How can I make a button have a rounded border in Swift?
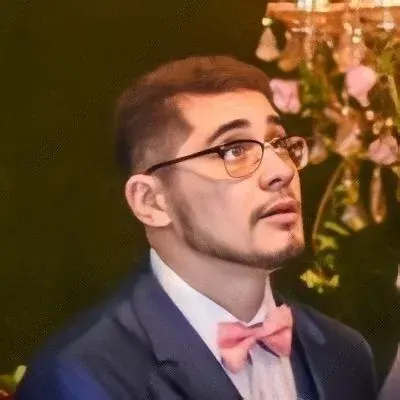
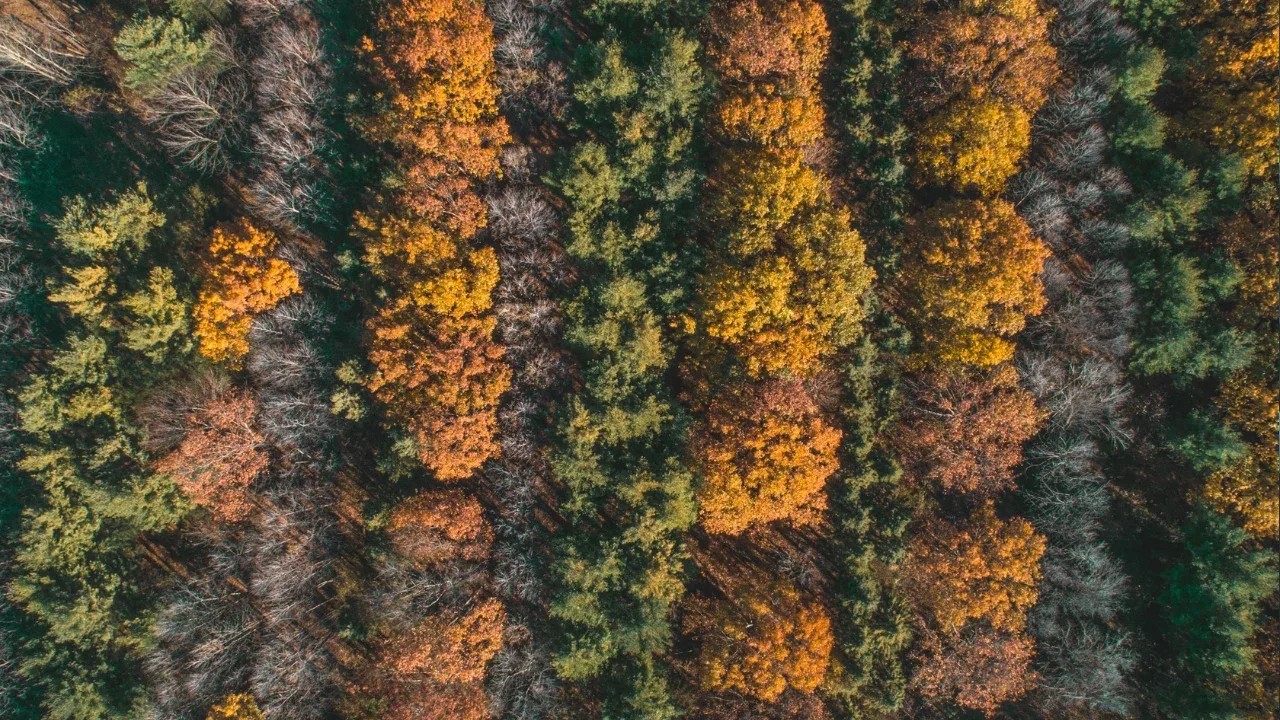
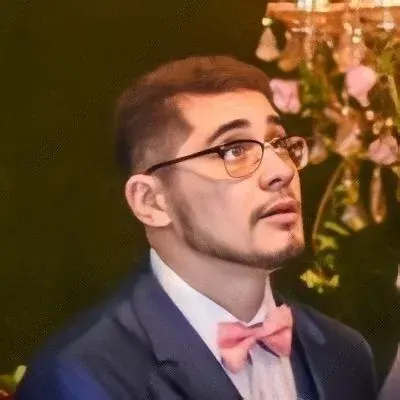
How to Make a Button Have a Rounded Border in Swift
If you're building an app using Swift and Xcode, you may have encountered the challenge of creating a button with a rounded border. In this guide, we will walk you through the steps to achieve this effect and even customize the border color. Let's dive in!
The Problem
The original question asked how to create a button with a slightly rounded border and no background color. This can be a bit tricky for newcomers to Swift, but fear not, we have the solution for you!
Step 1: Creating a Rounded Button
To make a button with a rounded border, you will need to modify its layer
property in Swift. Specifically, you will adjust the cornerRadius
property of the button's layer.
Here's an example of how you can achieve this:
let button = UIButton(type: .system)
button.frame = CGRect(x: 100, y: 100, width: 150, height: 50)
button.layer.cornerRadius = 10
button.layer.borderWidth = 1
In the above code snippet, we've created a button and set its cornerRadius
property to a value of 10. This will give the button its desired rounded border effect. Feel free to adjust the value of cornerRadius
to your liking.
Step 2: Changing the Border Color
Now that you have your rounded button, the second part of the question asks how to change the color of the border itself without adding a background color.
To accomplish this, you will make use of the borderColor
property of the button's layer. Here's an example:
button.layer.borderColor = UIColor.red.cgColor
In this example, we've set the borderColor
property to UIColor.red
to give the button a red border. You can easily change the color by replacing UIColor.red
with your desired color.
Step 3: Putting It All Together
To recap, here's how you can create a button with a rounded border and customize the border color:
let button = UIButton(type: .system)
button.frame = CGRect(x: 100, y: 100, width: 150, height: 50)
button.layer.cornerRadius = 10
button.layer.borderWidth = 1
button.layer.borderColor = UIColor.red.cgColor
With these steps, you can now create rounded buttons with different border colors to match your app's design and style.
Conclusion
Creating buttons with rounded borders can add a touch of elegance to your app's user interface. By using the cornerRadius
and borderColor
properties of a button's layer, you can easily achieve this effect. Don't be afraid to experiment with different values and colors to customize the look and feel of your buttons.
We hope you found this guide helpful in solving the challenge of making a button with a rounded border in Swift. If you have any questions or other topics you'd like us to cover, feel free to leave a comment below. Happy coding! 💻🚀