Get nth character of a string in Swift
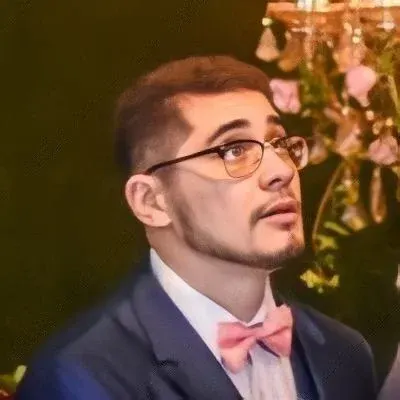
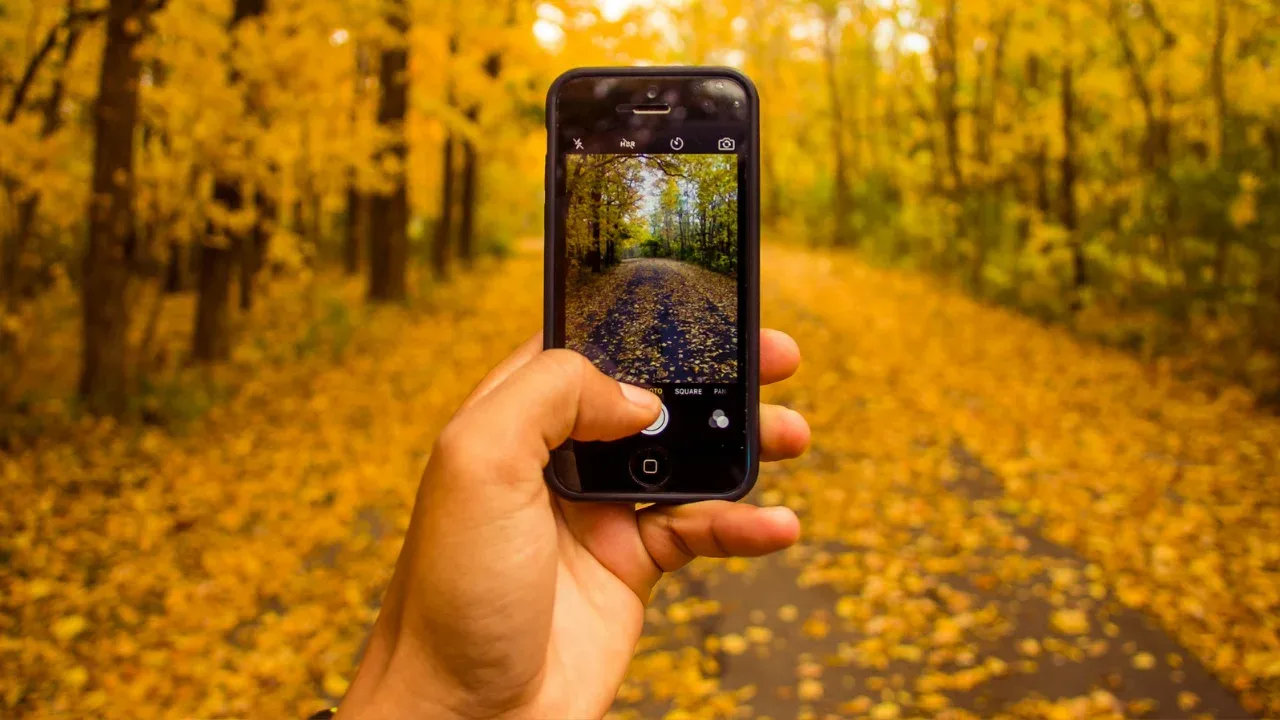
Get nth character of a string in Swift: A Complete Guide
Are you trying to get the nth character of a string in Swift, but encountering an error when using the bracket accessor? Don't worry, we've got you covered! In this blog post, we'll walk you through the common issues and provide easy solutions to help you get that desired character. 🎯
The Problem
Let's start by looking at the code example you provided:
var string = "Hello, world!"
var firstChar = string[0] // Throws error
When you try to access the character at position 0 using the bracket ([]
) accessor, Xcode throws an error with the following message:
ERROR: 'subscript' is unavailable: cannot subscript String with an Int, see the documentation comment for discussion
This error indicates that Swift does not allow direct subscripting of a string with an integer. However, worry not, as there are several easy solutions to obtain the nth character of a string.
