Get top most UIViewController
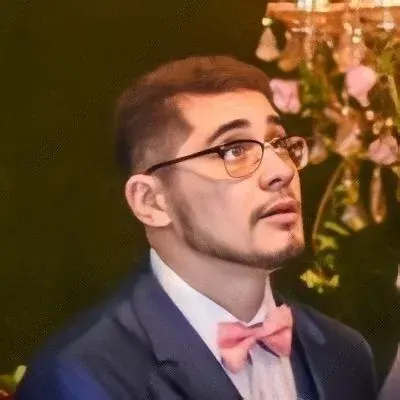
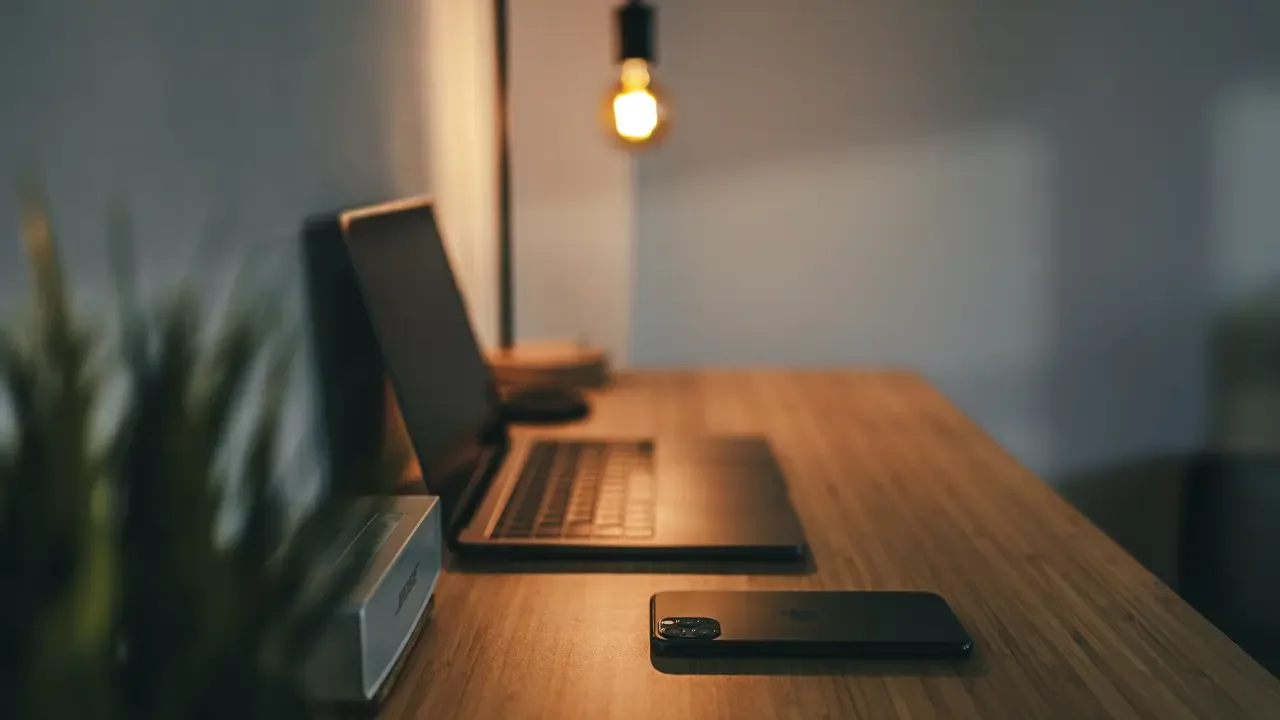
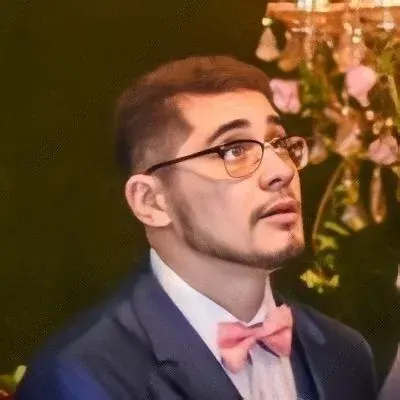
📚 Getting the Top Most UIViewController: Explained and Resolved! 💪
Have you ever struggled to get the top most UIViewController
without having access to a UINavigationController
? 🤔 Well, no worries! In this blog post, we're going to dive into this common issue and provide you with easy solutions. Let's buckle up and get started! 🚀
The Dilemma: Why Can't We Get the Top Most UIViewController? 🤷♀️🤷♂️
So, you attempted to fetch the top most UIViewController
using the following code:
UIApplication.sharedApplication().keyWindow?.rootViewController?.presentViewController(vc, animated: true, completion: nil)
But unfortunately, it didn't work! 😫 Even though the keyWindow
and rootViewController
have non-nil values, the expected result is not achieved. What could be the problem? Let's unravel this mystery together! 🕵️♀️🔍
Understanding the Issue: Breaking the MVC Pattern 🚫
Before we continue, it's crucial to understand that what we're trying to achieve by directly fetching the top most UIViewController
is actually considered a bad idea. 😮 Why, you may ask? Because it breaks the Model-View-Controller (MVC) pattern, which is a fundamental principle in iOS development.
In the MVC pattern, the UIViewController
is responsible for managing the presentation and interaction logic of the user interface. It should not have direct knowledge or control over other view controllers. By bypassing the standard flow, we risk introducing unexpected behaviors and making our codebase harder to maintain. So, proceed with caution! ⚠️
Solution: A Safer Approach! ✅
Now that you understand the underlying problem, let's explore a safer way to achieve what you need. Instead of directly accessing the top most UIViewController
, you can utilize proper navigation techniques and leverage the power of view controller containment. Here are some suggestions to consider:
1. Using a UINavigationController
If you have a UINavigationController
in your app's view hierarchy, you can take advantage of its stack to access the top most UIViewController
easily. Here's how you can do it:
if let topViewController = navigationController?.topViewController {
// Use the topViewController as needed
}
Keep in mind that this approach requires your view controller to be embedded within a navigation controller.
2. Implementing a Custom Delegate or Notification
Another approach is to implement a custom delegate or notification mechanism to communicate between view controllers. By doing this, you can pass necessary information or trigger actions at the appropriate times without explicitly accessing the top most UIViewController
.
3. Utilizing a Third-Party Library
If you find yourself frequently needing to access the top most UIViewController
across your app, you might consider using a well-established third-party library that provides a clean and supported solution. Some popular options include 'R.swift' and 'SwiftyStoryboard.'
Wrapping Up 🎁
As tempting as it may seem to directly access the top most UIViewController
, it's important to abide by the MVC pattern and use proper navigation techniques. We hope this blog post has shed some light on this common issue and provided you with alternative solutions. Remember, maintaining a clean and manageable codebase is essential for app development success! ✨
If you have any questions, comments, or alternative solutions, we would love to hear from you in the comments below. Sharing knowledge benefits the whole developer community! 🤝💡 So go ahead, let your voice be heard!
Until next time, happy coding! 💻🔥