Difference between == and ===
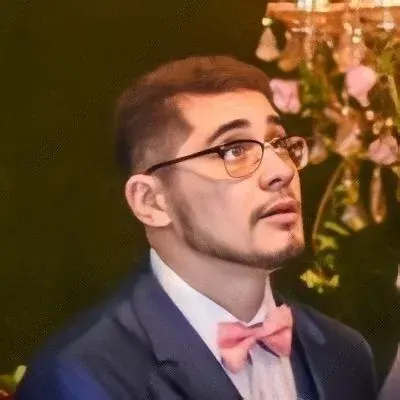
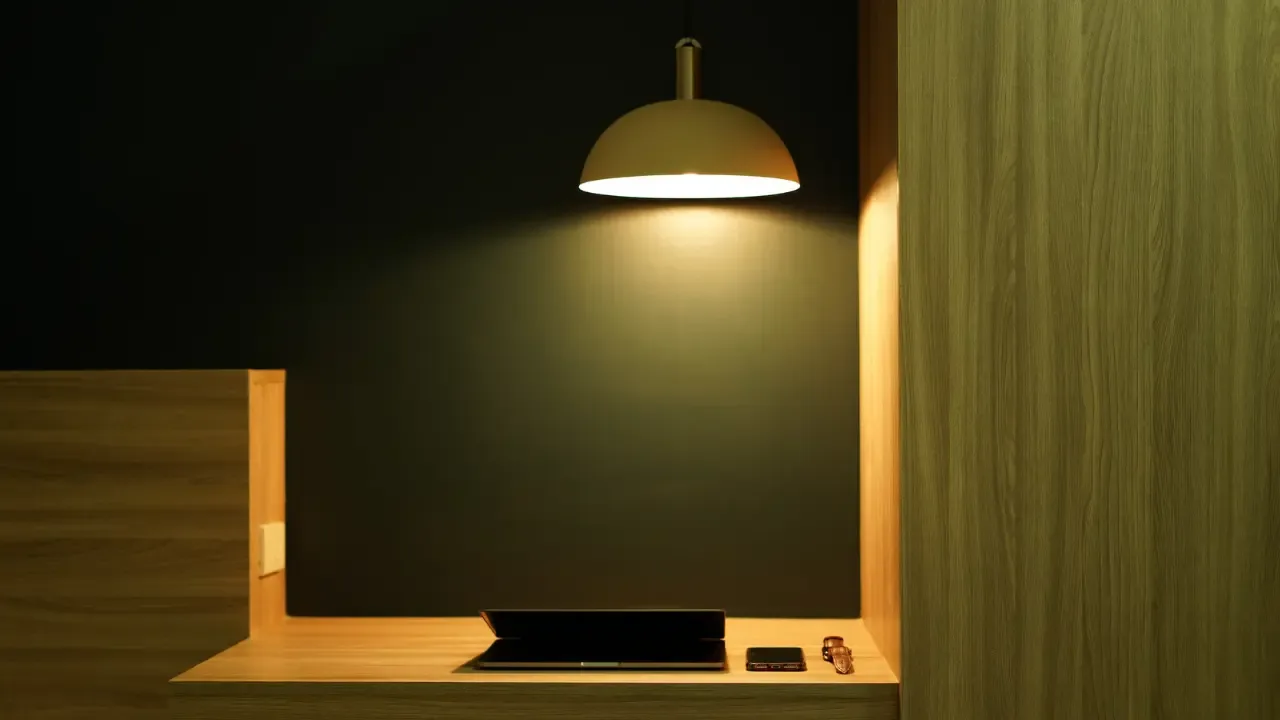
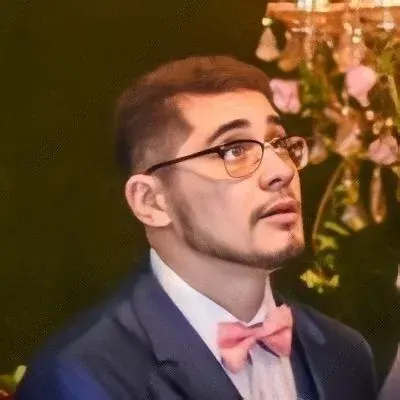
Equality Operators in Swift: == vs === ๐โโ๏ธ๐โโ๏ธ
Are you confused about the difference between the double equals (==
) and triple equals (===
) operators in Swift? ๐ค Don't worry, you're not alone! Many developers struggle to understand these seemingly similar operators. ๐ฉ But fear not! In this blog post, we'll break it down for you and provide easy solutions to common issues. Let's dive in! ๐โโ๏ธ
Understanding the Equality Operators ๐ค
The equality operators (==
and ===
) in Swift are used to compare the equality of two values. However, they have different behaviors and use cases. Let's take a closer look at each of them. ๐ง
1. Double Equals (==) Operator โก๏ธ
The double equals (==
) operator checks for value equality. It compares whether two values are equal in terms of their content. For example:
let x = 5
let y = 5
if x == y {
print("x is equal to y") // Output: x is equal to y
}
In this case, the condition x == y
is true
because both x
and y
have the same value, which is 5
. ๐
2. Triple Equals (===) Operator โก๏ธ
The triple equals (===
) operator checks for reference equality. It compares whether two objects or instances point to the exact same memory address. This operator is primarily used when working with reference types (e.g., classes) rather than value types (e.g., integers, strings). Here's an example:
class Person {
var name: String
init(name: String) {
self.name = name
}
}
let person1 = Person(name: "Alice")
let person2 = person1
if person1 === person2 {
print("person1 and person2 refer to the same instance") // Output: person1 and person2 refer to the same instance
}
In this code snippet, person1
and person2
are two different variables, but they both refer to the same instance of the Person
class. The condition person1 === person2
is true
because they share the same memory address. ๐ฒ
Common Issues and Easy Solutions ๐ ๏ธ