Solution 1: String Indexing
One way to get the nth character of a string is by using string indexing. Here's an example:
var string = "Hello, world!"
let index = string.index(string.startIndex, offsetBy: 0)
let firstChar = string[index]
print(firstChar) // Output: "H"
In this solution, we use the index(_:offsetBy:)
method provided by Swift's String
class. We specify the starting index (string.startIndex
) and the offset (0 in this case) to get the desired character. Remember to adjust the offset based on zero-indexing in Swift.
Solution 2: Substring
Another approach is to utilize the substring(from:)
method to extract the desired character as a substring. Consider the following code:
var string = "Hello, world!"
let index = string.index(string.startIndex, offsetBy: 0)
let firstChar = string.substring(from: index)
print(firstChar) // Output: "H"
Here, we first obtain the index of the desired character as explained in Solution 1. Then, we use the substring(from:)
method to extract a substring starting from that index. This solution also returns the desired character.
Solution 3: Character Array
If you prefer working with an array, you can convert the string into an array of characters and access the nth element accordingly. Check out this example:
var string = "Hello, world!"
let charArray = Array(string)
let firstChar = charArray[0]
print(firstChar) // Output: "H"
In this solution, we convert the string into an array of characters using the Array(_:)
initializer. Then, we can access the nth character using the bracket accessor ([]
) with the appropriate index.
Conclusion
Getting the nth character of a string in Swift may initially seem challenging, but with the right approach, it's a piece of 🍰! We explored three easy solutions: string indexing, substring extraction, and conversion to a character array.
Remember to adjust the offset when working with string indices and be mindful of zero-indexing. If you encounter any errors or have further questions, feel free to leave a comment below. Happy coding! 🚀
<!-- Insert a compelling call-to-action here -->
Do you have any other Swift-related questions or topics you'd like us to cover in our blog? Share with us by leaving a comment, and we'll create a comprehensive guide just for you! Let's continue exploring the Swift universe together. 👩💻🧑💻
Stay tuned for more helpful Swift tips and guides! Don't forget to share this article with your fellow developers! 👨💻👩💻
(Note: The code provided here may not include extensive error handling or cover all edge cases. It is intended to demonstrate the solution conceptually.)
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
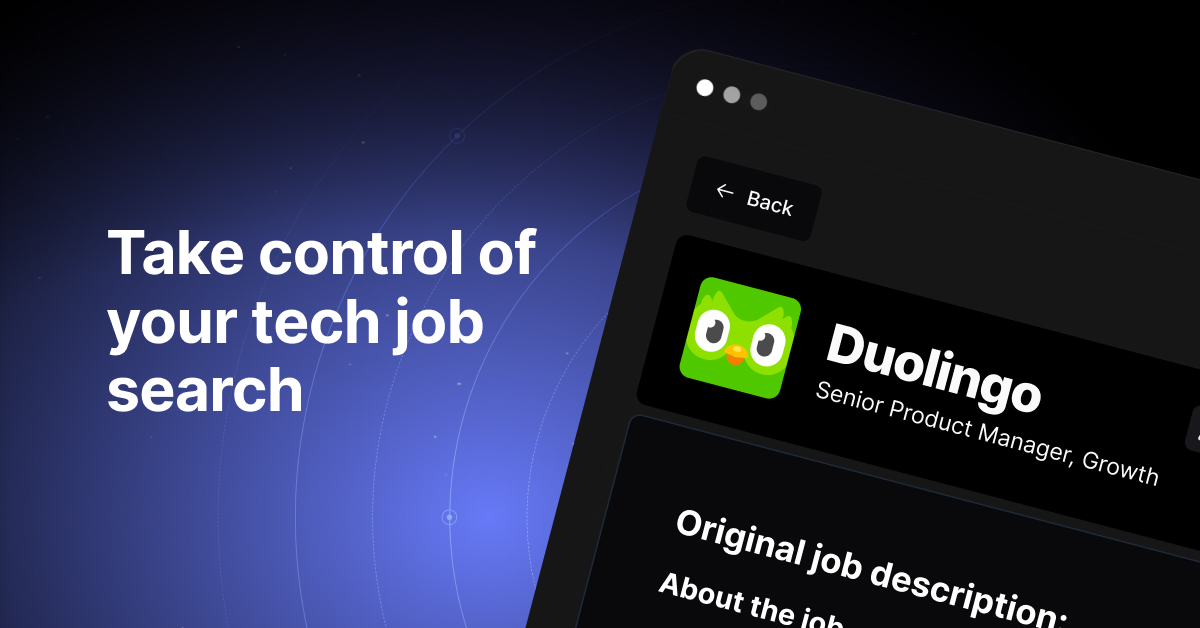