Determining if Swift dictionary contains key and obtaining any of its values
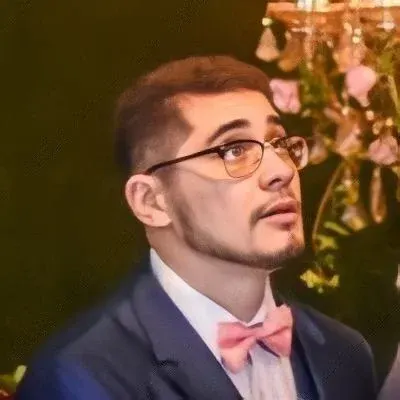
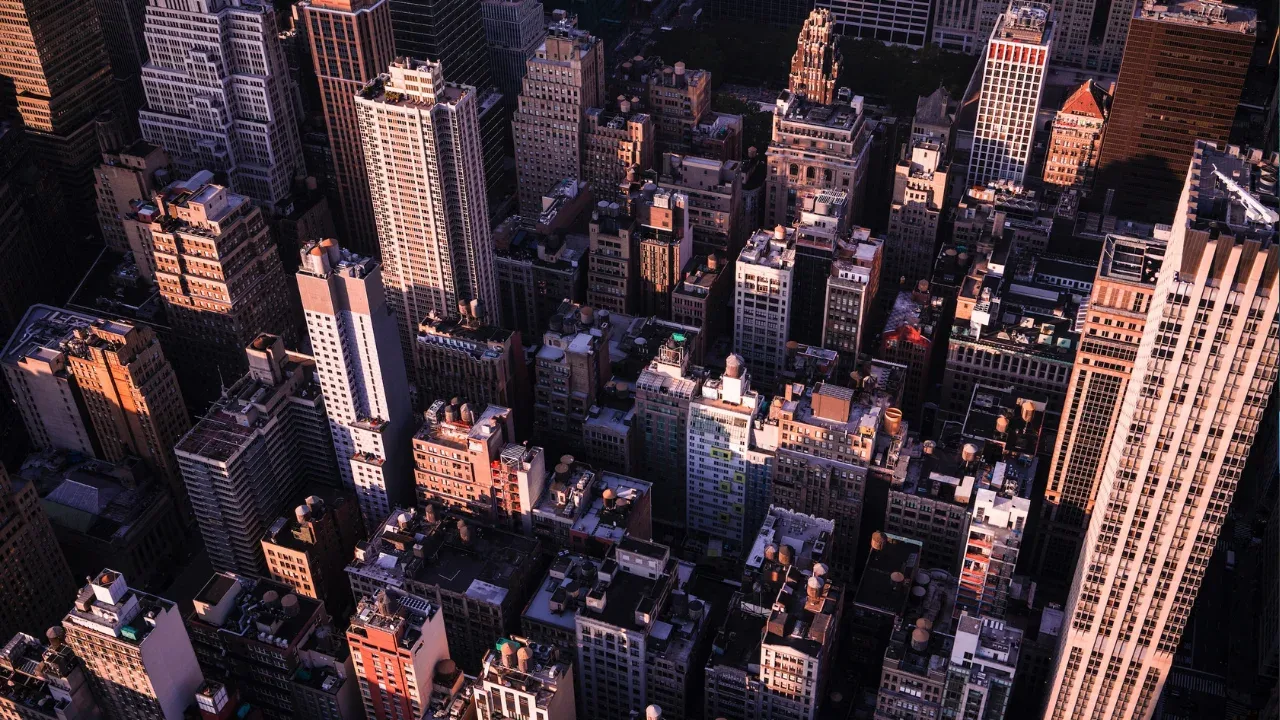
🚀 Determining if a Swift Dictionary Contains a Key and Obtaining Its Value 📚
Have you ever found yourself using clumsy code to determine if a Swift dictionary contains a specific key and then retrieving any of its values? 🤷♀️ Fear no more! In this blog post, we will show you how to elegantly solve this problem in Swift. Let's dive right in! 💻
The Clumsy Code 😣
Let's take a look at the code snippet provided:
// excerpt from method that determines if dict contains key
if let _ = dict[key] {
return true
} else {
return false
}
// excerpt from method that obtains the first value from the dict
for (_, value) in dict {
return value
}
While these snippets work, they might leave you scratching your head and thinking, "There must be a better way!" And indeed, there is a more elegant solution to this problem. 💡
The Elegant Solution 🌟
Determining if a Dictionary Contains a Key ✔️
Instead of using the if let _ = dict[key]
construct, we can use the Dictionary
class's contains
method to check if the key exists. This alternative provides a clearer and more readable approach. Here's an example:
if dict.contains(where: { $0.key == key }) {
return true
} else {
return false
}
By using contains(where:)
, we iterate through each key-value pair in the dictionary and return true
if the specified key matches any of the dictionary's keys. Otherwise, we return false
.
