to make a weak protocol reference in 'pure' Swift, it's time to put it into practice! Start adopting this approach in your codebase and embrace the power of Swift without compromising maintainability.
Have you encountered any other Swift conundrums? Share your experiences and questions in the comments below. Let's engage in a lively discussion and learn from each other!
Remember, Swift is a language that evolves continuously, so stay curious and keep exploring! 🚀
P.S. Don't forget to share this post with your fellow Swift enthusiasts. Together, we can conquer any coding challenge!