Now that we've covered the basics, let's address some common issues developers face when working with the equality operators, and how to solve them. ๐
1. Mistaking == for === or vice versa โโ
One common mistake is using the wrong equality operator. Remember, ==
is for value equality, while ===
is for reference equality. So, if you're dealing with value types like integers or strings, use ==
. If you're dealing with reference types like classes, use ===
. This simple rule will save you a lot of headaches! ๐ง ๐ก
2. Checking equality of optional values ๐โ๏ธ
When dealing with optional values, be cautious! Using ==
with optionals compares their wrapped values, which can sometimes lead to unexpected results. To avoid this, consider using optional binding (if let
) or the null coalescing operator (??
) to handle nil cases explicitly. Here's an example:
let optionalValue: Int? = nil
if optionalValue == 0 {
print("optionalValue is equal to 0") // This condition will wrongly evaluate to true
}
// Instead, use optional binding or the null coalescing operator
if let value = optionalValue {
print("optionalValue is not nil") // This condition will not execute since optionalValue is nil
}
let nonOptionalValue = optionalValue ?? 0
print("nonOptionalValue = \(nonOptionalValue)") // Output: nonOptionalValue = 0
By handling optional values properly, you can avoid unexpected behaviors and ensure your code is more robust. ๐
Your Turn! ๐๏ธ
Now that you've mastered the difference between ==
and ===
in Swift, it's time to put your knowledge into practice! ๐ Try out some code examples on your own and see how they behave. And don't forget to embrace the Swift playgrounds or Xcode's debugging tools for a hands-on learning experience. ๐งช๐จโ๐ป
If you still have questions or want to share your own experiences, we'd love to hear from you! Leave a comment below and let's discuss. Together, we can conquer Swift's equality operators! ๐ช๐ป
So, next time you come across the double equals (==
) or triple equals (===
) in Swift, you'll know exactly how they differ and which one to use. Happy coding! ๐๐