Obtaining a Value from the Dictionary 🔄
To retrieve any value from the dictionary instead of just the first one, we can enhance our solution using a guard statement. This ensures there is a value associated with the specified key before returning it. Check it out:
guard let value = dict[key] else {
// Handle the case when the key doesn't exist in the dictionary
// You can throw an error, return a default value, or do whatever suits your needs
// For example, you could return nil or an empty string ""
fatalError("Key doesn't exist in the dictionary")
}
return value
By using the guard statement, we safely unwrap the value associated with the key. If the key exists in the dictionary, we return the value. Otherwise, we handle the error case as needed.
Share Your Swift Wisdom! 📣
We hope these elegant solutions save you from using clumsy code and help you write more readable and efficient Swift applications. Do you have any other cool Swift tips and tricks that you'd like to share? Let us know in the comments below! 😄
Thanks for reading! 🙌
Happy coding! 💻💡
Disclaimer: The examples provided in this blog post assume a non-empty dictionary. Please adapt the solutions to handle cases where the dictionary may be empty.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
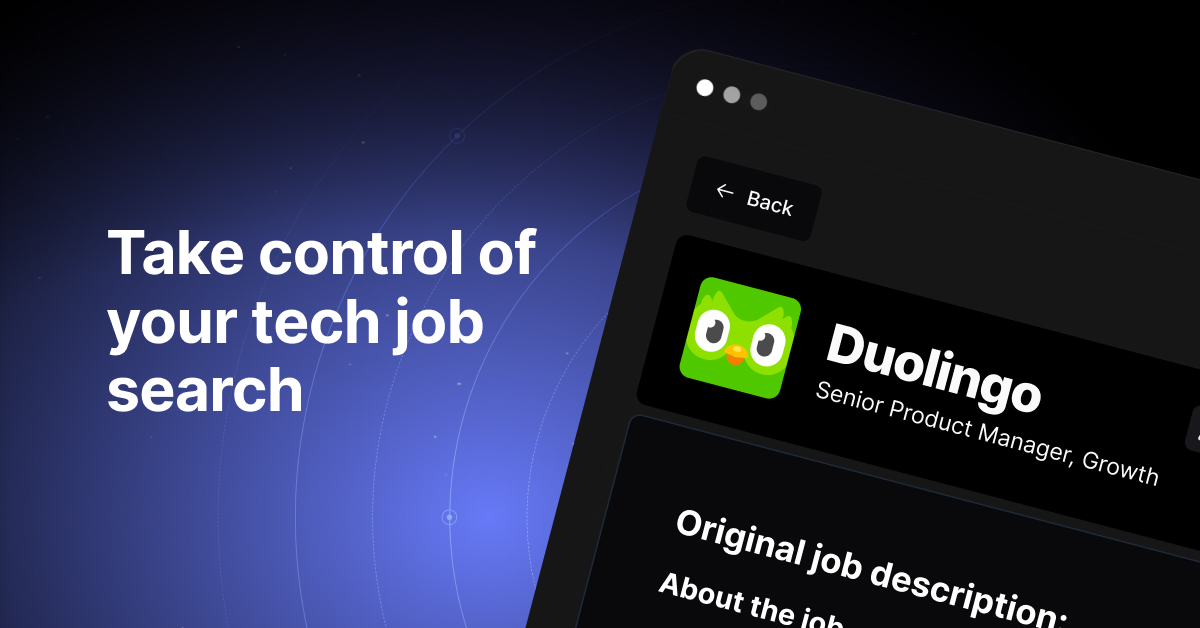