Any way to replace characters on Swift String?
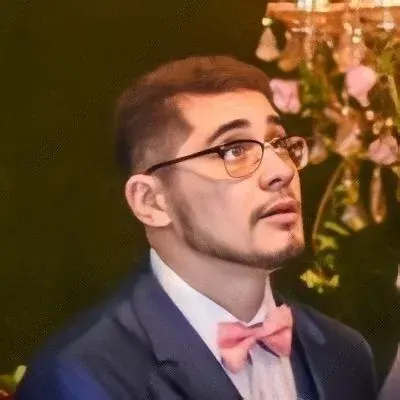
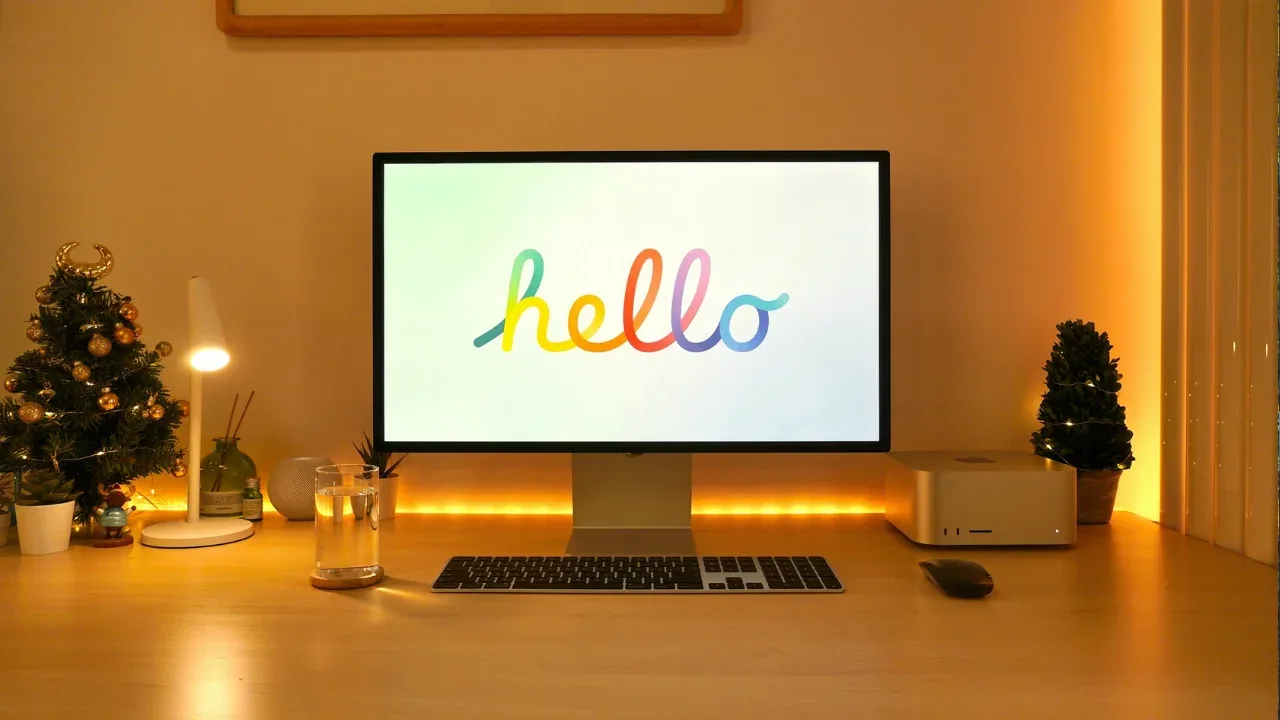
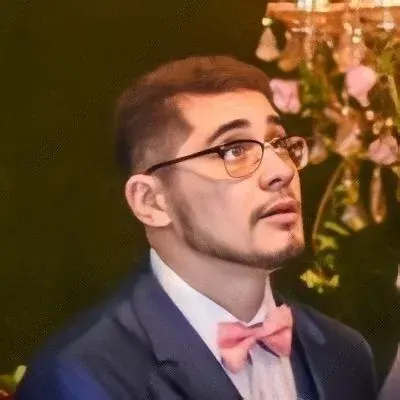
Any way to replace characters on Swift String? 🧐
Are you struggling to replace characters in a Swift String
? Don't worry, we got your back! In this guide, we'll address the common issues and provide easy solutions to help you achieve your desired result. Let's dive right in! 💪
The Problem: Replacing Characters in a Swift String
So, you have a string like this: "This is my string" and you want to replace all the spaces with the "+" symbol. Your expected output should be "This+is+my+string". But how can you achieve this transformation without breaking a sweat? 🤔
The Solution: Swift's replacingOccurrences(of:with:)
Method
Swift provides a handy method called replacingOccurrences(of:with:)
, which allows you to replace occurrences of a specific substring with another substring.
Let's see how we can use this method to replace spaces with the "+" symbol in your string:
let originalString = "This is my string"
let modifiedString = originalString.replacingOccurrences(of: " ", with: "+")
print(modifiedString) // Output: "This+is+my+string"
Voila! With just a single line of code, you were able to replace all the spaces with the "+" symbol. 🎉
Additional Tips and Tricks
Case Insensitivity: By default, the
replacingOccurrences(of:with:)
method is case-sensitive. If you want the replacement to be case-insensitive, you can use theoptions
parameter and set it to.caseInsensitive
. Here's an example:
let originalString = "This is my string"
let modifiedString = originalString.replacingOccurrences(of: " ", with: "+", options: .caseInsensitive)
print(modifiedString) // Output: "This+is+my+string"
Replace Multiple Substrings: If you need to replace multiple different substrings within a single string, you can simply chain multiple
replacingOccurrences(of:with:)
method calls. Here's an example:
let originalString = "This is my string"
let modifiedString = originalString
.replacingOccurrences(of: " ", with: "+")
.replacingOccurrences(of: "my", with: "your")
print(modifiedString) // Output: "This+is+your+string"
Conclusion
Replacing characters in a Swift String
might seem like a daunting task, but it's actually quite simple with the help of the replacingOccurrences(of:with:)
method. We hope this guide has provided you with easy solutions to your problem. Now, go ahead and rock your Swift coding skills! 💻✨
If you found this guide helpful, don't forget to share it with fellow developers who might be facing the same issue. And if you have any additional questions or insights, feel free to leave a comment below. Happy coding! 🚀🔥