Checking if an object is a given type in Swift
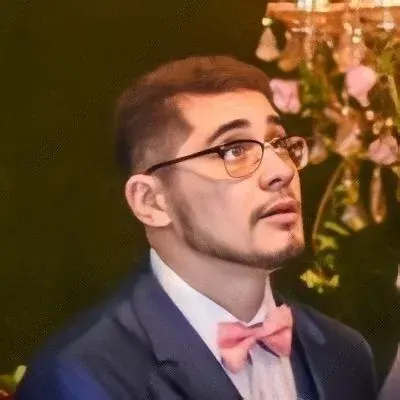
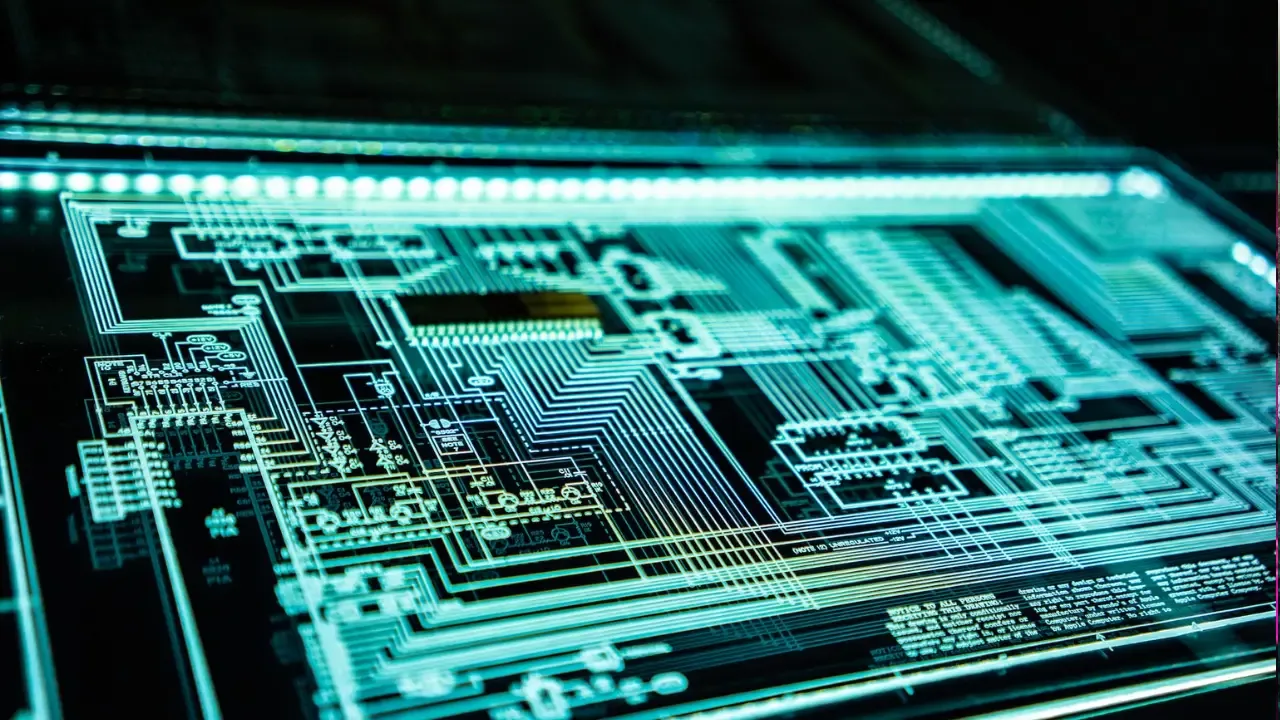
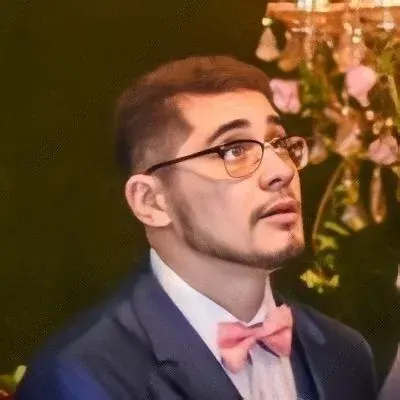
Checking if an object is a given type in Swift: A Handy Guide 📝
So, you have an array that contains AnyObject
and you'd like to identify all the elements that are of a particular type, such as an array instance. The good news is that Swift provides a straightforward way to accomplish this. In this blog post, we'll walk you through the process, address common issues, and offer easy solutions to your problem. Let's get started! 😎
Understanding the is
Operator
To check if an object is of a certain type in Swift, we can make use of the is
operator. This operator evaluates whether an instance is of a given type or a subclass of that type. It returns true
if the object matches the type and false
otherwise.
if object is Array<Any> {
// Object is an array
} else {
// Object is not an array
}
In the example above, we check if the object
is an instance of Array<Any>
. If it is, the code within the if
block will be executed; otherwise, the code within the else
block will run.
Iterating through an Array to Find Elements of a Specific Type
Now that we understand how to use the is
operator, let's use it to iterate through an array and find all elements that are array instances.
let array: [AnyObject] = [1, "hello", [2, 4], ["hi"], 5.7]
for element in array {
if let nestedArray = element as? [Any] {
// Element is an array
print(nestedArray)
}
}
Here, we define an array called array
that consists of various types. We iterate through each element of the array using a for
loop. To determine if an element is an array, we use optional type casting with as?
. If the element can be cast to [Any]
, it means it's an array, and we can safely work with it within the if
block.
In our example, the nested array [2, 4]
and ["hi"]
will be recognized as arrays and printed out.
Common Issues and Easy Solutions
Issue 1: Failing to Cast the Object
If you're having trouble casting the object to a specific type, make sure you're using the correct type in the cast expression. Pay close attention to any optional types or potential subclasses.
Issue 2: Array Containing AnyObject
vs. Any
If your array contains elements of type AnyObject
, you can check if an object is an array instance using is Array<AnyObject>
. However, if your array contains elements of type Any
, you should use is Array<Any>
.
Issue 3: Dealing with Optionals
If your array contains optional elements, you may need to unwrap them before checking their type. You can do this using optional binding or the nil coalescing operator.
Call-to-Action: Share Your Solutions and Tips! 🚀
Now that you've learned how to check if an object is of a given type in Swift, we'd love to hear from you! Have you faced any challenges in dealing with object types? Do you have any tips or tricks to share? Leave a comment below and let's spark a conversation! 💬
Happy coding! 💻✨